Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial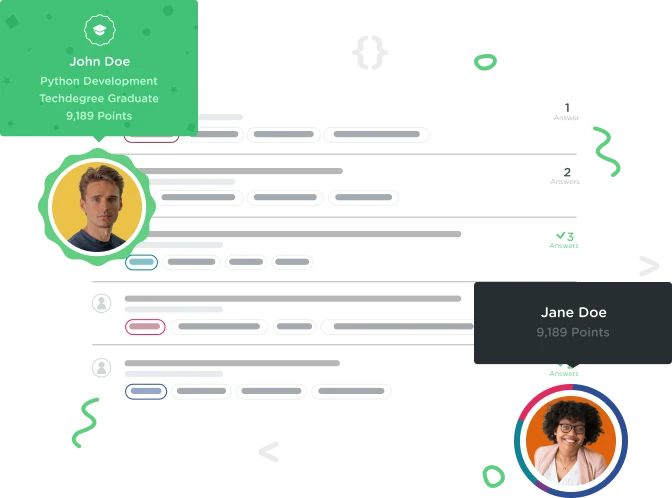

Michael Nanni
7,358 PointsAm I on the right track here? Unable to return 0 value.
In workspaces I can get the correct value with this code if all dice are the same value. Like all fives, fours etc.
What's eluding me is how to return a 0 value if not all dice are the same. Is my code even close?
class YatzyScoresheet:
def score_ones(self, hand):
return sum(hand.ones)
def _score_set(self, hand, set_size):
scores = [0]
for worth, count in hand._sets.items():
if count == set_size:
scores.append(worth*set_size)
return max(scores)
def score_one_pair(self, hand):
return self._score_set(hand, 2)
def score_chance(self, hand):
return sum(hand)
def score_yatzy(self, hand):
yatzy = [0]
for item in hand:
if item == hand[0]:
yatzy.append(item)
elif item != hand[0]:
return hand == 0
return sum(hand)*2
2 Answers

KRIS NIKOLAISEN
54,967 Points1) If there is a yatzy you need to loop through all items. Your loop will always exit the first time through because
elif item == hand[0]:
will always be true (comparing item to itself) for the first item
2) You should return just the value 0 or 50. You are currently returning the result of the comparison of hand to 0 or hand to 50.
3) Variable yatzy isn't used and can be deleted
The following will loop through all items unless there isn't a match. If that condition is met (all it has to happen is once) then you are safe to exit with a return value of 0. If you are able to loop through all items to completion, then they are all the same. Therefore after the loop you can return 50.
def score_yatzy(self, hand):
for item in hand:
if item != hand[0]:
return 0
return 50

KRIS NIKOLAISEN
54,967 PointsThere is no need to sum the hand. If all dice are the same you are to return 50. Therefore your logic could be simplified to just checking if item != hand[0]:
return 0 in your loop. Otherwise return 50.

Michael Nanni
7,358 PointsHey Kris. If I'm understanding you and the challenge correctly, then what you mean is that if all dice are the same I can just return the value 50. If not all dice are the same, then I can return 0. I think I'm missing some logic here because what I have isn't working in workspace. Here's what I'm trying to run in the console.
>>> from hands import YatzyHand
>>> from dice import D6
>>> from scoresheets import YatzyScoresheet
>>> five = D6(value=5)
>>> four = D6(value=4)
>>> hand = YatzyHand()
>>> hand[:] = [five, five, five, five, five]
>>> YatzyScoresheet.score_yatzy(hand)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: score_yatzy() missing 1 required positional argument: 'hand'
Here's my code:
def score_yatzy(self, hand):
yatzy = [0]
for item in hand:
if item != hand[0]:
return hand == 0
elif item == hand[0]:
return hand == 50