Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial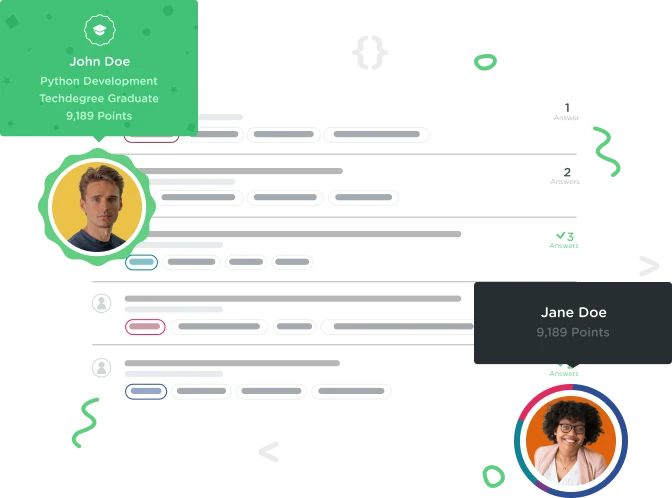
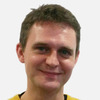
Joel Brennan
18,300 PointsAn unhandled exception of type 'System.InvalidOperationException' occurred in EntityFramework.SqlServer.dll
ComicBook.cs:
namespace ComicBookGalleryModel.Models
{
public class ComicBook
{
public ComicBook()
{
Artists = new List<ComicBookArtist>();
}
public int Id { get; set; }
public int IssueNumber { get; set; }
public string Description { get; set; }
public DateTime PublishedOn { get; set; }
public decimal? AverageRating { get; set; }
public Series Series { get; set; }
public ICollection<ComicBookArtist> Artists { get; set; }
public String displayText
{
get
{
return $"{Series?.Title} #{IssueNumber}";
}
}
public void AddArtist(Artist artist, Role role)
{
Artists.Add(new ComicBookArtist()
{
Artist = artist,
Role = role
});
}
}
}
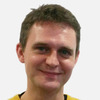
Joel Brennan
18,300 PointsArtist.cs:
namespace ComicBookGalleryModel.Models
{
public class Artist
{
public Artist()
{
ComicBooks = new List<ComicBookArtist>();
}
public int Id { get; set; }
public string Name { get; set; }
public ICollection<ComicBookArtist> ComicBooks { get; set; }
}
}
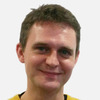
Joel Brennan
18,300 PointsProgram.cs:
namespace ComicBookGalleryModel
{
class Program
{
static void Main(string[] args)
{
using (var context = new Context())
{
var series1 = new Series()
{
Title = "The Amazing Spiderman"
};
var series2 = new Series()
{
Title = "The Invicible Iron Man"
};
var artist1 = new Artist()
{
Name = "Stan Lee"
};
var artist2 = new Artist()
{
Name = "Steve Ditko"
};
var artist3 = new Artist()
{
Name = "Jack Kirby"
};
var role1 = new Role()
{
Name = "Script"
};
var role2 = new Role()
{
Name = "Pencils"
};
var comicBook1 = new ComicBook()
{
Series = series1,
IssueNumber = 1,
PublishedOn = DateTime.Today
};
comicBook1.AddArtist(artist3, role1);
comicBook1.AddArtist(artist2, role2);
var comicBook2 = new ComicBook()
{
Series = series1,
IssueNumber = 2,
PublishedOn = DateTime.Today
};
comicBook2.AddArtist(artist1, role1);
comicBook2.AddArtist(artist3, role2);
var comicBook3 = new ComicBook()
{
Series = series2,
IssueNumber = 1,
PublishedOn = DateTime.Today
};
comicBook3.AddArtist(artist1, role2);
comicBook3.AddArtist(artist2, role2);
context.ComicBooks.Add(comicBook1);
context.ComicBooks.Add(comicBook2);
context.ComicBooks.Add(comicBook3);
context.SaveChanges();
var comicBooks = context.ComicBooks
.Include(cb => cb.Series)
.Include(cb => cb.Artists.Select(a => a.Artist))
.Include(cb => cb.Artists.Select(a => a.Role))
.ToList();
foreach (var comicBook in comicBooks)
{
var artistRolesNames = comicBook.Artists
.Select(a => $"{a.Artist.Name} - {a.Role.Name}").ToList();
var artistRolesDisplayText = string.Join(", ", artistRolesNames);
Console.WriteLine(comicBook.displayText);
Console.WriteLine(artistRolesDisplayText);
}
Console.ReadLine();
}
}
}
}
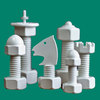
Steven Parker
231,275 PointsIf you run in Debug mode, you should be able to find out exactly where in the code this occurs.
2 Answers
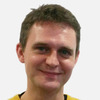
Joel Brennan
18,300 PointsHi Steven,
Additional information: A specified Include path is not valid. The EntityType 'ComicBookGalleryModel.ComicBookArtist' does not declare a navigation property with the name 'Role'.
Which is hit when it gets to this block: var comicBooks = context.ComicBooks .Include(cb => cb.Series) .Include(cb => cb.Artists.Select(a => a.Artist)) .Include(cb => cb.Artists.Select(a => a.Role)) .ToList();
I can't figure it out.
I thought I have the navigation property defined. Also the Roles.dbo doesn't seem to get generated.
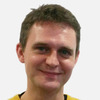
Joel Brennan
18,300 PointsFound it! I had forgotten to add the public access modifier if Role.cs. Forgot to post my Role.cs Sorry. Thanks for the help.
Joel Brennan
18,300 PointsJoel Brennan
18,300 PointsComicBookArtist.cs: