Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial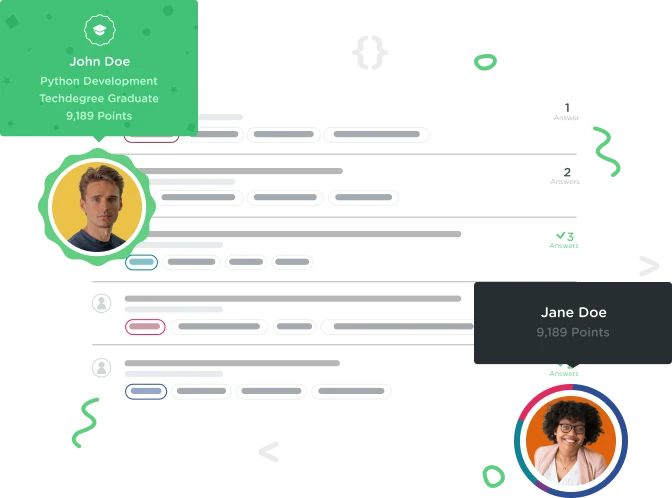

Casey Bell
2,604 PointsAndroid Parse.com Help
From a ListView I want a user to click on an item which will bring them to a “dashboard” where they are able to create data specific to the ParseObject they clicked on through the ListView. I am assuming that I need to pass the object Id of the item clicked to the dashboard activity. How do I go about doing this?
For instance, I am creating a Parse.com Class called projects which contains a project name and the user who created the project. The listview shows the project name and when the user clicks on the project name they enter all the data only related to the project. I am not sure how to set up the backend on Parse. Do I create a class called projects only and have subclasses of the data related to each project? Or do I create other classes that relate to a specific project? I am new to this so any help is appreciated.
1 Answer

Nick Edwards
Courses Plus Student 15,766 PointsHi Casey,
When you create an Intent to start a new activity, you can pass through extra data to that activity. In your case, you can pass through the ParseObject's ID (presuming you've already saved the ParseObject to Parse.com and have it referenced):
String projectId = myParseObject.getObjectId();
Intent intent = new Intent(context, DashboardActivity.class);
intent.putExtra("id", projectId);
startActivity(intent);
In your Dashboard activity, you can then retrieve that data:
Intent intent = getIntent();
projectId = intent.getStringExtra("id");
Now that you have your ParseObject's ID, you can use ParseQuery to retrieve the ParseObject:
ParseQuery query = new ParseQuery("Projects");
query.whereEqualTo("objectId", projectId);
query.findInBackground(myFindCallback);
In your FindCallback's done() method you'll have access to a List containing the specified project as a ParseObject. You can now reference this and add data to it.
As for the other part, I'm still a bit unsure as to what it is exactly you're trying to achieve, but I'll try my best to offer some advice. Nothing really needs to be set up on the backend - one of the big advantages to using it is that Parse will automatically create a new class when you create a new object.
Obviously how you set up your class(es) for Parse depends on how you want your data organised (ie. is the list of projects being displayed dependent on someone being logged in?), but you'll probably want it to be as uniform as possible to prevent cluttering things up with multiple classes. For the sake of example you could simply use the class Projects you've created on Parse and create a new object for it:
myProject = ParseObject("Projects");
// If the Projects class doesn't exist on Parse, it will be created when this ParseObject is saved
and then when you reference that in your Dashboard activity, you can simply add data to any existing fields (or create new fields), for example:
myProject.put("projectAuthor", authorName); // project's author
myProject.put("projectDescription" projectDesc); // project description
ParseObject's are pretty dynamic, so fields will be added automatically on Parse when the object is saved.
I hope this helps you a bit - let me know if you need anything else!
Casey Bell
2,604 PointsCasey Bell
2,604 PointsThank you for response! This is very helpful.
To dive a little deeper in the functionality of the app, the user logs in and is presented the list view of the projects that they are related to or have created. In this activity, users can also create more projects. The user will access the project through the listview and can do several things within it such as write worker information and write reports for the project. Users can also add other users to the project.
That being said, I need to figure out how to structure my data. Like you said, I do not want to clutter. Thanks again for the help!
Nick Edwards
Courses Plus Student 15,766 PointsNick Edwards
Courses Plus Student 15,766 PointsGlad to be of help!
Okay, in that case you'll also want to look at ParseRelations which are used for establishing many-to-many relationships for ParseObjects. One reason is that you can use this for keeping track of projects that are associated with multiple users, and users that are associated with multiple projects You can then use Parse queries to retrieve all X associated with Y.
Another reason is the possibility that your data structure might require creating a separate class for something like worker information which could be associated with multiple projects (again, this is entirely based on what data you need and how you decide to structure things).
Casey Bell
2,604 PointsCasey Bell
2,604 PointsI have another questions regarding this. I am able to obtain the project object ID and pass it to my main dashboard activity through my list view of projects. I created a fragment where the user can create workers specific to the project by getting the object id String passed to it.
However, In a separate Activity (not a fragment/ProjectDashBoard), I want to view the workers that have been uploaded. When I try to get the string (KEY_PROJECT_OBJECT_ID) that is being passed, there is no data available because I am only passing it to my dashboard main activity (where my fragment can get the intent).
My question is, how do I pass the project object id to several classes within the application? I have tried to create an Application Class for the global String, but there is no way I have found to call getIntent in the application class. Note that when a user adds a worker, the worker info is for data only (First Name, Last Name, Company) and they will not be “users” within the application. Is there a way to get out of passing this object id and use ParseRelation that I am not considering? I have gone through the ParserRelation documentation and have not found what I am looking for. Thank you again for your help!