Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial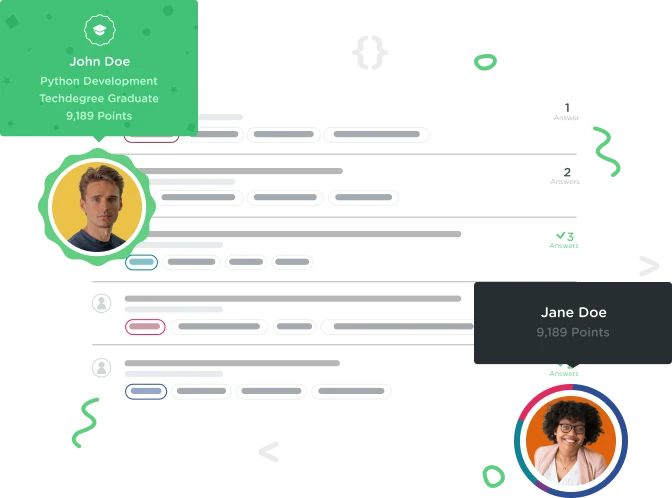
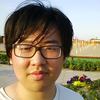
Luigi Zhou
16,121 PointsAndroid Studio - Alert Dialog does not appear
I'm using Android Studio and I don't understand why the Alert Dialog doesn't appear on the screen when the emulator (even the phone) is in airplane mode.
Here's the code i have written so far:
protected String[] mBlogPostTitles;
public static final int NUMBER_OF_POSTS = 20;
public static final String TAG = MainListActivity.class.getSimpleName();
protected JSONObject mBlogData;
protected ProgressBar mProgressBar;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main_list);
mProgressBar = (ProgressBar) findViewById(R.id.progressBar);
if(isNetworkAvailable()){
mProgressBar.setVisibility(View.VISIBLE);
GetBlogPostsTask getBlogPostsTask = new GetBlogPostsTask();
getBlogPostsTask.execute();
}
else{
Toast.makeText(this,"Network is unavailable",Toast.LENGTH_LONG);
}
//Toast.makeText(this, getString(R.string.no_items);, Toast.LENGTH_LONG).show();
}
private boolean isNetworkAvailable() {
ConnectivityManager manager = (ConnectivityManager) getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo networkInfo = manager.getActiveNetworkInfo();
boolean isAvailable = false;
if(networkInfo != null && networkInfo.isConnected()){
isAvailable = true;
}
return isAvailable;
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu_main_list, menu);
return true;
}
private void handleBlogResponse() {
mProgressBar.setVisibility(View.INVISIBLE);
if(mBlogData == null){
updateDisplayForError();
}
else{
try {
JSONArray jsonPosts = mBlogData.getJSONArray("posts");
mBlogPostTitles = new String[jsonPosts.length()];
for(int i = 0; i < jsonPosts.length();i++){
JSONObject post = jsonPosts.getJSONObject(i);
String title = post.getString("title");
title = Html.fromHtml(title).toString();
mBlogPostTitles[i] = title;
}
ArrayAdapter<String> adapter = new ArrayAdapter<String>(this,android.R.layout.simple_list_item_1,mBlogPostTitles);
setListAdapter(adapter);
} catch (JSONException e) {
Log.e(TAG,"Exception Caught!",e);
}
}
}
private void updateDisplayForError() {
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setTitle(getString(R.string.error_title));
builder.setMessage(getString(R.string.error_message));
builder.setPositiveButton(android.R.string.ok,null);
AlertDialog dialog = builder.create();
dialog.show();
TextView emptyTextView = (TextView) getListView().getEmptyView();
emptyTextView.setText(getString(R.string.no_items));
}
private class GetBlogPostsTask extends AsyncTask<Object, Void, JSONObject> {
@Override
protected JSONObject doInBackground(Object[] params) {
int responseCode = -1;
JSONObject jsonResponse = null;
try{
URL blogFeedUrl = new URL("http://blog.teamtreehouse.com/api/get_recent_summary/?count="+NUMBER_OF_POSTS);
HttpURLConnection connection = (HttpURLConnection) blogFeedUrl.openConnection();
connection.connect();
responseCode = connection.getResponseCode();
if(responseCode == HttpURLConnection.HTTP_OK){
InputStream inputStream = connection.getInputStream();
Reader reader = new InputStreamReader(inputStream);
int nextCharacter; // read() returns an int, we cast it to char later
String responseData = "";
while(true){ // Infinite loop, can only be stopped by a "break" statement
nextCharacter = reader.read(); // read() without parameters returns one character
if(nextCharacter == -1) // A return value of -1 means that we reached the end
break;
responseData += (char) nextCharacter; // The += operator appends the character to the end of the string
}
jsonResponse = new JSONObject(responseData);
}
else {
Log.i(TAG, "Unsuccessful HTTP Response Code: " + responseCode);
}
}
catch(MalformedURLException e){
Log.e(TAG, "Exception caught: ", e);
} catch (IOException e) {
Log.e(TAG, "Exception caught: ", e);
} catch (Exception e){
Log.e(TAG, "Exception caught: ", e);
}
return jsonResponse;
}
@Override
protected void onPostExecute(JSONObject result) {
mBlogData = result;
handleBlogResponse();
}
}
2 Answers
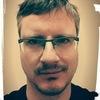
Steve Bedard
14,335 Pointsthis class (GetBlogPostsTask) only get called when network is available (airplane mode shut off networks) so handleBlogResponse() in the onPostExecute never gets called too so is updateDisplayForError()

Wesley Seago
10,424 PointsThe Toast doesn't appear because you never call the show() method on it.
Your line:
Toast.makeText(this,"Network is unavailable",Toast.LENGTH_LONG);
You need to add the show method:
Toast.makeText(this,"Network is unavailable",Toast.LENGTH_LONG).show();
Also, below this line you have a Toast message commented out:
//Toast.makeText(this, getString(R.string.no_items);, Toast.LENGTH_LONG).show();
I assume this was commented out because it also didn't work. You need to remove the ";".
//Toast.makeText(this, getString(R.string.no_items), Toast.LENGTH_LONG).show();
As Steve pointed out above, because the isAvailable returned false, execution ends after the toast message is called.
Luigi Zhou
16,121 PointsLuigi Zhou
16,121 PointsThank you for answering, but I checked and in the video tutorial the getBlogPostsTask class is called inside the if statement and in the emulator the Toast in the else statement does not appear.
Steve Bedard
14,335 PointsSteve Bedard
14,335 PointsAre you talking about the Toast or Dialog ? They are different things.
Wesley Seago
10,424 PointsWesley Seago
10,424 PointsBoth.
The toast doesn't get called because he has a code error.
The dialog doesn't get called because once onCreate is run, when isAvailable returns false, execution stops.
If he fixes the Toast message by calling the show() method on it, it will display the toast before execution stops, but as you stated before, the code to show the dialog will never be reached if the network is unavailable.
Luigi Zhou
16,121 PointsLuigi Zhou
16,121 PointsThank you both!
Like you pointed out, i forgot to call the show() method on the Toast, and the problem was in fact that all the code was in the if and was never called when there was no network.
I have fixed the problem now, thank you very much!
I gave best answer to Steve as he addressed the original problem, but if I could, i would have given best answer to the both of you :)
Again, thank you very much!
PS: I hope my english is understandable :)