Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial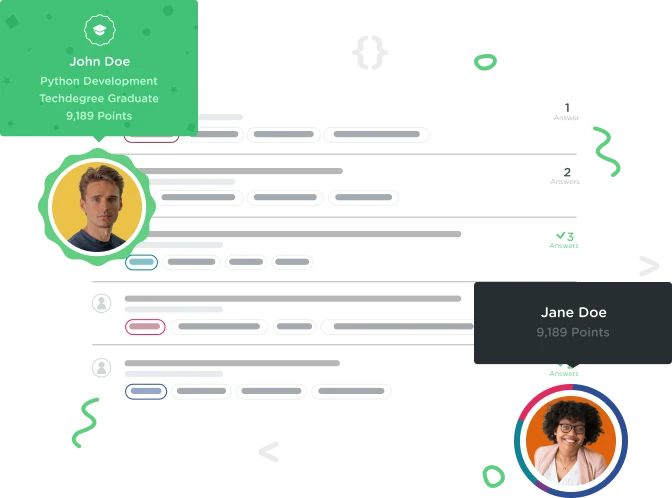
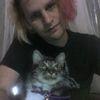
Bryan Wiedeman
10,203 Pointsandroid.content.res.Resources$NotFoundException: String resource ID #0x0
The app is crashing when I try to run it. I think this issue has something to do with the fact that I started out working on this on my own, and thus named my fields different things than you did, but I tried to ferret out a place where I accidentally put your name instead of my own but couldn't find one. Here's my ListAdapter.Java since that seems to be the only class it's erroring on that I had any hand in.
package me.paxana.android.golfscoreapptake2;
import android.content.Context;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.BaseAdapter;
import android.widget.Button;
import android.widget.TextView;
/**
* Created by paxie on 12/8/15.
*/
public class ListAdapter extends BaseAdapter {
private final Context mContext;
private final HoleScores[] mHoleScores;
public ListAdapter (Context context, HoleScores[] holeScores) {
mContext = context;
mHoleScores = holeScores;
}
@Override
public int getCount() {
return mHoleScores.length;
}
@Override
public Object getItem(int position) {
return mHoleScores[position];
}
@Override
public long getItemId(int position) {
return 0;
}
@Override
public View getView(final int position, View convertView, ViewGroup parent) {
final ViewHolder holder;
if (convertView == null) {
convertView = LayoutInflater.from(mContext).inflate(R.layout.list_item, null);
holder = new ViewHolder();
holder.holeNumber = (TextView) convertView.findViewById(R.id.holeNumber);
holder.holeScore = (TextView) convertView.findViewById(R.id.holeScore);
holder.plusButton = (Button) convertView.findViewById(R.id.plusButton);
holder.minusButton = (Button) convertView.findViewById(R.id.minusButton);
convertView.setTag(holder);
}
else {
holder = (ViewHolder) convertView.getTag();
}
holder.holeNumber.setText(mHoleScores[position].getLabel());
holder.holeScore.setText(mHoleScores[position].getHoleScore());
holder.minusButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
int updatedHoleScore = mHoleScores[position].getHoleScore() - 1;
if (updatedHoleScore < 0) updatedHoleScore = 0;
mHoleScores[position].setHoleScore(updatedHoleScore);
holder.holeScore.setText(updatedHoleScore + "");
}
});
holder.plusButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
int updatedHoleScore = mHoleScores[position].getHoleScore() + 1;
mHoleScores[position].setHoleScore(updatedHoleScore);
holder.holeScore.setText(updatedHoleScore + "");
}
});
return convertView;
}
private static class ViewHolder {
TextView holeNumber;
TextView holeScore;
Button plusButton;
Button minusButton;
}
}
2 Answers

Seth Kroger
56,413 Points// if getHoleScore() returns an int, then you'd need to change it to a String first...
holder.holeScore.setText(mHoleScores[position].getHoleScore());
// to this
holder.holeScore.setText( Integer.toString(mHoleScores[position].getHoleScore()) );
// or use smushing or your conversion method of choice.

Fernando Hernandez
11,013 PointsHi Bryan,
I had this same error pop up. It looks like you (like me) didn't convert the int to a string in the method that was missed at 6:46 of the video, and later fixed 8:54.
More concretely, here at this block of code:
holder.holeNumber.setText(mHoleScores[position].getLabel());
holder.holeScore.setText(mHoleScores[position].getHoleScore());
holder.minusButton.setOnClickListener(new View.OnClickListener() {
@Override
If you want to follow the same syntax, can change to:
holder.holeNumber.setText(mHoleScores[position].getLabel());
holder.holeScore.setText(mHoleScores[position].getHoleScore() + "");
holder.minusButton.setOnClickListener(new View.OnClickListener() {
@Override
As Seth pointed out, this would work too:
holder.holeScore.setText( Integer.toString(mHoleScores[position].getHoleScore()) );
Or this:
holder.strokeCount.setText( String.valueOf(mHoleScores[position].getHoleScore())) );
I hope this helps a bit!
Thanks, F