Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial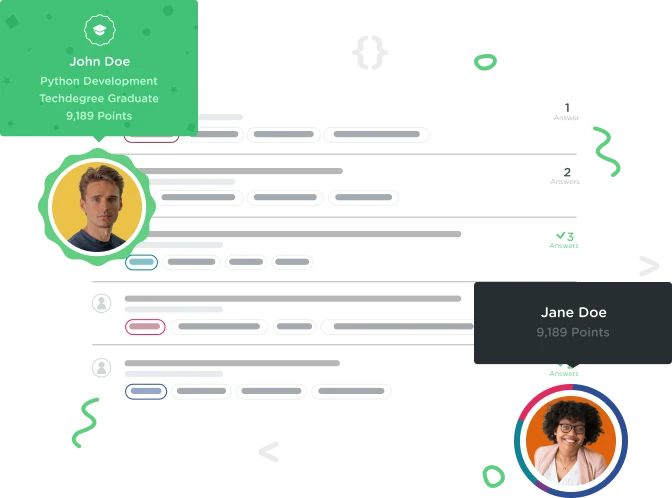

Sarvesh Tandon
1,142 PointsAndroid Application doing too much work on its main thread error. Pls help.
Why is it show- I/Choreographer: Skipped 120 frames! The application may be doing too much work on its main thread. - even after I have moved the time consuming task onto a new thread. Please Help.
package com.example.sarvesh007.othello;
import android.content.Context;
import android.graphics.drawable.Drawable;
import android.os.Build;
import android.os.SystemClock;
import android.support.annotation.RequiresApi;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.Toast;
import java.sql.Time;
import java.util.Timer;
@RequiresApi(api = Build.VERSION_CODES.LOLLIPOP)
public class GameActivity extends AppCompatActivity implements View.OnClickListener{
Button squares[][]= new Button[8][8];
int matrix[][] = new int[8][8];
int col = 0, row = 0;
String TAG = GameActivity.class.getSimpleName();
Drawable userButtonIcon, computerButtonIcon, emptyButtonIcon;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_game);
userButtonIcon = findViewById(R.id.button).getBackground();
computerButtonIcon =findViewById(R.id.button4).getBackground();
emptyButtonIcon = findViewById(R.id.button2).getBackground();
runOnUiThread(new Runnable() {
@Override
public void run() {
startBoard();
}
});
}
@Override
public void onClick(final View v) {
{
int k = 0, matri[][] = new int[8][8];
for (int i = 0; i < 8; i++) {
for (int j = 0; j < 8; j++) {
if (v.getId() == squares[i][j].getId()) {
k++;
col = i; //Possible logical error check values of col and row
row = j; //Possible logical error check values of col and row
break;
}
}
if (k > 0)
break;
}
if (matrix[col][row] != 2 && matrix[col][row] != 1)
matrix[col][row] = 1; // Possible logical error check values of col and row
for (int i = 0; i < 8; i++) {
for (int j = 0; j < 8; j++) {
matri[i][j] = matrix[i][j];
}
}
new Thread(new Runnable() {
@Override
public void run() {
matrix = Processor.Move(matrix, row, col);
}
}).start();
setBoard(matrix);
new Thread(new Runnable() {
@Override
public void run() {
matrix = Processor.computerMove(matrix);
}
}).start();
setBoard(matrix);
int temp = 0;
for (int i = 0; i < 8; i++) {
for (int j = 0; j < 8; j++) {
if (matri[i][j] != matrix[i][j])
temp++;
}
}
if (temp == 0) {
runOnUiThread(new Runnable() {
@Override
public void run() {
Toast.makeText(getBaseContext(), "Illegal Move!", Toast.LENGTH_LONG).show();
}
});
}
}
}
public void setBoard ( int[][] matrix){
for (int i = 0; i < 8; i++)
for (int j = 0; j < 8; j++)
switch (matrix[i][j]) {
case 1:
squares[i][j].setBackground(userButtonIcon);
break;
case 2:
squares[i][j].setBackground(computerButtonIcon);
break;
default:
squares[i][j].setBackground(emptyButtonIcon);
break;
}
Log.i(TAG, "Board set");
SystemClock.sleep(1000);
}
private void startBoard () {
squares[0][0] = findViewById(R.id.square1x1);
squares[0][1] = findViewById(R.id.square1x2);
squares[0][2] = findViewById(R.id.square1x3);
squares[0][3] = findViewById(R.id.square1x4);
squares[0][4] = findViewById(R.id.square1x5);
squares[0][5] = findViewById(R.id.square1x6);
squares[0][6] = findViewById(R.id.square1x7);
squares[0][7] = findViewById(R.id.square1x8);
squares[1][0] = findViewById(R.id.square2x1);
squares[1][1] = findViewById(R.id.square2x2);
squares[1][2] = findViewById(R.id.square2x3);
squares[1][3] = findViewById(R.id.square2x4);
squares[1][4] = findViewById(R.id.square2x5);
squares[1][5] = findViewById(R.id.square2x6);
squares[1][6] = findViewById(R.id.square2x7);
squares[1][7] = findViewById(R.id.square2x8);
squares[2][0] = findViewById(R.id.square3x1);
squares[2][1] = findViewById(R.id.square3x2);
squares[2][2] = findViewById(R.id.square3x3);
squares[2][3] = findViewById(R.id.square3x4);
squares[2][4] = findViewById(R.id.square3x5);
squares[2][5] = findViewById(R.id.square3x6);
squares[2][6] = findViewById(R.id.square3x7);
squares[2][7] = findViewById(R.id.square3x8);
squares[3][0] = findViewById(R.id.square4x1);
squares[3][1] = findViewById(R.id.square4x2);
squares[3][2] = findViewById(R.id.square4x3);
squares[3][3] = findViewById(R.id.square4x4);
squares[3][4] = findViewById(R.id.square4x5);
squares[3][5] = findViewById(R.id.square4x6);
squares[3][6] = findViewById(R.id.square4x7);
squares[3][7] = findViewById(R.id.square4x8);
squares[4][0] = findViewById(R.id.square5x1);
squares[4][1] = findViewById(R.id.square5x2);
squares[4][2] = findViewById(R.id.square5x3);
squares[4][3] = findViewById(R.id.square5x4);
squares[4][4] = findViewById(R.id.square5x5);
squares[4][5] = findViewById(R.id.square5x6);
squares[4][6] = findViewById(R.id.square5x7);
squares[4][7] = findViewById(R.id.square5x8);
squares[5][0] = findViewById(R.id.square6x1);
squares[5][1] = findViewById(R.id.square6x2);
squares[5][2] = findViewById(R.id.square6x3);
squares[5][3] = findViewById(R.id.square6x4);
squares[5][4] = findViewById(R.id.square6x5);
squares[5][5] = findViewById(R.id.square6x6);
squares[5][6] = findViewById(R.id.square6x7);
squares[5][7] = findViewById(R.id.square6x8);
squares[6][0] = findViewById(R.id.square7x1);
squares[6][1] = findViewById(R.id.square7x2);
squares[6][2] = findViewById(R.id.square7x3);
squares[6][3] = findViewById(R.id.square7x4);
squares[6][4] = findViewById(R.id.square7x5);
squares[6][5] = findViewById(R.id.square7x6);
squares[6][6] = findViewById(R.id.square7x7);
squares[6][7] = findViewById(R.id.square7x8);
squares[7][0] = findViewById(R.id.square8x1);
squares[7][1] = findViewById(R.id.square8x2);
squares[7][2] = findViewById(R.id.square8x3);
squares[7][3] = findViewById(R.id.square8x4);
squares[7][4] = findViewById(R.id.square8x5);
squares[7][5] = findViewById(R.id.square8x6);
squares[7][6] = findViewById(R.id.square8x7);
squares[7][7] = findViewById(R.id.square8x8);
for (int i = 0; i < 8; i++)
for (int j = 0; j < 8; j++) {
squares[i][j].setOnClickListener(this);
squares[i][j].setBackground(findViewById(R.id.button2).getBackground());
Log.i(TAG, "OnCLickListener set");
}
squares[3][3].setBackground(userButtonIcon);
squares[3][4].setBackground(computerButtonIcon);
squares[4][3].setBackground(computerButtonIcon);
squares[4][4].setBackground(userButtonIcon);
matrix[3][3] = 1;
matrix[4][3] = 2;
matrix[3][4] = 2;
matrix[4][4] = 1;
}
}