Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial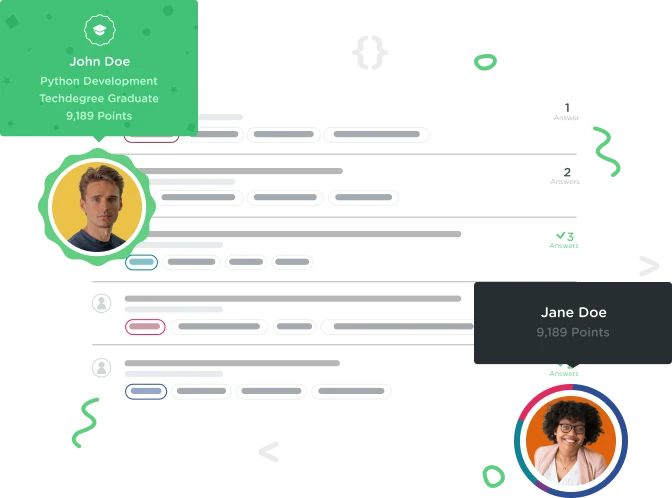

mimik2
14,766 Pointsandroid:text = "Can I make it a function?"
Hi All, I am trying to make an app in android where the top left text will read Question 1 of 10 for example. The problem is I want it to update and not just say question 1 of 10 for all questions. I was thinking about a function that does: "Question" + int[i] + "of" + questions.length
How can I do that?
1 Answer
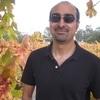
Kourosh Raeen
23,733 PointsYou don't have to set the text of that TextView in the xml layout file. You can set it dynamically with java code. Give the TextView an id in the xml file:
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/questionNumberTextView" />
Then in the java file get a reference to the TextView using findViewById:
TextView textView = (TextView) findViewById(R.id.questionNumberTextView);
Now, you can set the text:
textView.setText("Question " + questionNumber + " of" + questions.length);
assuming that you have an int variable questionNumber and an array called questions.