Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial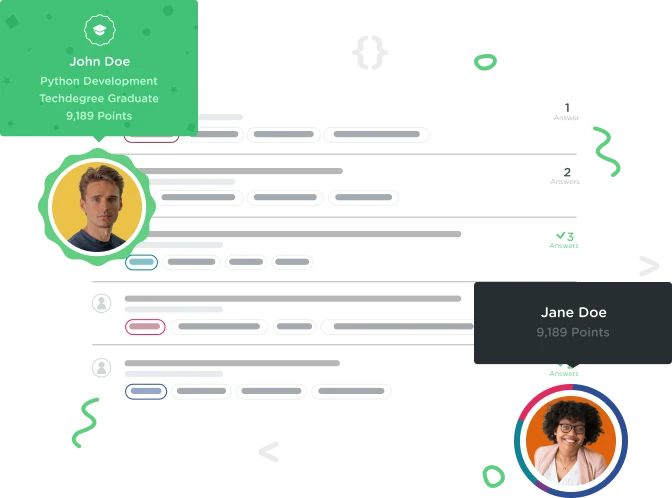

Nidhi Saini
2,627 PointsAndroid : I am using Parse as a backend and I want to save text message on the backend. How to save text mesg?
Code for creating image and text message
protected ParseObject createMessage(){
ParseObject message = new ParseObject(ParseConstants.CLASS_MESSAGES);
message.put(ParseConstants.KEY_SENDER_ID, ParseUser.getCurrentUser().getObjectId());
message.put(ParseConstants.KEY_SENDER_NAME, ParseUser.getCurrentUser().getUsername());
message.put(ParseConstants.KEY_RECIPIEN_ID, getRecipientIds());
message.put(ParseConstants.KEY_FILE_TYPE, mFileType);
if(mFileType == "image"){
byte[] fileBytes = FileHelper.getByteArrayFromFile(this, mMediaUri);
if(fileBytes == null){
return null;
}
else{//we have an image
if(mFileType.equals(ParseConstants.TYPE_IMAGE)){
//reduce the size of file and it will store it in the file bytes - 10mb is the limit
fileBytes = FileHelper.reduceImageForUpload(fileBytes);
}
String fileName =FileHelper.getFileName(this, mMediaUri, mFileType);
ParseFile file = new ParseFile(fileName, fileBytes);
message.put(ParseConstants.KEY_FILE, file);
}
}
else
{
message.put("Text File", mTextMessage);
}
return message;
}
protected void send(ParseObject message){ message.saveInBackground(new SaveCallback() {
@Override
public void done(ParseException e) {
if(e == null){
//successful
Toast.makeText(RecipientsActivity.this, R.string.success_message, Toast.LENGTH_LONG).show();
}
else{//
Log.e(TAG, e.getMessage());
Toast.makeText(RecipientsActivity.this, e.getMessage(), Toast.LENGTH_LONG).show();
/* AlertDialog.Builder builder = new AlertDialog.Builder(RecipientsActivity.this);
builder.setMessage(R.string.error_sending_message);
builder.setTitle(R.string.error_selecting_file_title);
builder.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();*/
}
}
});
}
03-17 12:15:20.352: V/Provider/Settings(13895): from settings cache , name = sound_effects_enabled , value = 0 03-17 12:15:20.356: D/AndroidRuntime(13895): Shutting down VM 03-17 12:15:20.359: W/dalvikvm(13895): threadid=1: thread exiting with uncaught exception (group=0x4195f9a8) 03-17 12:15:20.373: E/AndroidRuntime(13895): FATAL EXCEPTION: main 03-17 12:15:20.373: E/AndroidRuntime(13895): java.lang.IllegalArgumentException: value may not be null. 03-17 12:15:20.373: E/AndroidRuntime(13895): at com.parse.ParseObject.put(ParseObject.java:2536) 03-17 12:15:20.373: E/AndroidRuntime(13895): at com.turtalabs.bagit.RecipientsActivity.createMessage(RecipientsActivity.java:197) 03-17 12:15:20.373: E/AndroidRuntime(13895): at com.turtalabs.bagit.RecipientsActivity.onOptionsItemSelected(RecipientsActivity.java:158) 03-17 12:15:20.373: E/AndroidRuntime(13895): at android.app.Activity.onMenuItemSelected(Activity.java:2566) 03-17 12:15:20.373: E/AndroidRuntime(13895): at com.android.internal.policy.impl.PhoneWindow.onMenuItemSelected(PhoneWindow.java:995) 03-17 12:15:20.373: E/AndroidRuntime(13895): at com.android.internal.view.menu.MenuBuilder.dispatchMenuItemSelected(MenuBuilder.java:735) 03-17 12:15:20.373: E/AndroidRuntime(13895): at com.android.internal.view.menu.MenuItemImpl.invoke(MenuItemImpl.java:149) 03-17 12:15:20.373: E/AndroidRuntime(13895): at com.android.internal.view.menu.MenuBuilder.performItemAction(MenuBuilder.java:874) 03-17 12:15:20.373: E/AndroidRuntime(13895): at com.android.internal.view.menu.ActionMenuView.invokeItem(ActionMenuView.java:577) 03-17 12:15:20.373: E/AndroidRuntime(13895): at com.android.internal.view.menu.ActionMenuItemView.onClick(ActionMenuItemView.java:115) 03-17 12:15:20.373: E/AndroidRuntime(13895): at android.view.View.performClick(View.java:4243) 03-17 12:15:20.373: E/AndroidRuntime(13895): at android.view.View$PerformClick.run(View.java:17520) 03-17 12:15:20.373: E/AndroidRuntime(13895): at android.os.Handler.handleCallback(Handler.java:725) 03-17 12:15:20.373: E/AndroidRuntime(13895): at android.os.Handler.dispatchMessage(Handler.java:92) 03-17 12:15:20.373: E/AndroidRuntime(13895): at android.os.Looper.loop(Looper.java:153) 03-17 12:15:20.373: E/AndroidRuntime(13895): at android.app.ActivityThread.main(ActivityThread.java:5297) 03-17 12:15:20.373: E/AndroidRuntime(13895): at java.lang.reflect.Method.invokeNative(Native Method) 03-17 12:15:20.373: E/AndroidRuntime(13895): at java.lang.reflect.Method.invoke(Method.java:511) 03-17 12:15:20.373: E/AndroidRuntime(13895): at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:833) 03-17 12:15:20.373: E/AndroidRuntime(13895): at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:600) 03-17 12:15:20.373: E/AndroidRuntime(13895): at dalvik.system.NativeStart.main(Native Method)
8 Answers

Nidhi Saini
2,627 PointsI don't know what is the best way to solve this issue, but i figured out the solution by creating new class that stores text messages. Thanks teamhouse team and students for your encouragement.
protected ParseObject createTextMessage(){
ParseObject message = new ParseObject(ParseConstants.CLASS_TEXT_MESSAGES);
message.put(ParseConstants.KEY_SENDER_ID, ParseUser.getCurrentUser().getObjectId());
message.put(ParseConstants.KEY_SENDER_NAME, ParseUser.getCurrentUser().getUsername());
message.put(ParseConstants.KEY_RECIPIEN_ID, getRecipientIds());
message.put(ParseConstants.KEY_TEXT_MESSAGE, mTextMessage);
return message;
}
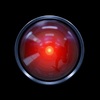
Paul Stevens
4,125 PointsHello,
I answered this question for someone else. Not much explanation in the answer i'm afraid, but it does have all the code I used so may help you.
It's on this forum thread:
https://teamtreehouse.com/forum/can-someone-elaborate-a-bit-more-on-how-to-send-simple-text
Hope it helps, Paul :)

Nidhi Saini
2,627 PointsBest so far:)

Nidhi Saini
2,627 PointsHi Paul,
Thanks for sharing this link. It is really helpful.
I have some questions Q What is this My MessageAdapter code doing ? Q Where should i add the code for - My MessageAdapter ?
I really appreciate your help. Thanks Again
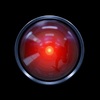
Paul Stevens
4,125 PointsHello,
It may be that you haven't got to the part that deals with the message adapter yet. Ben shows you how to create the MessageAdapter class in the tutorials in the section called "Retrieving and Viewing Messages". The videos that deal with the Message Adapter are called "Creating a Custom List Adapter for Messages"
That bit of code will make more sense when you have got to these videos.
Paul :)

Nidhi Saini
2,627 Pointsyep....Just started with Retrieving and Viewing Messages.

Nidhi Saini
2,627 PointsHi Paul, Please help me fix this issue. Thanks.
onCreate from RecipientsActivity:
//get image or video message
mMediaUri =getIntent().getData();
mFileType = getIntent().getExtras().getString(ParseConstants.KEY_FILE_TYPE);
//get text message
mTextMessage = getIntent().getExtras().getString("TextMessage");
createMessage
protected ParseObject createMessage(){
ParseObject message = new ParseObject(ParseConstants.CLASS_MESSAGES);
message.put(ParseConstants.KEY_SENDER_ID, ParseUser.getCurrentUser().getObjectId());
message.put(ParseConstants.KEY_SENDER_NAME, ParseUser.getCurrentUser().getUsername());
message.put(ParseConstants.KEY_RECIPIEN_ID, getRecipientIds());
message.put(ParseConstants.KEY_FILE_TYPE, mFileType);
if(mFileType.equals(ParseConstants.TYPE_IMAGE)){
byte[] fileBytes = FileHelper.getByteArrayFromFile(this, mMediaUri);
if(fileBytes == null){
return null;
}
else{//we have an image
if(mFileType.equals(ParseConstants.TYPE_IMAGE)){
//reduce the size of file and it will store it in the file bytes - 10mb is the limit
fileBytes = FileHelper.reduceImageForUpload(fileBytes);
}
String fileName =FileHelper.getFileName(this, mMediaUri, mFileType);
ParseFile file = new ParseFile(fileName, fileBytes);
message.put(ParseConstants.KEY_FILE, file);
return message;
}
}
else{
message.setObjectId(mTextMessage);
message.put(ParseConstants.KEY_FILE_TYPE, "text");
message.put(ParseConstants.KEY_FILE, mTextMessage);
return message;
}
}
Error
03-20 11:07:14.238: E/AndroidRuntime(2133): java.lang.IllegalArgumentException: value may not be null. 03-20 11:07:14.238: E/AndroidRuntime(2133): at com.parse.ParseObject.put(ParseObject.java:2536) 03-20 11:07:14.238: E/AndroidRuntime(2133): at com.turtalabs.bagit.RecipientsActivity.createMessage(RecipientsActivity.java:214) 03-20 11:07:14.238: E/AndroidRuntime(2133): at com.turtalabs.bagit.RecipientsActivity.onOptionsItemSelected
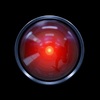
Paul Stevens
4,125 PointsHello,
Here is my createMessage Method:
protected ParseObject createMessage(){
ParseObject message = new ParseObject(ParseConstants.CLASS_MESSAGES);
message.put(ParseConstants.KEY_SENDER_ID, ParseUser.getCurrentUser().getObjectId());
message.put(ParseConstants.KEY_SENDER_NAME, ParseUser.getCurrentUser().getUsername());
message.put(ParseConstants.KEY_RECIPIENT_IDS, getRecipientIds());
message.put(ParseConstants.KEY_FILE_TYPE, mFileType);
//If the message is a text message then set the message and leave method before converting the file to bytes.
if (mFileType.equals(ParseConstants.TYPE_TEXT)) {
message.put(ParseConstants.KEY_MESSAGE, mMyMessage);
message.put(ParseConstants.KEY_FILE_TYPE, ParseConstants.TYPE_TEXT);
return message;
}else{
//******************************************************************************************************************************************
byte[] fileBytes = FileHelper.getByteArrayFromFile(this, mMediaUri);
if (fileBytes == null) {
return null;
}else {
if(mFileType.equals(ParseConstants.TYPE_IMAGE)) {
fileBytes = FileHelper.reduceImageForUpload(fileBytes);
}
String fileName = FileHelper.getFileName(this, mMediaUri, mFileType);
ParseFile file = new ParseFile(fileName, fileBytes);
message.put(ParseConstants.KEY_FILE, file);
return message;
}
}
}
I leave my method with the return line before converting the file into bytes if it is a text message. This may be your problem. I think if you continue into the file conversion one of the values (pretty sure it's mMediaUri) is a null because we didn't set it for the text message.
Hope this helps,
Paul :)