Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial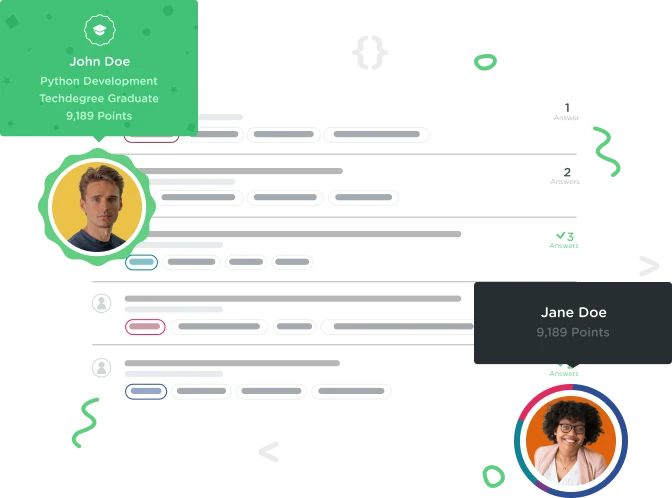
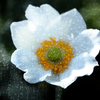
ellie adam
26,377 PointsAnimal.noise Q 3 of 3
from animal import Animal
class Sheep(Animal):
sound = "Meow"
pass
def animal.noise():
return self.sound.lower()
def Sheep.noise():
return Sheep.noise.upper()
from animal import Animal
class Sheep(Animal):
sound = "Meow"
pass
def animal.noise():
return self.sound.lower()
def Sheep.noise():
return Sheep.noise.upper()
error is task one is not passing any more.
2 Answers

Dan Johnson
40,533 PointsWhen you're overriding the methods of a parent class you only need to match the function signature. So in order to override noise you'll use this signature:
def noise(self):
# Return the uppercased sound string
Don't forget to make sure the method is indented properly. Also you can get rid of pass
it's only needed as a placeholder.
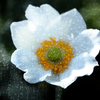
ellie adam
26,377 PointsI get this error now.. Bummer! Expected "MEOW", got "meow".
from animal import Animal
class Sheep(Animal):
sound = "Meow"
def noise(self):
return Sheep.noise.upper()

Dan Johnson
40,533 PointsYour noise method still needs to be indented to be in the class block. Also you'll need to use noise to deal with the string.
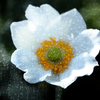
ellie adam
26,377 PointsHow to deal with string?

Dan Johnson
40,533 PointsRefer to sound using self
and then call upper on it. Return the result.
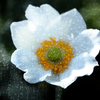
ellie adam
26,377 PointsI tried few different things nothing works.
from animal import Animal
class Sheep(Animal):
sound = "Meow"
def noise(self):
return self.upper()
def Sheep.noise(self):
return self.upper()
def noise(self):
return sheep.upper()
:(

Dan Johnson
40,533 Pointsself
refers to the instance and sound is a property / instance variable / member variable. So to access it you'd do this:
# Accessing the sound property to call upper on it.
self.sound.upper()
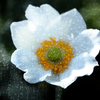
ellie adam
26,377 Pointsfrom animal import Animal
class Sheep(Animal):
sound = "Meow"
def noise(self):
self.sound.upper()
it won't pass.
from animal import Animal
class Sheep(Animal):
sound = "Meow"
def noise(self):
return self.sound.upper()
it won't let me pass.

Dan Johnson
40,533 PointsCareful with the indentation. Also you still need the return:
from animal import Animal
class Sheep(Animal):
sound = "Meow"
def noise(self):
return self.sound.upper()
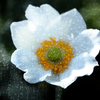
ellie adam
26,377 Pointsthanks Dan!
ellie adam
26,377 Pointsellie adam
26,377 Pointsthanks for helping me out!