Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial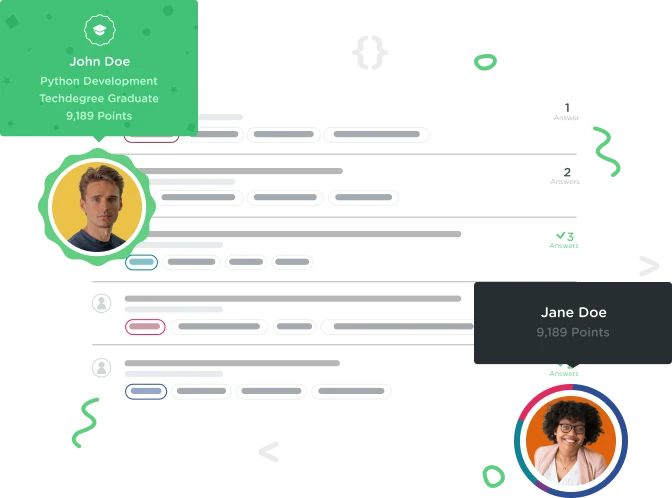

Tinashe Gwariro
4,691 Pointsandroid.support.v7.view.menu.MenuItemImpl cannot be cast to android.content.Context
I am trying to open a new activity in my app from a popup menu which shows two options one to view homes and another to book home. Each option should open a new activity using an intent.
However Im getting this error message in the android monitor. java.lang.ClassCastException: android.support.v7.view.menu.MenuItemImpl cannot be cast to android.content.Context
here is the code: public class HouseAdapter extends RecyclerView.Adapter<HouseAdapter.MyViewHolder> {
//private member variables
private Context mContext;
private List<Houses> mHousesList;
//Create new subclass for MyViewHolder which extends Recycler
public class MyViewHolder extends RecyclerView.ViewHolder {
//public member variables
public TextView title, location, count;
public ImageView thumbnail, overflow;
public MyViewHolder(View itemView) {
super(itemView);
title = (TextView) itemView.findViewById(R.id.houseTitle);
location = (TextView) itemView.findViewById(R.id.city_location);
count = (TextView) itemView.findViewById(R.id.bedCount);
thumbnail = (ImageView) itemView.findViewById(R.id.dzimba_thumbnail);
overflow = (ImageView) itemView.findViewById(R.id.overflow);
}
}
public HouseAdapter(Context mContext, List<Houses> mHouseList) {
this.mContext = mContext;
this.mHousesList =mHouseList;
}
//Instantiates the item layout file and view holder
@Override
public MyViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View itemView = LayoutInflater.from(parent.getContext())
.inflate(R.layout.house_card_view, parent, false);
return new MyViewHolder(itemView);
}
//Loads the data (images & texts) at the specified position on the cardviews
@Override
public void onBindViewHolder(final MyViewHolder holder, int position) {
Houses houses = mHousesList.get(position);
holder.title.setText(houses.getHouseName());
holder.location.setText(houses.getCity());
holder.count.setText(houses.getNumOfBeds() + " beds");
//use the glide library to load the house images
Glide.with(mContext).load(houses.getThumbnail()).into(holder.thumbnail);
holder.overflow.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
showPopupMenu(holder.overflow);
}
});
}
/**
* Showing popup menu when tapping on 3 dots
* @param view
*/
private void showPopupMenu(View view) {
//inflate menu
PopupMenu popup = new PopupMenu(mContext, view);
MenuInflater inflater = popup.getMenuInflater();
inflater.inflate(R.menu.bookings_menu, popup.getMenu());
popup.setOnMenuItemClickListener(new MyHomeMenuItemClickListener());
popup.show();
}
//the listenr class for the popup menu
class MyHomeMenuItemClickListener implements PopupMenu.OnMenuItemClickListener {
public MyHomeMenuItemClickListener(){
}
@Override
public boolean onMenuItemClick(MenuItem menuItem) {
switch (menuItem.getItemId()) {
case R.id.bookings_page:
Intent intent = new Intent((Context) menuItem, BookingPayments.class);
mContext.startActivity(intent);
return true;
case R.id.view_homes_menu:
return true;
default:
}
return false;
}
}
@Override
public int getItemCount() {
return mHousesList.size();
}
}
Tinashe Gwariro
4,691 PointsTinashe Gwariro
4,691 PointsThis is where im facing the difficulty
@Override public boolean onMenuItemClick(MenuItem menuItem) { switch (menuItem.getItemId()) { case R.id.bookings_page: Intent intent = new Intent((Context) menuItem, BookingPayments.class); mContext.startActivity(intent); return true; }