Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial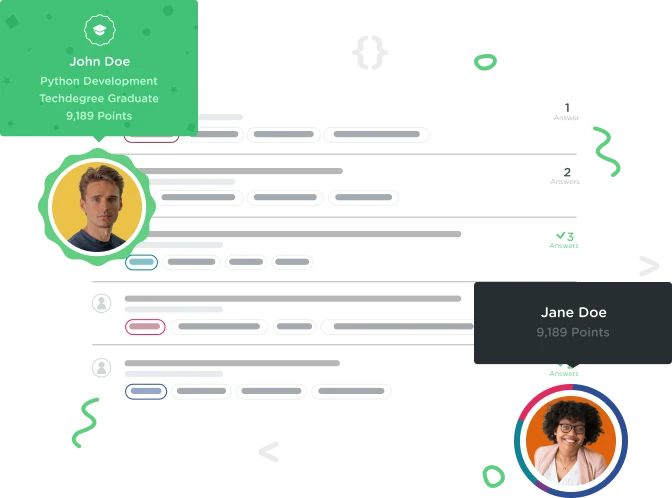

Kip Yin
4,847 PointsAngularJS service returns undefined
I just finished learning AngularJS and started working on my own project. Here it is:
The Code
'use strict';
angular.module("lifeTableLookup", [])
.service("lifeTableData", ($http) => {
// ISSUE: The controller cannot "find" `lifeTableData`.
this.getData = (callback) => {
$http.get("mock/lifeTables.json").then(callback);
};
console.log(typeof this.getData); // function
})
.controller("mainCtrl", [ "lifeTableData", ($scope, lifeTableData) => {
console.log(typeof lifeTableData); // undefined
lifeTableData.getData( (response) => {
console.log("Retriving data")
$scope.tables = response.data;
});
$scope.ILT = $scope.tables.ILT;
$scope.SUSM = $scope.tables.SUSM;
console.log("it's working");
}]);
I was following along with this sample code on treehosue.
The Issue
The issue is that in .controller()
method, lifeTableData
is undefined
. I've tried changing all arrow notations to normal function notations and dropping the square brackets in .controller
method, but nothing is working.
I was initially getting Error: $injector:unpr
, and added the square brackets according to the official guide, but it doesn't seem to help.
Anything I'm missing?
2 Answers

Thomas Nilsen
14,957 PointsThe square-brackets has to contain the same arguments, in exactly the same order:
.controller("mainCtrl", [ "$scope", "lifeTableData", ($scope, lifeTableData) => {
console.log(typeof lifeTableData); // undefined
lifeTableData.getData( (response) => {
console.log("Retriving data")
$scope.tables = response.data;
});
$scope.ILT = $scope.tables.ILT;
$scope.SUSM = $scope.tables.SUSM;
console.log("it's working");
}]);

Thomas Nilsen
14,957 PointsMost likely because you need to return the data:
this.getData = (callback) => {
return $http.get("mock/lifeTables.json").then(callback);
};

Kip Yin
4,847 PointsTried that.
angular.module("lifeTableLookup")
.controller("mainCtrl", [ "$scope", "loadLifeTable", ($scope, loadLifeTable) => {
console.log(typeof loadLifeTable); // undefined
loadLifeTable.getData((response) => {
$scope.tables = response.data;
console.log($scope.tables);
});
}])
.service("loadLifeTable", ["$http", ($http) => {
this.getData = (callback) => {
return $http.get("mock/lifeTables.json").then(callback);
};
}]);
loadLifeTable
becomes an empty object, and I get an error message:
Error: loadLifeTable.getData is not a function. (In 'loadLifeTable.getData((response) => {
$scope.tables = response.data;
console.log($scope.tables);
})', 'loadLifeTable.getData' is undefined)
I used .factory(/* ... */)
instead of .service(/* ... */)
and it worked. But I still don't understand why .service
won't work, even though I followed the exact steps in the tutorial.
Kip Yin
4,847 PointsKip Yin
4,847 PointsI realized that and added the injection but the issue persists. I got an empty object instead.