Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial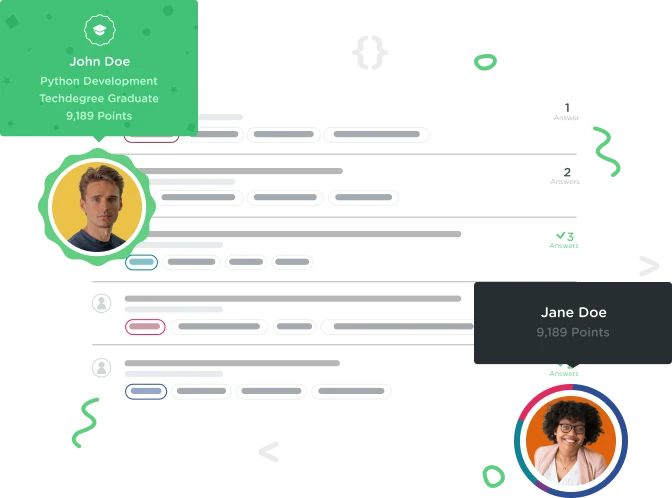

Constantine Antonakos
9,741 PointsAndroid Animations
Can anyone point me to good Android tutorials for animations, such as tween animations as show in Google's DevBytes series with Chet Haas?
Or could we perhaps get an awesome Treehouse-style Android animation tutorial (any, but perhaps ListView?) from our very awesome Ben Jakuben? :)
I'm trying to create ListView animations for my Android app.
2 Answers

Ben Jakuben
Treehouse TeacherThis is a good idea, I'll keep this in mind for my next workshop or tip video. In the meantime, here is some sample animation code that might help you get started. You can find more information about each type of animation in the Android documentation.
MainActivity.java
public class MainActivity extends Activity {
protected ImageView mRotatingImage;
protected ImageView mSlidingImage;
protected ImageView mScaleImage;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Rotate an image
mRotatingImage = (ImageView) findViewById(R.id.rotatingImage);
Animation rotation = AnimationUtils.loadAnimation(this, R.anim.rotate);
rotation.setRepeatCount(Animation.INFINITE);
mRotatingImage.startAnimation(rotation);
// Slide an image back and forth horizontally
mSlidingImage = (ImageView) findViewById(R.id.slidingImage);
Animation translation = new TranslateAnimation(0, 320,0, 0);
translation.setDuration(1000);
translation.setRepeatCount(Animation.INFINITE);
translation.setRepeatMode(Animation.REVERSE);
mSlidingImage.startAnimation(translation);
// Zoom in and out of an image
mScaleImage = (ImageView) findViewById(R.id.scaleImage);
Animation resize = new ScaleAnimation(1, 2, 1, 2, 200, 50);
resize.setDuration(2000);
resize.setRepeatCount(Animation.INFINITE);
resize.setRepeatMode(Animation.REVERSE);
mScaleImage.startAnimation(resize);
}
}
rotate.xml (in res/anim)
<?xml version="1.0" encoding="UTF-8"?>
<rotate
xmlns:android="http://schemas.android.com/apk/res/android"
android:interpolator="@android:anim/linear_interpolator"
android:fromDegrees="0"
android:toDegrees="360"
android:pivotX="50%"
android:pivotY="50%"
android:repeatCount="2"
android:duration="1500" />

Constantine Antonakos
9,741 PointsThank you so much!! I'm incredibly grateful for your thoughtful response! :)
Constantine Antonakos
9,741 PointsConstantine Antonakos
9,741 PointsThank you! I'm excited to see your future Android videos. :)