Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial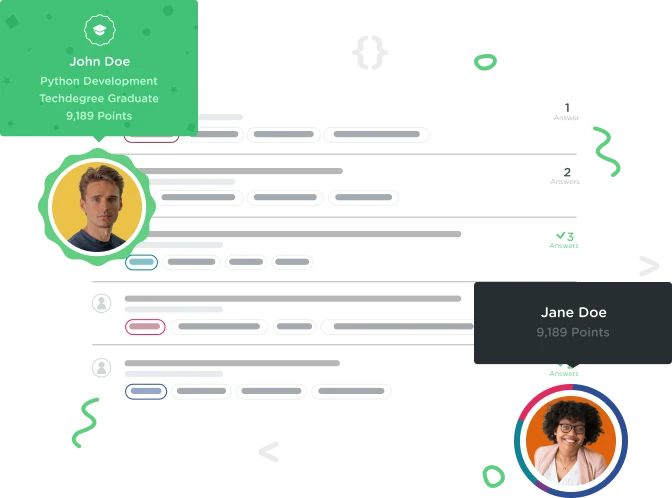
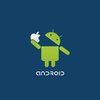
Jared M
3,680 PointsAndroid ListView Scrolling Scrambling
Hello all. This doesn't have to do with any Treehouse course or anything but just listViews in general. My ListView works fine except when I scroll fast some of the items are duplicated and in random order. It's kind of hard to explain but here's the beginning of my getView() method.
@Override
public View getView(final int position, View convertView, ViewGroup arg2) {
View view;
if (convertView == null) {
LayoutInflater inflater = (LayoutInflater) context
.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
view = inflater.inflate(R.layout.list_item, null);
}else{
view = convertView;
}.......
In my MainActivity I call notifySetAdapterChanged(). Anyone know how to fix this?
Here is what the Logcat says each getView():
-Layouts #:1 and position is 0
Layouts #:1 and position is 0 Layouts #:2 and position is 1 Layouts #:3 and position is 2 Layouts #:4 and position is 3 Layouts #:5 and position is 4 Layouts #:6 and position is 5 Layouts #:7 and position is 6 Layouts #:8 and position is 7 Layouts #:9 and position is 8 //click one of the list items Layouts #:10 and position is 0 Layouts #:11 and position is 1 Layouts #:12 and position is 2 Layouts #:13 and position is 3 Layouts #:14 and position is 4 Layouts #:14 and position is 5 Layouts #:14 and position is 6 Layouts #:14 and position is 7 Layouts #:14 and position is 8 Layouts #:14 and position is 0 Layouts #:14 and position is 1 Layouts #:14 and position is 2 Layouts #:14 and position is 3 Layouts #:14 and position is 4 Layouts #:14 and position is 5 Layouts #:14 and position is 6 Layouts #:14 and position is 7
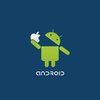
Jared M
3,680 PointsHere is my whole getView method. In my MainActivty I call notifyDataSetChanged() whenever I need the list altered and I even try to clear all the layouts every time.
@Override
public View getView(final int position, View convertView, ViewGroup arg2) {
View view;
if (convertView == null) {
LayoutInflater inflater = (LayoutInflater) context
.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
view = inflater.inflate(R.layout.list_item, arg2, false);
}else{
view = convertView;
}
//task textview
TextView taskTextView = (TextView) view.findViewById(R.id.textView);
taskTextView.setTextSize(tinyDB.getInt(SettingsActivity.TEXT_SIZE, 0));
taskTextView.setText(tasks.get(position));
//layout background
final RelativeLayout layout = (RelativeLayout) view.findViewById(R.id.layout);
//sortButton
final ImageButton sortButton = (ImageButton) view.findViewById(R.id.sortButton);
if(itemLayouts.size() < tasks.size()) {
itemLayouts.add(position, layout);
sortButtonLayouts.add(position, sortButton);
}else{
itemLayouts.set(position, layout);
sortButtonLayouts.set(position, sortButton);
}
Log.d("Item Layouts: ", "Layouts #:" + itemLayouts.size() + " and position is " + position);
sortButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (sortItemPosition == position) {
Log.e("Method ", "1");
//same item already selected, so deselect
sortItemIsSelected = false;
sortItemPosition = -1;
MainActivity.makeSortButtonsInvisible();
layout.setBackgroundColor(context.getResources().getColor(R.color.background_material_light));
sortButton.setBackgroundColor(context.getResources().getColor(R.color.background_material_light));
MainActivity.makeRoomForListView();
} else if (sortItemIsSelected) {
Log.e("Method ", "2");
//other item is already selected
//deselct selected layout
itemLayouts.get(sortItemPosition).setBackgroundColor(context.getResources().getColor(R.color.background_material_light));
sortButtonLayouts.get(sortItemPosition).setBackgroundColor(context.getResources().getColor(R.color.background_material_light));
sortItemPosition = position;
sortItemIsSelected = true;
layout.setBackgroundColor(context.getResources().getColor(R.color.lightGray));
sortButton.setBackgroundColor(context.getResources().getColor(R.color.lightGray));
} else {
Log.e("Method ", "3");
//select item
sortItemPosition = position;
sortItemIsSelected = true;
MainActivity.makeSortButtonsVisible();
layout.setBackgroundColor(context.getResources().getColor(R.color.lightGray));
sortButton.setBackgroundColor(context.getResources().getColor(R.color.lightGray));
MainActivity.makeRoomForButtons();
}
}
});
//checkbox
CheckBox checkBox = (CheckBox) view.findViewById(R.id.checkBox);
checkBox.setChecked(false);
checkBox.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) {
}
});
return view;
}
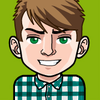
Harry James
14,780 PointsThanks Jared!
I'm going to run this code on my side when I get the time and see if I can reproduce it so that hopefully, we'll see how to prevent this from happening.
Get back to you soon! :)
1 Answer
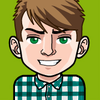
Harry James
14,780 PointsHello again Jared!
I've been trying this out through the last 2 days but haven't been able to reproduce the problem. I've built a test app and set up my code exactly as you stated in the first post and the ListView scrolls fine, even upon a reset.
This leads me to believe that it must be something that you're doing in the setting part. From the logcat you provided above, nothing seems strange about the position - that is going up as expected so, every part of the list is being loaded (Here, I'm assuming you reset it back to the start twice).
Please go ahead and check your setting parts of your code and change some things around in there to see if it fixes the problem.
It might also be worth moving your OnClickListener's elsewhere if possible. Right now, they're in your getView()
method which is called every time a new view is put on the screen so, this is very inefficient and means that you're creating a new instance of an OnClickListener for each item on the screen. If you're setting things in your ListView in these listener's, they may be causing the problem (I'm not sure how your ListView is set up as I don't know what each of your variables are set to so, you'll know whether you are or not). It may be the fact that multiple instances of the OnClick are listening and therefore, all running their own code (Which would be very bad!). Even if this isn't the case, I'd still recommend you move the listeners.
If you still need help and need this fixed, feel free to send me your Project Files on here and I'll take a look and see if I can reproduce and then fix the problem from there. I understand that this may not be your thing however, and if it isn't, don't feel obliged to do so (Some people have private projects that they wouldn't want to share publicly on the internet).
Anyhow, I wish you the best of luck on your app and hopefully this will prove helpful. If there's anything else I can help you with, give me a shout :)
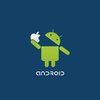
Jared M
3,680 PointsHow would you recommend I share it? I tried Github but it's really confusing.
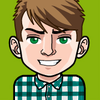
Harry James
14,780 PointsHey Jared!
To do this, right-click on your project in Android Studio and press Show in Explorer. Then, upload your project directory into a service like Google Drive, Mega.co.nz, SkyDrive or another service of your choice.
Then, share the project via a URL and I'll download it and try and reproduce your problem, then find a solution.
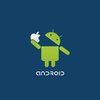
Jared M
3,680 PointsI tried out Mega.co.nz, here's the link.
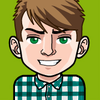
Harry James
14,780 PointsThanks Jared!
I'll take a look and get back to you soon :)
Edit: Sorry about the delay - been busy recently. I am still going to take a look!
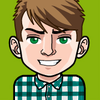
Harry James
14,780 PointsHey again Jared!
Sorry about the huge delay there. Been busy with exams and QA'ing a new course so things got pushed behind quite a bit.
Anyhow, I've made a few changes over here so let me know how this goes for you.
I've commented out some code with no function, set up a new ViewHolder system (Should put less strain on resources and prevent the occasional crashes I was getting before) and made a few other minor tweaks.
I've left one thing to do before your ListView system is working which is to reset the text size each time it is changed. I tried looking around for a way to do this but couldn't actually think of a good way to go about it. Hopefully you might know how to do so though and if anything pops into my head I'll let you know.
Hope it helps and if there's anything else, give me a shout :)
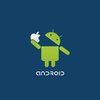
Jared M
3,680 PointsThank you Harry for sticking with me. I tried the code you gave me and some things seemed to work better but it still has the problem when you select an item and move it up or down and when you scroll the items get all mixed up and multiple items are suddenly selected looking. Anyways, I'm kind of done working on this app but if you still want to try to solve the problem feel free to do so. Thanks for being so helpful.
Harry James
14,780 PointsHarry James
14,780 PointsHey Jared!
Do you mind if I see where you're setting the values for your views? I wonder if something strange is going on there.