Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial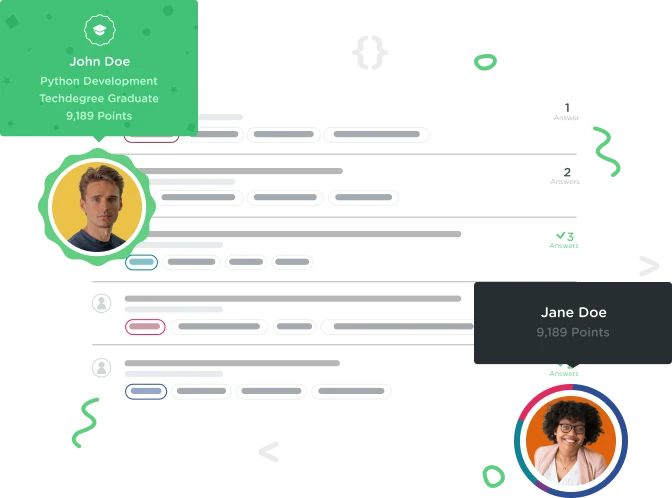

Andre Colares
5,437 PointsAndroid Studio - CODE UNTIL HERE
This is the functional code until this video.
4 Answers
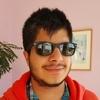
faraz
Courses Plus Student 21,474 PointsAndre,
Please clarify about what your problem is, I'm having trouble understanding. Thanks!

Andre Colares
5,437 PointsI'm just posting the code until this video... Because the video is outdated. I'm using android studio and facing a lot of problems for every video of this course. When this videos will be updated?

TheBigoso /\
3,217 PointsHi Faraz,
Do you think you can help me out.
my program compiles fine. I can take a picture or a 10 sec video. Then I press ok on the picture or video, Im able to choose my friends from a list of my added friends.
The problem is that there is no actionbar displayed and with no actionbar there is no send button displayed as it is involved with the actionbar. Since the release of this video things have been deprecated and the course is outdated.
Do you have any informantion on how to deal with my problem so i can move onto the next part.
Thank you, TheBigOso
here is my github https://github.com/TheBigOso/RibbitSendButton
RecipientsActivity.Java
package com.fartyou.thedirtyappstore.ribbit2;
import android.app.AlertDialog;
import android.app.ListActivity;
import android.os.Bundle;
import android.util.Log;
import android.view.MenuItem;
import android.view.Window;
import android.widget.ArrayAdapter;
import android.widget.ListView;
import com.parse.FindCallback;
import com.parse.ParseException;
import com.parse.ParseRelation;
import com.parse.ParseUser;
import java.util.List;
public class RecipientsActivity extends ListActivity {
public static final String TAG = RecipientsActivity.class.getSimpleName();
protected List<ParseUser> mFriends;
protected ParseRelation<ParseUser> mFriendsRelation;
protected ParseUser mCurrentUser;
protected MenuItem mSendMenuItem;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
requestWindowFeature(Window.FEATURE_INDETERMINATE_PROGRESS);
setContentView(R.layout.activity_recipients);
getListView().setChoiceMode(ListView.CHOICE_MODE_MULTIPLE);
}
@Override
public void onResume() {
super.onResume();
mCurrentUser = ParseUser.getCurrentUser();
mFriendsRelation = mCurrentUser.getRelation(ParseConstants.KEY_FRIENDS_RELATION);
mFriendsRelation.getQuery().findInBackground(new FindCallback<ParseUser>() {
@Override
public void done(List<ParseUser> friends, ParseException e) {
if (e == null) {
mFriends = friends;
String[] usernames = new String[mFriends.size()];
int i = 0;
for (ParseUser user : mFriends) {
usernames[i] = user.getUsername();
i++;
}
ArrayAdapter<String> adapter = new ArrayAdapter<String>(
getListView().getContext(),
android.R.layout.simple_list_item_checked,
usernames);
setListAdapter(adapter);
} else {
Log.e(TAG, e.getMessage());
AlertDialog.Builder builder = new AlertDialog.Builder(RecipientsActivity.this);
builder.setMessage(e.getMessage())
.setTitle(R.string.error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}
}
activity_recipients.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:paddingBottom="@dimen/activity_vertical_margin"
tools:context="com.fartyou.thedirtyappstore.ribbit2.RecipientsActivity">
<ListView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@android:id/list"
android:layout_alignParentTop="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"/>
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@android:id/empty"
android:text="@string/empty_recipients_list_message"
/>
</RelativeLayout>

Andre Colares
5,437 PointsYou need to set your action bar theme @ Android Manifest.

TheBigoso /\
3,217 Pointsi added the action bar to the android manifest should it look like this?
thank you so much for your response
thebigoso
<activity android:name=".RecipientsActivity"
android:screenOrientation="portrait"
android:theme="@style/Theme.AppCompat.Light.DarkActionBar">
here is my full manifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.fartyou.thedirtyappstore.ribbit2" >
<uses-sdk
android:minSdkVersion="14"
android:targetSdkVersion="19" />
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<android:uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<android:uses-permission android:name="android.permission.READ_PHONE_STATE" />
<android:uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
<android:uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<uses-feature
android:name="android.hardware.camera"
android:required="true" />
<application
android:name=".RibbitApplication"
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:screenOrientation="portrait"
android:supportsRtl="true"
android:theme="@style/AppTheme" >
<meta-data
android:name="com.parse.APPLICATION_ID"
android:value="@string/parse_app_id" />
<meta-data
android:name="com.parse.CLIENT_KEY"
android:value="@string/parse_client_key" />
<activity
android:name=".MainActivity"
android:label="@string/app_name"
android:screenOrientation="portrait"
android:theme="@style/AppTheme.NoActionBar" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<activity
android:name=".LoginActivity"
android:screenOrientation="portrait" >
</activity>
<activity
android:name=".SignUpActivity"
android:parentActivityName=".LoginActivity"
android:screenOrientation="portrait" >
</activity>
<activity
android:name=".EditFriendsActivity"
android:screenOrientation="portrait" >
</activity>
<activity android:name=".RecipientsActivity"
android:screenOrientation="portrait"
android:theme="@style/Theme.AppCompat.Light.DarkActionBar">
</activity>
</application>
</manifest>

Andre Colares
5,437 Points@[TheBigoso /](https://teamtreehouse.com/ryanruddell)
Check out this complete tutorial, is the one I used: http://www.recursiverobot.com/post/59267986367/setting-up-the-new-actionbarcompat-in-android

Andre Colares
5,437 PointsI added this project to github:
Andre Colares
5,437 PointsAndre Colares
5,437 PointsFriendsFragment
SECTIONPAGERADAPTER.JAVA
EDITFRIENDSFRAGMENT.JAVA
EDITFRIENDSACTIVITY