Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial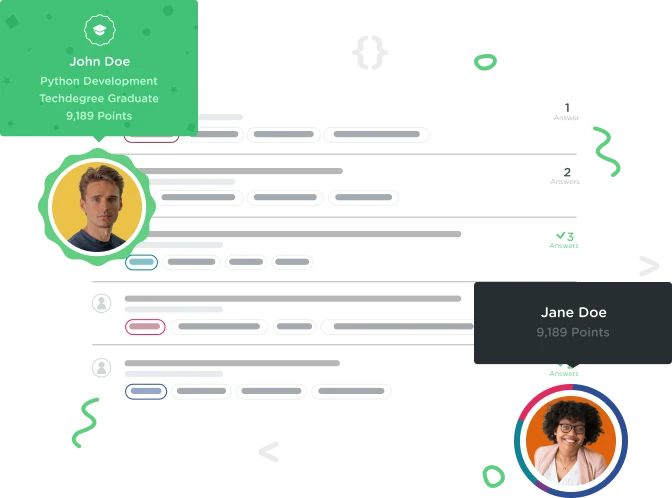
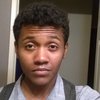
Ricky Sparks
22,249 PointsAndroid Eclipse has errors after new app( shake detector java)
package com.rickyjames1750.crystalball;
import android.hardware.Sensor;
import android.hardware.SensorEvent;
import android.hardware.SensorEventListener;
public class ShakeDetector implements SensorEventListener {
// Minimum acceleration needed to count as a shake movement
private static final int MIN_SHAKE_ACCELERATION = 5;
// Minimum number of movements to register a shake
private static final int MIN_MOVEMENTS = 2;
// Maximum time (in milliseconds) for the whole shake to occur
private static final int MAX_SHAKE_DURATION = 500;
// Arrays to store gravity and linear acceleration values
private float[] mGravity = { 0.0f, 0.0f, 0.0f };
private float[] mLinearAcceleration = { 0.0f, 0.0f, 0.0f };
// Indexes for x, y, and z values
private static final int X = 0;
private static final int Y = 1;
private static final int Z = 2;
// OnShakeListener that will be notified when the shake is detected
private OnShakeListener mShakeListener;
// Start time for the shake detection
long startTime = 0;
// Counter for shake movements
int moveCount = 0;
// Constructor that sets the shake listener
public ShakeDetector(OnShakeListener shakeListener) {
mShakeListener = shakeListener;
}
@Override
public void onSensorChanged(SensorEvent event) {
// This method will be called when the accelerometer detects a change.
// Call a helper method that wraps code from the Android developer site
setCurrentAcceleration(event);
// Get the max linear acceleration in any direction
float maxLinearAcceleration = getMaxCurrentLinearAcceleration();
// Check if the acceleration is greater than our minimum threshold
if (maxLinearAcceleration > MIN_SHAKE_ACCELERATION) {
long now = System.currentTimeMillis();
// Set the startTime if it was reset to zero
if (startTime == 0) {
startTime = now;
}
long elapsedTime = now - startTime;
// Check if we're still in the shake window we defined
if (elapsedTime > MAX_SHAKE_DURATION) {
// Too much time has passed. Start over!
resetShakeDetection();
}
else {
// Keep track of all the movements
moveCount++;
// Check if enough movements have been made to qualify as a shake
if (moveCount > MIN_MOVEMENTS) {
// It's a shake! Notify the listener.
mShakeListener.onShake();
// Reset for the next one!
resetShakeDetection();
}
}
}
}
@Override
public void onAccuracyChanged(Sensor sensor, int accuracy) {
// Intentionally blank
}
private void setCurrentAcceleration(SensorEvent event) {
/*
* BEGIN SECTION from Android developer site. This code accounts for
* gravity using a high-pass filter
*/
// alpha is calculated as t / (t + dT)
// with t, the low-pass filter's time-constant
// and dT, the event delivery rate
final float alpha = 0.8f;
// Gravity components of x, y, and z acceleration
mGravity[X] = alpha * mGravity[X] + (1 - alpha) * event.values[X];
mGravity[Y] = alpha * mGravity[Y] + (1 - alpha) * event.values[Y];
mGravity[Z] = alpha * mGravity[Z] + (1 - alpha) * event.values[Z];
// Linear acceleration along the x, y, and z axes (gravity effects removed)
mLinearAcceleration[X] = event.values[X] - mGravity[X];
mLinearAcceleration[Y] = event.values[Y] - mGravity[Y];
mLinearAcceleration[Z] = event.values[Z] - mGravity[Z];
/*
* END SECTION from Android developer site
*/
}
private float getMaxCurrentLinearAcceleration() {
// Start by setting the value to the x value
float maxLinearAcceleration = mLinearAcceleration[X];
// Check if the y value is greater
if (mLinearAcceleration[Y] > maxLinearAcceleration) {
maxLinearAcceleration = mLinearAcceleration[Y];
}
// Check if the z value is greater
if (mLinearAcceleration[Z] > maxLinearAcceleration) {
maxLinearAcceleration = mLinearAcceleration[Z];
}
// Return the greatest value
return maxLinearAcceleration;
}
private void resetShakeDetection() {
startTime = 0;
moveCount = 0;
}
/*
* Definition for OnShakeListener definition. I would normally put this
* into it's own .java file, but I included it here for quick reference
* and to make it easier to include this file in our project.
*/
public interface OnShakeListener {
public void onShake();
}
}
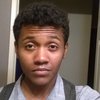
Ricky Sparks
22,249 PointsDescription Resource Path Location Type The type java.lang.Object cannot be resolved. It is indirectly referenced from required .class files ShakeDetector.java /CrystalBall/src/com/rickyjames1750/crystalball line 1 Java Problem Unable to resolve target 'android-19' until the SDK is loaded. appcompat_v7_2 Unknown Android Target Problem Unable to resolve target 'android-19' until the SDK is loaded. MasterDetailExample Unknown Android Target Problem Unable to resolve target 'android-19' until the SDK is loaded. CrystalBall Unknown Android Target Problem The type java.lang.Object cannot be resolved. It is indirectly referenced from required .class files DummyContent.java /MasterDetailExample/src/com/example/masterdetailexample/dummy line 1 Java Problem Unable to resolve target 'android-19' until the SDK is loaded. appcompat_v7 Unknown Android Target Problem Unable to resolve target 'android-19' until the SDK is loaded. Copy of CrystalBall Unknown Android Target Problem The project was not built since its build path is incomplete. Cannot find the class file for java.lang.Object. Fix the build path then try building this project CrystalBall Unknown Java Problem The type java.lang.Object cannot be resolved. It is indirectly referenced from required .class files ShakeDetector.java /Copy of CrystalBall/src/com/example/crystalball line 1 Java Problem The project was not built since its build path is incomplete. Cannot find the class file for java.lang.Object. Fix the build path then try building this project Copy of CrystalBall Unknown Java Problem Unable to resolve target 'android-19' until the SDK is loaded. MasterDetailExample Unknown Android Target Problem The project was not built since its build path is incomplete. Cannot find the class file for java.lang.Object. Fix the build path then try building this project MasterDetailExample Unknown Java Problem
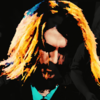
Justin Horner
Treehouse Guest TeacherIs there any chance possible that you might have also made another change in addition to adding this new file? If you remove this file from the project completely can you then build successfully?
Also, what API version are your targeting?
1 Answer

John Karanja
5,839 Pointstry deleting the shake detector file and then copy it back.
Justin Horner
Treehouse Guest TeacherJustin Horner
Treehouse Guest TeacherHello Ricky,
Thanks for posting your code! Would you mind sharing the error(s) you are seeing?
Thanks!