Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial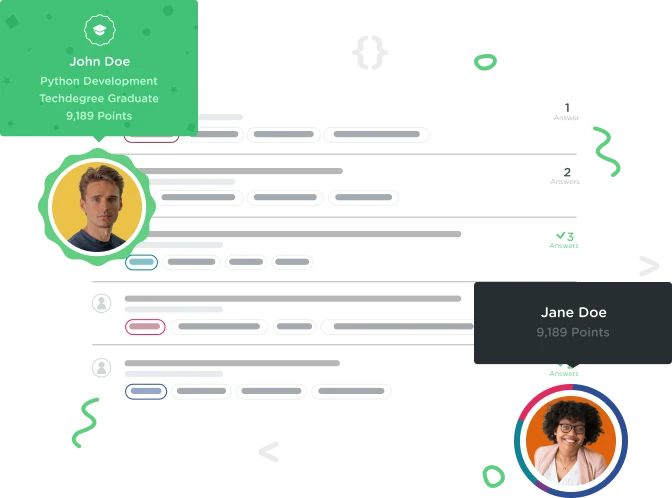

Christopher Stewart
7,531 Pointsandroid.content.res.Resources$NotFoundException: String resource ID #0x7f0c0013
It works just fine when empty:
package com.teamtreehouse.testingbase;
import android.os.Build;
import android.view.inputmethod.EditorInfo;
import org.junit.Before;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.robolectric.Robolectric;
import org.robolectric.RobolectricGradleTestRunner;
import org.robolectric.annotation.Config;
import static org.junit.Assert.*;
@RunWith(RobolectricGradleTestRunner.class)
@Config(constants = BuildConfig.class, sdk = Build.VERSION_CODES.LOLLIPOP)
public class MainActivityTest {
MainActivity mMainActivity;
@Before
public void setUp() throws Exception {
//mMainActivity = Robolectric.setupActivity(MainActivity.class);
}
@Test
public void editTextUpdatesTextView() throws Exception {
/* // Arrange
String givenString = "test123";
mMainActivity.editText.setText(givenString);
// Act
mMainActivity.editText.onEditorAction(EditorInfo.IME_ACTION_DONE);
// Assert
String actualString = mMainActivity.textView.getText().toString();
assertEquals(givenString, actualString);*/
}
}
Using RoboElectric 3.1
dependencies {
compile fileTree(dir: 'libs', include: ['*.jar'])
testCompile 'junit:junit:4.12'
compile 'com.android.support:appcompat-v7:23.4.0'
testCompile "org.mockito:mockito-core:1.+"
testCompile 'org.robolectric:robolectric:3.1'
}
However, when I un-comment the setUp method I get this error
at android.content.res.Resources.getText(Resources.java:274)
at android.content.res.Resources.getString(Resources.java:360)
at android.content.Context.getString(Context.java:376)
at org.robolectric.util.ActivityController.getActivityTitle(ActivityController.java:120)
at org.robolectric.util.ActivityController.attach(ActivityController.java:61)
at org.robolectric.util.ActivityController.of(ActivityController.java:32)
at org.robolectric.Robolectric.buildActivity(Robolectric.java:82)
at org.robolectric.Robolectric.buildActivity(Robolectric.java:78)
at org.robolectric.Robolectric.setupActivity(Robolectric.java:86)
at com.teamtreehouse.testingbase.MainActivityTest.setUp(MainActivityTest.java:25)
at org.junit.runners.model.FrameworkMethod$1.runReflectiveCall(FrameworkMethod.java:50)
at org.junit.internal.runners.model.ReflectiveCallable.run(ReflectiveCallable.java:12)
at org.junit.runners.model.FrameworkMethod.invokeExplosively(FrameworkMethod.java:47)
at org.junit.internal.runners.statements.RunBefores.evaluate(RunBefores.java:24)
at org.robolectric.RobolectricTestRunner$2.evaluate(RobolectricTestRunner.java:265)
at org.robolectric.RobolectricTestRunner.runChild(RobolectricTestRunner.java:191)
at org.robolectric.RobolectricTestRunner.runChild(RobolectricTestRunner.java:56)
at org.junit.runners.ParentRunner$3.run(ParentRunner.java:290)
at org.junit.runners.ParentRunner$1.schedule(ParentRunner.java:71)
at org.junit.runners.ParentRunner.runChildren(ParentRunner.java:288)
at org.junit.runners.ParentRunner.access$000(ParentRunner.java:58)
at org.junit.runners.ParentRunner$2.evaluate(ParentRunner.java:268)
at org.robolectric.RobolectricTestRunner$1.evaluate(RobolectricTestRunner.java:157)
at org.junit.runners.ParentRunner.run(ParentRunner.java:363)
at org.junit.runner.JUnitCore.run(JUnitCore.java:137)
at com.intellij.junit4.JUnit4IdeaTestRunner.startRunnerWithArgs(JUnit4IdeaTestRunner.java:68)
at com.intellij.rt.execution.junit.IdeaTestRunner$Repeater.startRunnerWithArgs(IdeaTestRunner.java:51)
at com.intellij.rt.execution.junit.JUnitStarter.prepareStreamsAndStart(JUnitStarter.java:242)
at com.intellij.rt.execution.junit.JUnitStarter.main(JUnitStarter.java:70)
at com.intellij.rt.execution.application.AppMainV2.main(AppMainV2.java:131)
Looks like it can't find the resource string for the title? The string appears to exist in res/values/strings.xml
I am using Android Studio 3.0.1.
I did need to set the working directory and delete the .gradle folder to get it to succeed while empty.
Any ideas?
4 Answers

vicentelee
6,662 PointsTake a look at the top answer here https://stackoverflow.com/questions/45922916/robolectric-resourcesnotfoundexception-string-resource-id-with-android-gradle
setting android.enableAapt2=false
in the gradle.properties
file worked for me

Boban Talevski
24,793 PointsHad the same problem and what worked for me from the stackoverflow link mentioned in the other answer (the first thing I actually tried) is adding this to the build.gradle file
android {
//..
testOptions {
unitTests {
includeAndroidResources = true
}
}
}
Btw, I'm using the latest version of Robolectric currently available, not the SNAPSHOT release though.
testCompile 'org.robolectric:robolectric:3.6.1'
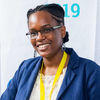
Hannah Olukoye
247 PointsNow using testImplementation 'org.robolectric:robolectric:4.3.1'

Sattha Puangput
249 PointsFollow the latest setup of Roboelectric work for me http://robolectric.org/getting-started/#building-with-android-studiogradle
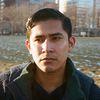
Omar Carino
11,162 PointsI just want to add to solutions above.
1) Make sure you're using the latest version of Android StudioI updated mine to (3.4) 2) You may have to update compileSdkVersion and targetSdkVersion to 28 3) You may have to update your buildToolsVersion to '28.0.3'
After all, that is set you should be able to add the solution mentioned above and it should all run smoothly!