Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial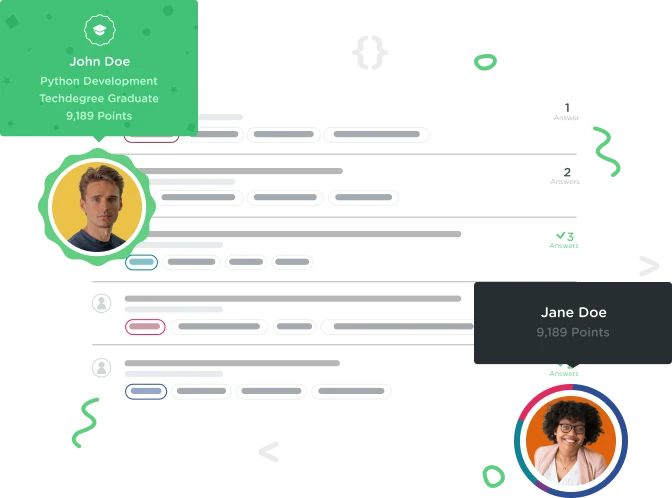

Ahmet GULER
7,181 PointsAndroid FunFacts App crashes when button is pressed.
if the Strings returns from a method then App crashes when i press the button. If string is coming from a variable or directly.. then App works
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/mainLayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="20dp"
android:background="@android:color/holo_blue_bright"
tools:context="com.example.levi.funfacts.MainActivity">
<TextView
android:id="@+id/factsTextView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:layout_centerVertical="true"
android:text="Default Text"
android:textColor="@android:color/white"
android:textSize="24sp"
tools:layout_editor_absoluteX="0dp"
tools:layout_editor_absoluteY="253dp" />
<Button
android:id="@+id/factsButton"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:text="Push Me!!!"
android:textSize="24sp"
tools:layout_editor_absoluteX="8dp"
tools:layout_editor_absoluteY="518dp" />
<TextView
android:id="@+id/ustBaslik"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/factsTextView"
android:layout_alignParentTop="true"
android:layout_alignStart="@+id/factsTextView"
android:layout_marginTop="26dp"
android:text="Did you know?"
android:textColor="@color/colorPrimaryDark"
android:textSize="30sp" />
</RelativeLayout>
package com.example.levi.funfacts;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.RelativeLayout;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity {
private Button mButton;
private TextView mText;
private RelativeLayout mLayout;
private FactBook factBook;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mButton = findViewById(R.id.factsButton);
mText = findViewById(R.id.factsTextView);
mLayout = findViewById(R.id.mainLayout);
OnClickListener listener = new OnClickListener() {
@Override
public void onClick(View view) {
mText.setText(factBook.getFact());
}
};
mButton.setOnClickListener(listener);
}
}
package com.example.levi.funfacts;
import android.graphics.Color;
import java.util.Random;
public class FactBook {
public String[] facts = {"1st fact","2nd fact","3rd fact","4th fact","5th fact"};
public String getFact() {
return "Test string abc";
}
}
3 Answers

Ahmet GULER
7,181 PointsHello,
Here is the logcat msgs.
12-26 18:40:48.299 14618-14618/com.example.levi.funfacts V/ViewRootImpl: Contents drawing finished : com.example.levi.funfacts/com.example.levi.funfacts.MainActivity
12-26 18:40:48.300 14618-14618/com.example.levi.funfacts I/Timeline: Timeline: Activity_idle id: android.os.BinderProxy@3183e65 time:497809223
12-26 18:40:49.455 14618-14618/com.example.levi.funfacts I/ViewRootImpl: ViewRoot's Touch Event : ACTION_DOWN
12-26 18:40:49.574 14618-14618/com.example.levi.funfacts I/ViewRootImpl: ViewRoot's Touch Event : ACTION_UP
12-26 18:40:49.576 14618-14618/com.example.levi.funfacts I/AudioManagerEx: AudioManagerEx created
12-26 18:40:49.582 14618-14618/com.example.levi.funfacts D/AndroidRuntime: Shutting down VM
12-26 18:40:49.583 14618-14618/com.example.levi.funfacts E/AndroidRuntime: FATAL EXCEPTION: main
Process: com.example.levi.funfacts, PID: 14618
java.lang.NullPointerException: Attempt to invoke virtual method 'java.lang.String com.example.levi.funfacts.FactBook.getFact()' on a null object reference
at com.example.levi.funfacts.MainActivity$1.onClick(MainActivity.java:30)
at android.view.View.performClick(View.java:5224)
at android.view.View$PerformClick.run(View.java:21356)
at android.os.Handler.handleCallback(Handler.java:739)
at android.os.Handler.dispatchMessage(Handler.java:95)
at android.os.Looper.loop(Looper.java:148)
at android.app.ActivityThread.main(ActivityThread.java:5585)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:730)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:620)
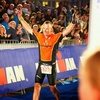
Steve Hunter
57,712 PointsHi there,
Yes, this shows that your code is throwing a NullPointerException
. This means one of your variables is pointing at nothing, i.e. it hasn't had anything assigned into it. The error further states that getFact()
is being called on that null
reference. It is. Your variable factBook
is instantiated as being of type FactBook
but never has anything assigned to it!
You need to assign something into factBook
- along the lines of:
private FactBook factBook = new FactBook();
Or do the assignment in onCreate()
but you need to put something into that variable.
Steve.

Ahmet GULER
7,181 Pointsthanks for your help. yes it worked.
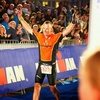
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsWhat error does you app throw? If you look through the Logcat, you should find the specific message.
Also, have you tried casting the views:
Steve.