Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial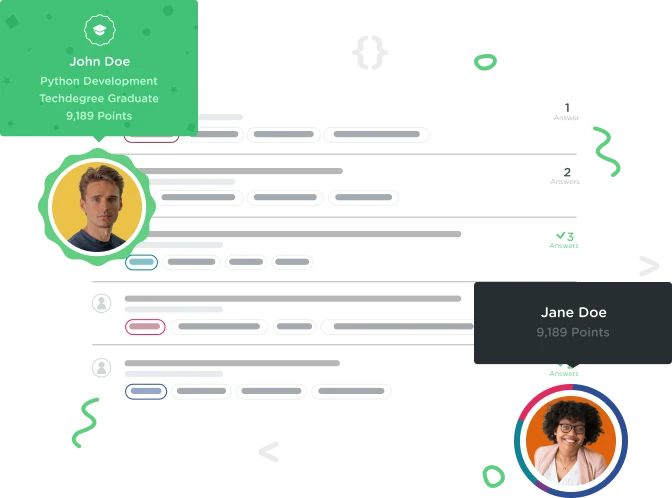

Dan Holroyd
1,172 PointsAm I storing the variable incorrectly?
I'm trying to A) store the random number as a variable, and B) use .index to return that position's value. The syntax doesn't look highlighted the way it should.
import random
def random_item('value'):
here = random.randint(0,4)
return random_item('value').index(here)
# EXAMPLE
# random_item("Treehouse")
# The randomly selected number is 4.
# The return value would be "h"
1 Answer
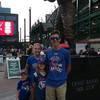
AJ Salmon
5,675 PointsHi Dan,
There are a couple of issues here. Firstly, when you set the parameter of the function, you don't need quotation marks, as the argument is really just a placeholder in this case. When the code challenge tests your code, it will call the function and pass it any iterable it wants, like in the example "Treehouse." Your random number wouldn't work, because it needs to have the same max range as the length of whatever the iterable is, minus one (because indexes start at zero.) SO, whenever you use the key word value inside the function, you're really telling it, "Hey, whatever I do to this keyword, do to the actual argument that gets passed to you later on" if that makes sense, haha. I'll help you out a little bit:
import random
def random_item(value):
here = random.randint(0, len(value)-1)
return ?
With the len() method, we get whatever the length the the iterable is as an integer. And with randint, you give it a range, of two intergers, 0, and then the length of any iterable that happens to be passed to the function. This makes it so that the function can be used with any and every iterable out there, not just the parameter you set it with. Now return iterable member that has the same index as the randomly generated number. If you have any questions at all about functions, or anything else in this challenge, please feel free to ask, hopefully this helps!
Cam Treschuk
7,378 PointsCam Treschuk
7,378 PointsA: You are storing the variable correctly, but treehouse wants the second number to be the length of value, minus one. B: For the return, you would do value[here]. Also, the parameter 'value' shouldn't be in quotes.
Here's the code: