Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial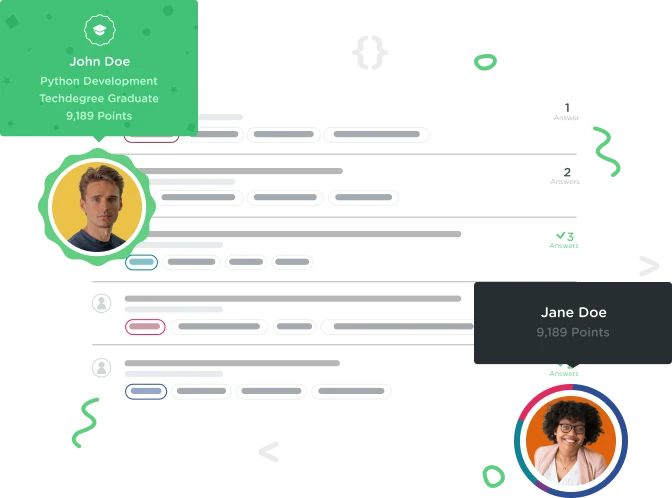

lvwdhydynw
6,443 PointsAm I right about this? (Structures, arrays, instances)
This is my code right now: http://pastebin.com/rN7ekgDd
It's just for fun and in order to learn using structures. Anyway, I have just run into something I don't really understand.
var premierLeague = League(name: "Premier League", country: "England")
var chelsea = Club(name: "Chelsea FC", country: "England")
var manUnited = Club(name: "Manchester United FC", country: "England")
premierLeague.teams.append(chelsea)
premierLeague.teams.append(manUnited)
premierLeague.match(homeTeam: &chelsea, awayTeam: &manUnited, goalsForHomeTeam: 4, goalsForAwayTeam: 0)
chelsea.statusInTable()
manUnited.statusInTable()
premierLeague.createLTable()
Alright. So I created an instance of structure League and then two instances of structure Club. Then I added them both premierLeague's array called "teams". So far so good I then let Chelsea and Manchester United play a match. The method "match" updated their statistics (number of played matches, victories/defeats and goals for them/against them).
On both chelsea and manUnited I then used method statusInTable() to show their statistics and it worked. Then I created a method createTable() in structure League and I decided to try it out. And suddenly, both teams had default values (0 everywhere).
I wondered what's wrong. Then I tried this:
premierLeague.match(homeTeam: &premierLeague.teams[0], awayTeam: &premierLeague.teams[3], goalsForHomeTeam: 4, goalsForAwayTeam: 0)
I called the method again and it worked!
I was honestly surprised. I think it has something to do with the value/reference types
My guess is that when I added "chelsea" and "manUnited" to that arrays, I added their copies and them themselves. So, while "chelsea" and "manUnited" were playing (and their statistics were changing), it had nothing to do with their copies in array "teams" (premierLeague.teams[0] and premierLeague.teams[3]).
And so, when I wanted to make a table from teams saved in array "teams". they had default values because I haven't been working with them.
Extra question: So, next time instead of this
var chelsea = Club(name: "Chelsea FC", country: "England")
premierLeague.teams.append(chelsea)
I should do this
premierLeague.teams.append(Club(name: "Chelsea FC", country: "England"))
unless I will need to use it somewhere else as well - right?