Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial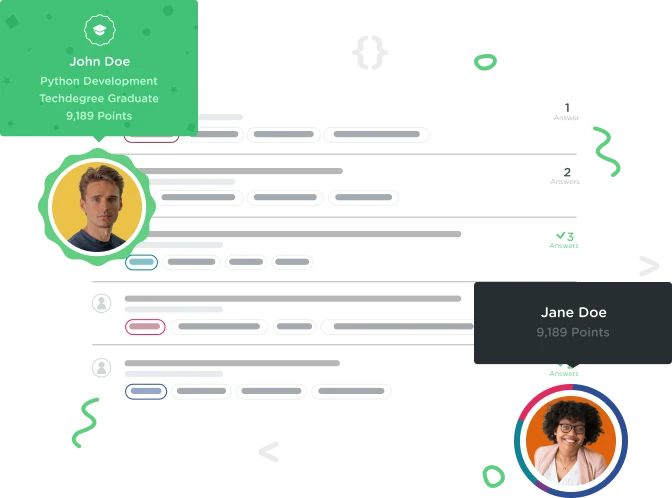

Robert Amato
Courses Plus Student 3,271 PointsAn easier way of writing function. It's not a treehouse assignment, just practice.
//Mr. Cerise teaches high school math. Write a function
//that assigns and prints a letter grade, given an integer
//representing a score from 0 to 100? Those getting 90+
//get an ‘A’, 80-89 earn ‘B’, 70-79 is a ‘C’, 60-69 should
//get a ‘D’, and lower than 60 receive ‘F’.
//Example: given 88, you should log "Score:88. Grade: B".
//Given the score 61, log the string "Score: 61. Grade: D".
//For an additional challenge, add ‘-’ signs to scores in
//the bottom two percent of A, B, C and D scores, and “ +”
//signs to the top two percent of B, C and D scores
//(sorry, Mr. Cerise never gives an A+).
//Example: Given 88, console.log "Score: 88. Grade: B+".
//Given 61, log "Score: 61. Grade: D-".
const letterGrade = (grade) => {
if (grade < 60) {
console.log(`Score: ${grade}, Grade: F`);
} else if (grade >= 60 && grade <= 62) {
console.log(`Score: ${grade}, Grade: D-`);
} else if (grade >= 68 && grade < 70) {
console.log(`Score: ${grade}, Grade: D+`);
} else if (grade > 62 && grade < 68) {
console.log(`Score: ${grade}, Grade: D`);
} else if (grade >= 70 && grade <= 72) {
console.log(`Score: ${grade}, Grade: C-`);
} else if (grade >= 78 && grade < 80) {
console.log(`Score: ${grade}, Grade: C+`);
} else if (grade > 72 && grade < 78) {
console.log(`Score: ${grade}, Grade: C`);
} else if (grade >= 80 && grade <= 82) {
console.log(`Score: ${grade}, Grade: B-`);
} else if (grade >= 88 && grade < 90) {
console.log(`Score: ${grade}, Grade: B+`);
} else if (grade > 82 && grade < 88) {
console.log(`Score: ${grade}, Grade: B`);
} else if (grade >= 90 && grade <= 92) {
console.log(`Score: ${grade}, Grade: A-`);
} else if (grade > 92) {
console.log(`Score: ${grade}, Grade: A`);
}
};
letterGrade(58);
letterGrade(69);
letterGrade(65);
letterGrade(61);
letterGrade(79);
letterGrade(75);
letterGrade(71);
letterGrade(89);
letterGrade(85);
letterGrade(81);
letterGrade(99);
letterGrade(92);
1 Answer
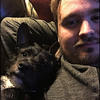
Matthew Long
28,407 PointsThe if/else statements are pretty ugly and hard to read so maybe you could consider using a switch statement? That's the first thing that comes to mind.. I think a switch statement isn't really meant to be used in this way so be aware of that. Maybe you could just extract the string that is being logged out and create a new function out of that? That would at least clean up the conditional statements a little, and making it easier to read. Either way here is what I have using the switch statement:
const gradeString = grade => {
console.log(`Score: ${grade}, Grade: ${letterGrade(grade)}`);
}
const letterGrade = grade => {
switch (true) {
case (grade < 60): return "F";
case (grade >= 60 && grade <= 62): return "D-";
case (grade >= 68 && grade <= 70): return "D+";
case (grade >= 62 && grade < 68): return "D";
case (grade >= 70 && grade <= 72): return "C-";
case (grade >= 78 && grade < 80): return "C+";
case (grade >= 72 && grade < 78): return "C";
case (grade >= 80 && grade <= 82): return "B-";
case (grade >= 88 && grade < 90): return "B+";
case (grade >= 82 && grade < 88): return "B";
case (grade >= 90 && grade <= 92): return "A-";
case (grade >= 98): return "A+";
case (grade >= 92 && grade < 98): return "A";
}
}
gradeString(68);
// Score: 68, Grade: D+"
Consider doing this except keeping the if/else statements instead of the switch?
Robert Amato
Courses Plus Student 3,271 PointsRobert Amato
Courses Plus Student 3,271 PointsGreat thanks! I will go change some code around! I will go read up on the switch statement a little more. I saw that in my searches but didn't really understand its use.