Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial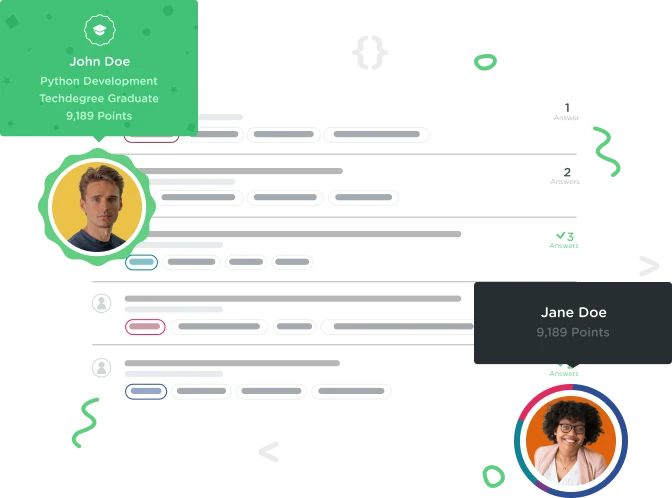

Tijo Thomas
9,737 PointsAnother solution to this problem.
Another way to solve this problem is as follows:
if (event.target.className == "down") {
let li = event.target.parentNode;
let nextLi = li.nextElementSibling;
let ul = li.parentNode;
if (nextLi) {
ul.insertBefore(li, nextLi.nextSibling);
}
}
This combines the insertBefore method and the nextSibling property and is referenced on the Node.insertBefore() page on MDN.
9 Answers
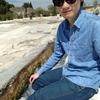
Yiqiu Li
11,619 PointsThats exactly what I did, it seems more intuitive

Justin Hicks
14,290 PointsWhat's awesome is I read the MDN and did this exact thing
if(event.target.className == 'down') {
let li = event.target.parentNode;
let nextLi = li.nextElementSibling;
let ul = li.parentNode;
if(nextLi) {
ul.insertBefore(li, nextLi.nextElementSibling);
}
}

Raymer Ramos
7,683 PointslistUL.addEventListener('click', (e)=>{
if (e.target.tagName.toLowerCase()==='button') {
let li = e.target.parentNode;
let ul = li.parentNode;
if (e.target.className === "remove") {
ul.removeChild(li);
} else if (e.target.className==="up")
{
let prevLi = li.previousElementSibling;
if (prevLi) {
ul.insertBefore(li, prevLi);
}
}else {
let nextLi = li.nextElementSibling;
if (nextLi) {
ul.insertBefore(nextLi, li);
}
}
}
})
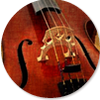
Bethany Cooksey
14,242 PointsI had a lot of fun with this one. :D
if (event.target.className == 'down') {
let li = event.target.parentNode;
let nextNextLi = li.nextElementSibling.nextElementSibling;
let ul = li.parentNode;
ul.insertBefore(li, nextNextLi)
}

pauladams2
8,399 PointsThis is a similar solution that I done
if (event.target.className == 'down') {
let li = event.target.parentNode;
let nextLi = li.nextElementSibling;
let ul = li.parentNode;
if (nextLi) {
let gpNextLi = li.nextElementSibling.nextElementSibling;
ul.insertBefore(li, gpNextLi);
}
}

Stan Clarke Jr.
4,745 PointsI should have looked harder for a solution using insertBefore, but I came across insertAdjacentElement. Took me awhile but I got it to work.
if (event.target.className === "down") {
let li = event.target.parentNode;
let nextLi = li.nextElementSibling;
let ul = li.parentNode;
if (nextLi) {
nextLi.insertAdjacentElement("afterend", li)
}
}
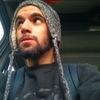
Cosimo Scarpa
14,047 PointsYou need do the same but swap previousElementSibling with nextElementSibling because in this case you need invert the process.
if (event.target.className == 'down') {
let li = event.target.parentNode;
let nextLi = li.nextElementSibling;
let ul = li.parentNode;
if (nextLi) {
ul.insertBefore(nextLi, li)
}
}
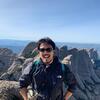
Carlos Chang Cheng
Courses Plus Student 12,030 PointsGreat feedback guys, me too, I think that the most basic solution was this one:
```js
if(e.target.className == 'down'){
let li = event.target.parentNode;
let nextLi = li.nextElementSibling; let ul = li.parentNode; if(nextLi){ ul.insertBefore(li, nextLi.nextSibling); } } ```
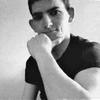
Peter Csakany
3,925 PointsI had similar solution but I guess I complicated it too much.
if (event.target.className === 'down') {
let li = event.target.parentNode;
let nextLi = li.nextElementSibling;
let afterNextLi = nextLi.nextElementSibling;
let ul = li.parentNode;
if (nextLi && afterNextLi) {
ul.insertBefore(li, afterNextLi);
}
else{
ul.appendChild(li)
}
}
nishnash
6,267 Pointsnishnash
6,267 PointsThanks for sharing this. this made a lot more sense to me.. Wish i thoroughly read MDN :)