Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial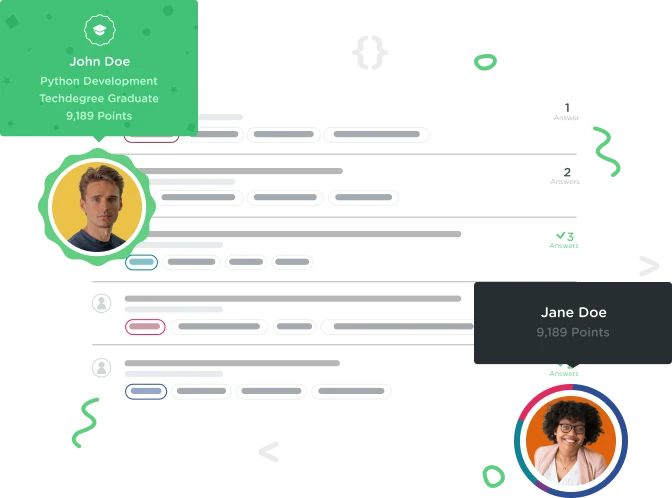

Piers FC
10,402 PointsAnother poor question - I can't understand from the compiler errors what I am doing wrong - swift 2.0 changing
Let's get some practice working with views and colors! In the editor below you have a view controller subclass.
Add a stored property to the view controller named blueColor and assign an instance of UIColor as the default value.
You can use UIColor's RGB initializer method and pass the values 0,0,255,1.0 for red, green, blue and alpha respectively. Alternatively, UIColor has a convenience method that you can use: UIColor.blueColor().
This is my answer, I have tried several different ones
class ViewController: UIController { /* declaring the stored property with an Instance of UIColor as the default value using the convenience method */ let blueColor = UIColor.blueColor()
override func viewDidLoad() {
// Do any additional setup after loading the view, typically from a nib.
}
override func didReceiveMemoryWarning() {
// Dispose of any resources that can be recreated
}
}
I then get this error
swift_lint.swift:48:23: error: use of undeclared type 'UIController' class ViewController: UIController { ^~~~~~~~~~~~ swift_lint.swift:52:19: error: method does not override any method from its superclass override func viewDidLoad() { ~~~~~~~~ ^ swift_lint.swift:56:19: error: method does not override any method from its superclass override func didReceiveMemoryWarning() {
Any idea why Treehouse can become so frustrating / what is the solution to this problem?
class ViewController: UIController {
override func viewDidLoad() {
// Do any additional setup after loading the view, typically from a nib.
}
override func didReceiveMemoryWarning() {
// Dispose of any resources that can be recreated
}
let blueColor = UIColor()
view.backgroundColor = blueColor(red: 0, green: 0, blue: 255, alpha: 1.0)
}
4 Answers

Chase Marchione
155,055 PointsHi Piers FC, and Martin,
What got me through the first challenge was declaring and initializing blueColor by using the RGB initializer method and passing in the provided values that resemble a blue color. Then for the second challenge, I stored the statement that changes the view's backgroundColor within the viewDidLoad method (which is where properties of the view or view controller object get initialized.)
class ViewController: UIViewController {
let blueColor = UIColor(red: 0, green: 0, blue: 255, alpha: 1.0)
override func viewDidLoad() {
// Do any additional setup after loading the view, typically from a nib.
view.backgroundColor = blueColor
}
override func didReceiveMemoryWarning() {
// Dispose of any resources that can be recreated
}
}
Hope this helps!
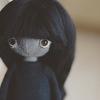
Asma Al-Marrikhi
45,524 Pointsclass ViewController: UIViewController {
let blueColor = UIColor.blue
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
}

Martin Granger
14,521 PointsUnfortunately, I noticed a typo in the challenge code. "UIController" should be "UIViewController". I tried
class ViewController: UIViewController {
let blueColor = UIColor(red: 0, green: 0, blue: 255, alpha: 1.0)
override func viewDidLoad() {
// Do any additional setup after loading the view, typically from a nib.
view.backgroundColor = blueColor
}
override func didReceiveMemoryWarning() {
// Dispose of any resources that can be recreated
}
}

Piers FC
10,402 PointsHi Guys
Thanks for the help and explanations. I can see where I was going wrong CJ, so thanks. I was trying to do both in the first challenge as well, which didn't help.
I have another question on AutoLayout which would be great if you could look at.
Cheers
Martin Granger
14,521 PointsMartin Granger
14,521 PointsUnfortunately, I noticed a typo in the challenge code. "UIController" should be "UIViewController". I tried