Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial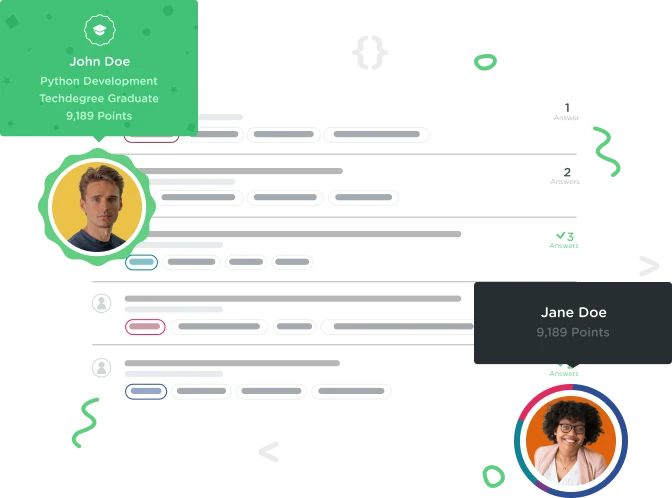
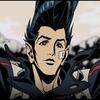
JP Tomberlin
14,317 PointsAndroid Crystal Ball App
I'm unable to get the crystal ball app to put out the random answers. Anytime I launch the emulator and click on the button it keeps giving me the answer 'Yes'. Here is what I have in MainActivity.java. Where is my mistake?
package com.example.crystalball;
import java.util.Random;
import android.app.Activity;
import android.os.Bundle;
import android.view.Menu;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Declare our View variables and assign them the Views from the layout file
final TextView answerLabel = (TextView) findViewById(R.id.textView1);
Button getAnswerButton = (Button) findViewById(R.id.button1);
getAnswerButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View arg0) {
String[] answers = {
"It is certain",
"It is decidedly so",
"All signs say yes",
"The stars are not aligned",
"My reply is NO",
"It is doubtful",
"Better not tell you now",
"Concentrate and ask again",
"Unable to answer now"};
// The button was clicked, so update the answer label with an answer
String answer = "";
// Randomly select one of three answers: Yes, No or Maybe
Random randomGenerator = new Random(); //
int randomNumber = randomGenerator.nextInt(answers.length);
answer = answers[randomNumber];
// Update the label with our dynamic answer
answerLabel.setText(answer);
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
}
1 Answer

Ernest Grzybowski
Treehouse Project ReviewerYour java looks good! My guess is that your button onClick
is never getting triggered. Can you post your xml?