Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial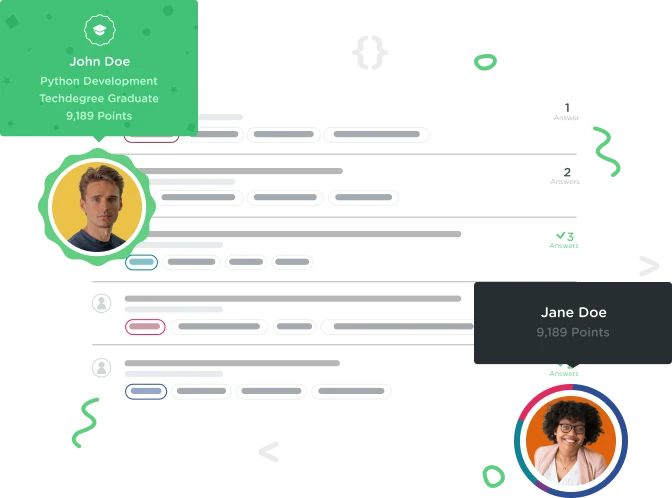
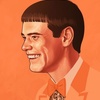
Mike Fondario
11,286 PointsAnonymous Functions Clarification
Can someone please give me another explanation of the significance or practical application of the anonymous function?
Does it have to do with scope? It seems like they're called the same way, and there doesn't seem to be a difference in how they're executed.
2 Answers
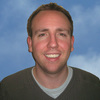
Ian Robinson
337 PointsHi Mike,
It does make handling scope easier sometimes.
I also like anonymous functions because it makes reading the JavaScript easier.
For example, in jQuery if you fade out a DOM element, you might want to do something else after it disappears, such as set a flag value to be used elsewhere in your code. Creating a named function here would be unnecessary and arguably, harder to read in a large application.
For example, I would do something like this:
var paragraphHidden = false;
$("#myParagaph").fadeOut("slow", function() {
paragraphHidden = true;
});
Although the code below would also work, I wouldn't normally do this, unless I thought my function setFlag might be used elsewhere in my application:
var paragraphHidden = false;
$("#myParagaph").fadeOut("slow", setFlag());
function setFlag() {
paragraphHidden = true;
}
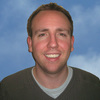
Ian Robinson
337 PointsHi Mike,
This article explains anonymous functions a little, i.e. "Not having to set a name for an anonymous function is just a convenience thing since in most cases the name of the function doesn’t really matter. Most of the time anonymous functions and named functions will both do any job perfectly well."
I also find anonymous functions useful when creating an object that contains methods/functions inside it, to do certain things.
A great example of this can be found in John Resig's excellent book. Below I've shown a modified version of his example from the book:
<script>
function Ninja() {
var feints = 0;
this.getFeints = function() {
return feints;
};
this.feint = function() {
feints++;
};
}
var ninja_1 = new Ninja();
var ninja_2 = new Ninja();
ninja_1.feint(); // increments the fients variable inside the Ninja object
alert(ninja_1.getFeints()); // displays the number of feints, i.e. 1 feint
alert(ninja_2.getFeints()); // displays the number of feints in our second ninja, i.e. 0 feints
</script>
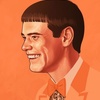
Mike Fondario
11,286 PointsThanks Ian, your links provided the distinction I was looking for. I'll check out that book.