Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial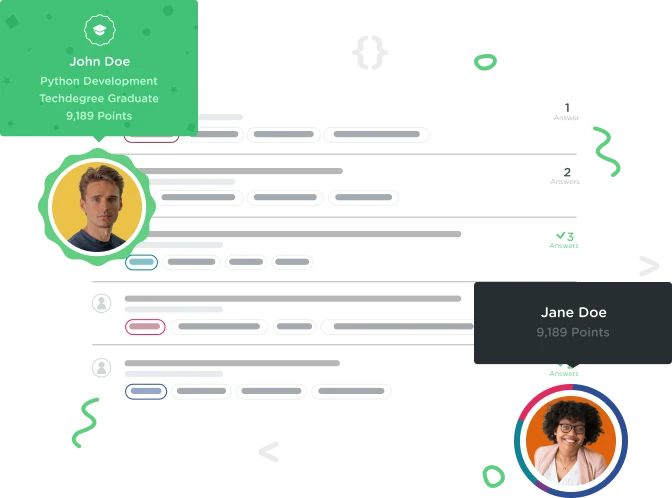
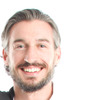
Jef DeWitt
26,460 PointsAngularJS Expression Not Displaying
I'm simply trying to get an AngularJS expression to display on screen. However, nothing shows up between the curly braces. I've inspected the app with ng-inspector and although I see an object being created with an ng-model directive, I can't display the value with the object key.
Furthermore, for testing purposes, I can't even get a simple math expression to display either.
Here's what I'm working with.
<!doctype html>
<html lang="en">
<head>
<title></title>
<link href='styles/main.css' rel='stylesheet' type="text/css"> -->
</head>
<body ng-app="angularApp">
<div ng-controller="firstCtrl">
<input ng-model="project.completed" type="checkbox">
<input ng-model="project.title" type="text" placeholder="Project Title">
<label>
{{project.title}}
1+2={{1 + 2}}
</label>
<input ng-model="project.time" type="text" placeholder="Project Time">
<label for="">{{project.time}}</label>
<button ng-click="helloWorld()">Press Me</button>
</div>
<script src="/vendor/angular.js" type="text/javascript"></script>
<script src="/scripts/app.js" type="text/javascript"></script>
</body>
</html>
...and here's the controller:
angular.module('angularApp', [])
.controller('firstCtrl', function($scope) {
$scope.helloWorld = function() {
console.log('You just pressed the button.');
};
$scope.project = {
completed :false,
title :'test',
};
});
The only thing that shows up in the label is '1+2='. I'm happy to provide more code but I believe this may be all that's necessary.
UPDATE: After spending a ridiculous amount of time trying to debug this I have been able to get the first value of the math expression to display -- the '1'. I achieved this by adding a space around the '+' operator. Still, the full expression is not evaluating.
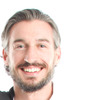
Jef DeWitt
26,460 PointsThanks for taking a look Thomas. It's in a Jekyll project and I've had success using ng-bind as a means to display ng-model values but I still have had no luck with evaluating expressions.
1 Answer
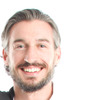
Jef DeWitt
26,460 PointsIf you're using another templating engine, such as Twig, Liquid, or Django, the curly braces may be being stripped out. This results in the values not displaying or evaluating properly.
The solution I found is editing the interpolation characters or $interpolateProvider like so inside your controller:
angular.module('angularApp', []).config(function($interpolateProvider){
$interpolateProvider.startSymbol('{[{').endSymbol('}]}');
})
Then, just wrap your expression in the new symbols, e.g.:
{[{ 1+2 }]}
...or
{[{ project.title }]}
Thomas Nilsen
14,957 PointsThomas Nilsen
14,957 PointsPlease show us your entire .html-file. I think you forgot to include something there.