Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial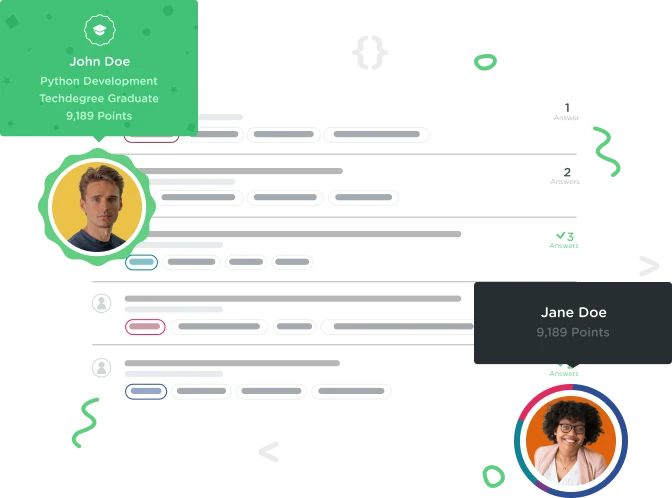
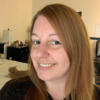
Charlynn Schmiedt
9,288 PointsAm I using the 'or' operator wrong, or is it something else?
Based on the directions, I created an if statement that tested whether the input was 0, 1, or 2 and anything else would default to the else statement. I keep getting an error that my if statement isn't correct. Am I missing something?
const laws = document.getElementsByTagName('li');
const indexText = document.getElementById('boldIndex');
const button = document.getElementById('embolden');
button.addEventListener('click', (e) => {
const index = parseInt(indexText.value, 10);
for (let i = 0; i < laws.length; i += 1) {
let law = laws[i];
// replace 'false' with a correct test condition on the line below
if (index === 0 || 1 || 2) {
law.style.fontWeight = 'bold';
} else {
law.style.fontWeight = 'normal';
}
}
});
<!DOCTYPE html>
<html>
<head>
<title>Newton's Laws</title>
</head>
<body>
<h1>Newton's Laws of Motion</h1>
<ul>
<li>An object in motion tends to stay in motion, unless acted on by an outside force.</li>
<li>Acceleration is dependent on the forces acting upon an object and the mass of the object.</li>
<li>For every action, there is an equal and opposite reaction.</li>
</ul>
<input type="text" id="boldIndex">
<button id="embolden">Embolden</button>
<script src="app.js"></script>
</body>
</html>
1 Answer
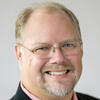
Jason Larson
8,353 PointsYou are using the or statement correctly, sort of. Unfortunately, you can't write things as succinctly as you have it. You have to put the entire statement in there, like so:
if (index === 0 || index === 1 || index === 2) {...}
While that will fix your conditional statement, it won't pass the challenge. Note that the if statement is within the for loop, which will loop over the possible values that index should match on, so you don't need the or statements at all. Just check to see if the variable that is being looped over (i
) equals the value in the textbox (index
).
Charlynn Schmiedt
9,288 PointsCharlynn Schmiedt
9,288 PointsWow, I really overthought this one. Thank you for your help, and thanks also for reminding me that variables need to go with every and/or condition in an if statement. I forgot that!