Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial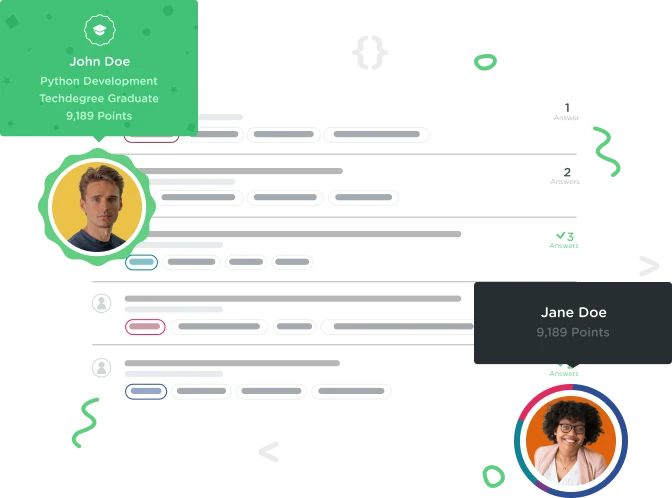

Zailey Walls
5,427 PointsAn object using another objects methods?
In the playlist project we created play and stop methods on the song object. But then we use those two functions from within the Playlist's play and stop methods... why are we able to do this?
//song
Song.prototype.play = function() {
this.is_playing = true;
}
Song.prototype.stop = function() {
this.is_playing = false;
}
//playlist
Playlist.prototype.play = function() {
this.songs[this.current_song].play();
}
Playlist.prototype.stop = function() {
this.songs[this.current_song].stop();
}
Also, why do we need to create play and stop functions for both objects?
3 Answers
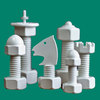
Steven Parker
231,275 PointsIt's not important that the calls are within the playlist's methods. What matters it that the play and stop methods are being called on song objects (which are held in the songs property of the playlist). They are still song object methods.
Inside the playlist, this refers to the playlist. But this.songs[some_index] is a song object.
As to why you would have similar methods for both objects, it's mostly a convenience. This way, when working with a playlist, you can simply call my_playlist.play()
where otherwise you'd need to call my_playlist.songs[my_playlist.current_song].play();
(plus you don't have to specify current_song).

nagamani palamuthi
6,160 Pointsto add to Steven's answer, I think because all the properties and methods declared in both objects - Songs and Playlist are public variables which we have not spoken about so far. May be he will talk about it further down this lesson. public variables inside a constructor can be accessed outside the object unlike private variables which are declared inside a constructor using the keyword var.
Hope I am right.... Pls correct me if I am wrong!

nagamani palamuthi
6,160 Pointsyou are creating the play and stop methods in the song object only. You are calling those methods in the playlist object using currentSong.play() and currentSong.stop();