Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial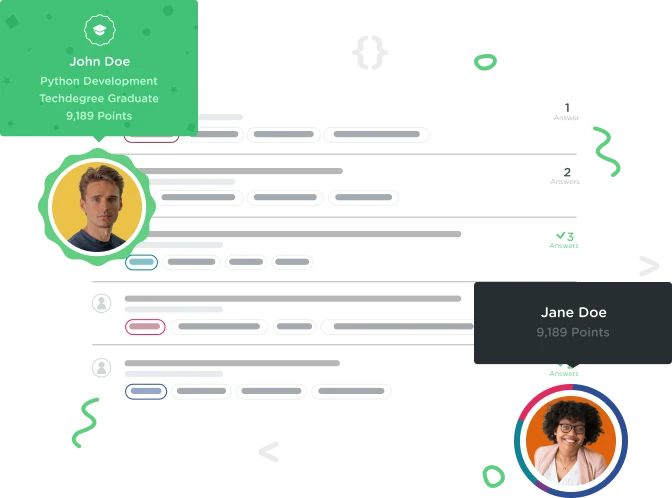
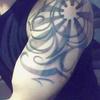
Paolo Scamardella
24,828 PointsAnonymous Function vs Function Declaration
Just a side note for those who wants to understand the difference between Anonymous Function(Expression Function) vs Function Declaration(Normal Function). Jim Hoskins prefers using Anonymous Function over Function Declaration, and he stated why in the video. No matter which way you prefer, at the end, you will end up with a function. I prefer Function Declaration over Anonymous Function because there is a difference behind the scene as to how a Function Declaration and an Anonymous Function are created. A Function Declaration is loaded before any code executes, so the JavaScript engine does what's called hoisting. It hoist the Function Declaration to the top, so it loads it first. This means that you can execute the function anywhere in your code. With Function Declaration you can call the function before or after declaring the function. With Anonymous Function, you will get an error if you try calling it before you declare it.
Example:
declarationFunction(); // This will work
function declarationFunction(){
}
declarationFunction(); // This will work too
///////////////////////////////////////////////////////////////////////////////////////////////////////////
anonymousFunction(); // You'll get an error!!!!
var anonymousFunction = function(){
}
anonymousFunction(); // This will work
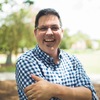
Dave McFarland
Treehouse TeacherAnother weird thing about function expressions is that you can actually give them named functions, and call them using that name. For example, you could re-write your code like this:
var yell = function shout(n){
return n > 0 ? shout(n-1) + "a" : "hiy";
}
if (yell(4) == "hiyaaaa") {
console.log("I R JSNinja!");
}
Weird...
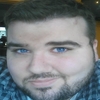
Marcus Parsons
15,719 PointsDo I detect sarcasm for some reason? Because it really seems like you're being sarcastic with me, and I have not a single clue why.
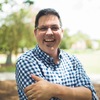
Dave McFarland
Treehouse TeacherSorry Marcus Parsons
No sarcasm intended. I just meant to point out that one can also give a name to a function, even when assigning it to a variable using a function expression. And that name could be used to call the function recursively like with your example.
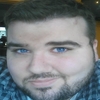
Marcus Parsons
15,719 PointsOh okay. I'm sorry, as well. I took that the wrong way. It's been a long couple of weeks fraught with misery...sorry for the confusion.

John Magee
Courses Plus Student 9,058 PointsRecursion is the act of calling a function upon itself
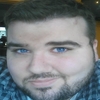
Marcus Parsons
15,719 PointsIt sure is, John!
1 Answer
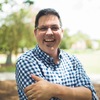
Dave McFarland
Treehouse TeacherIf a line begins with function
it is a declaration; otherwise it is an expression.
Declaration:
function sayHi() {
alert('Hi!');
}
Expression:
var sayHi = function() {
alert('Hi!');
};
Notice that there's a semicolon at the end of a function expression -- because this is really an assignment to a variable this code is a statement and should have a semicolon at the end.
The main difference between the two is that a function declaration can be placed ANYWHERE in a script including at the bottom of the script. The browser's JavaScript interpreter reads the script before running anything and commits the function to memory. Because of this it can be called anywhere in the script -- even BEFORE the declaration actually appears in the script. For example, this works:
sayHi();
function sayHi() {
alert('Hi');
};
A function expression, on the other hand, is only created during runtime -- in other words you can't call the function before until the JavaScript interpreter evaluates (or runs) the expression. For example this WON'T work"
sayHi();
var sayHi = function() {
alert('Hi');
};
To avoid all this confusion, it's often a good idea to declare your functions at the beginning of a script when possible.
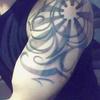
Paolo Scamardella
24,828 PointsHi Dave, You are right! It was a mistake, and I totally forgot to add a semi-colon at the end of the Expression Function (Anonymous Function). Also, I agree with declaring functions at the beginning of a script when possible. I just wanted to point out a minor difference between Declaration Function vs Expression (Anonymous) Function that wasn't discuss in the video to help others.
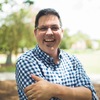
Dave McFarland
Treehouse TeacherSorry if my post sounded critical. It wasn't meant to be. I just wanted to expand on your initial discussion (which is a great point to bring up) for other students.
Keep up the good work!
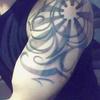
Paolo Scamardella
24,828 PointsDave, You didn't sound critical at all! I actually prefer someone pointing out my mistakes over someone who doesn't. I learn from my mistakes.
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsAnother cool feature of naming functions in JavaScript is that you can call that function you've declared from inside itself like so: