Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial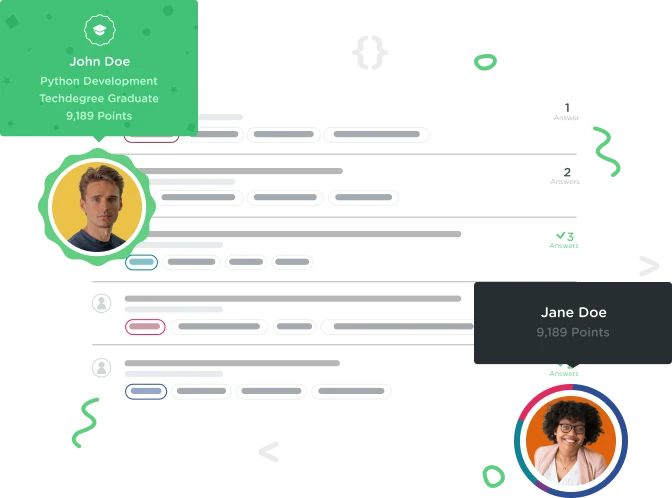
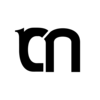
Colin Marshall
32,861 PointsAm I understanding this correctly?
In this video we create the remove_item method.
def mark_complete(name)
if index = find_index(name)
todo_items[index].mark_complete!
return true
else
return false
end
end
The line I'm curious about is:
if index = find_index(name)
I understand that the find_index method is run to see if the name passed through the mark_complete method is present in the array. It will set the index variable to nil if it's not present or the index of the array item where the name appears if it is present.
Is the reason that it will pass as true if name is present is because index will be set to an integer and the boolean of an integer object evaluates to true in Ruby?
4 Answers

Daniel Cunningham
21,109 PointsMy understanding of the logic is as follows:
The find_index method: <br> 1. assigns index = 0 <br> 2. checks a "todo_item" for a match <br> 3. if it matches, found = true and the do method stops. Otherwise, index +=1 and check next item. <br> 4. When the method stops, if the item is found (if found = true), then the return will be the index, or else it will return nil. <br> <br>
Hypothetically, if there were 5 items in the todo_list, the name = "squash", and "squash" was NOT in the list , the procedure would loop through index until 5, assigning index = 5 at the end. However, the variable found is still false (found = false), forcing it to return nil in the next if function. <br> <br> in the case of the mark_complete method, if we were to assign the two sides of the equation: index = 5 <br> find_index(name) = nil <br>
the if statement would populate: <br>
if 5 == nil <br> todo_items[index].mark_complete! <br> return true <br> else <br> return false <br> end <br>
This would of course, be false and therefore return false. The way the index effectively "tests itself" is very strange, but it seems powerful. <br>
Hope that helps!

Derek Fan
4,645 PointsHi Danial,
Thank you very much for help. However, based on your opinion, shall the code be "if index == find_index(name)" instead of "if index = find_index(name)" ?
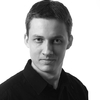
Maciej Czuchnowski
36,441 PointsIt boils down to "falsy" and "truthy" values. Whenever you are checking for boolean values (for example in if statements), nil
and false
will always be treated as false
- they are falsy values. All other values are treated as true when evaluated. You have to be careful here, because in JavaScript (which you usually use side by side with Ruby in web development) it's different - for example 0 is treated as false (in Ruby EVERY number is a truthy value) and empty string is treated as false. This table can be helpful: https://www.launchacademy.com/codecabulary/learn-ruby/truthy-and-falsy

Rachelle Wood
15,362 PointsThanks for bringing this up. This is a trap I would have probably fallen into. Very useful!

Derek Fan
4,645 Pointsthanks, Maciej!!!

Jose Vidal
15,016 PointsMy understanding is that he uses the 'true' and 'false' values only because, later on, he does this:
if todo_list.mark_complete("Milk")
puts "Milk was marked as complete."
end
Since the 'mark_complete' function returns 'true' (if all goes well), his 'if' statement translates to 'if (true) puts "Milk...".
It is a funny way to do it. Notice this is the way he is running the 'mark_complete' method: through the if statement. Since the method is called with a ("Milk") parameter being passed to it, it runs right then and there and returns 'true'.
In my humble/unexperienced opinion: the boolean return he uses is not necessary by itself. It is only useful because he decided to use an 'If' statement to both mark 'Milk' as complete and use the puts.
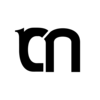
Colin Marshall
32,861 PointsThanks for the answers guys!!!
Brandon Keene
7,217 PointsBrandon Keene
7,217 PointsI think that's right. If I understand it correctly, all Ruby objects evaluate to true or false, and in this case if index evaluates to nil, it evaluates to false because nil and false are both boolean values that evaluate to false.