Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial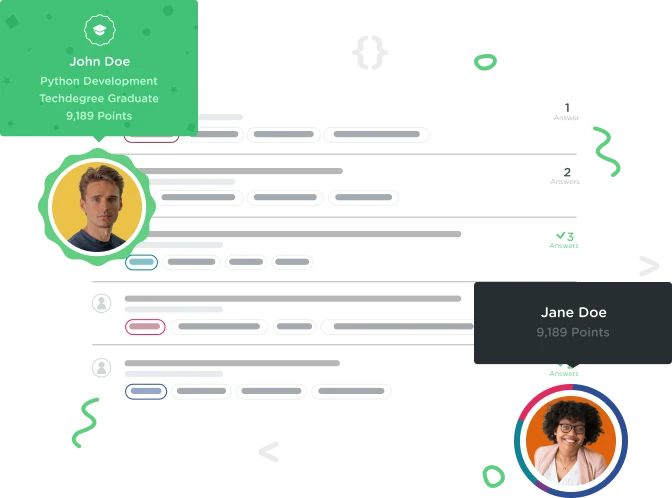

james white
78,399 PointsAnswer analyzer doesn't really seem to be testing rigorously for task 1 of this objective..
Objective (task 1 of 2):
Write a function named is_2012 that accepts a single argument and returns whether that argument's year attribute is equal to 2012.
Here's the link to the Filter objective:
http://teamtreehouse.com/library/functional-python/functional-workhorses/filter-2
So I tried:
def is_2012(year):
if year == 2012:
return True
This gives me:
Bummer! is_2012 returned False for a 2012 datetime.
So I try:
def is_2012(year):
if year == datetime(2012):
return True
Again I get:
Bummer! is_2012 returned False for a 2012 datetime.
Alright...what if I try:
def is_2012(year):
return True
This gives me:
Well done! You're doing great!
That is --I'm doing great at "faking out" the answer for task 1 of the objective..
Not so fast, though!!!!!
The second part of the objective is:
Challenge Task 2 of 2
Now create a variable named dt_2012 that uses filter() and is_2012 to return only the datetimes from dates that are from the year 2012.
Somehow I don't think my "faked out" is_2012 function
from part one is really going to be of much help when attempting to
to frame some sort of working code (in combination with filter() )
to solve for task 2 of the objective.
What do you think?
Maybe the testing for part 1 should have included testing for dates that weren't "2012"
(like "2013", "2011", etc.) to see
if the "is_2012" function provided passes a "false" test in these cases.
Then (and only then) might the "is_2012" function be useful for filtering purposes in part 2 of the objective (is what I'm thinking).
While I was waiting for someone to notice this forum thread I tried
testing dozens and dozens of possible answers for Part 1 and
found this code also seems to work:
def is_2012(dates):
return dates.year == 2012
Maybe that was the intended answer...I don't really know.
How could that be worked into a filter() for task 2 though?
After dozens of tries (and still no answer to this thread),
I finally hit on:
def is_2012(dates):
return dates.year == 2012
Which seemed to solve task 2 of the objective.
So I completed both task 1 and task 2 of the objective,
however, my question still remains:
Should the code below have been excepted as a valid answer to Part1 of the objective?
def is_2012(year):
return True
..because it doesn't do any sort of equality test to return:
whether that argument's year attribute is equal to 2012.
2 Answers

Ken Alger
Treehouse TeacherJames;
Task 1
Write a function named
is_2012
that accepts a single argument and returns whether that argumentβsyear
attribute is equal to 2012.
Your working solution is what I came up with as well. I didn't use dates
as the argument name but if we look at what the task is explicitly asking, it makes sense, right?
def is_2012(argument):
return argument.year == 2012
That is a function with the correct name, accepts a single argument, and returns whether the argument's year
attribute is equal to 2012.
Your question on the code:
def is_2012(year):
return True
I guess depends on what the testing engine is looking for. If the test is passing in something with a year of 2012, then having the function return True
is what would be expected. Perhaps it wasn't tested for 1999.
Not sure that is what you were looking for in terms of an answer, but those are my thoughts.
Happy coding,
Ken
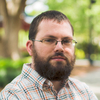
Kenneth Love
Treehouse Guest TeacherYour early first is_2012
solution, just returning True
, was a bug. I fixed it so thanks for that!
Dean McKenzie
11,149 PointsHi Kenneth, first and foremost happy new year. I was wondering if you could explain why the code at the bottom does not work in task 1.
import datetime
dates = [ datetime.datetime(2012, 12, 15), datetime.datetime(1987, 8, 20), datetime.datetime(1965, 2, 28), datetime.datetime(2015, 4, 29), datetime.datetime(2012, 6, 30), ]
def is_2012(args): for i in args: if i.year == 2012: return True
Dean McKenzie
11,149 PointsHi Kenneth, first and foremost happy new year. I was wondering if you could explain why the code at the bottom does not work in task 1.
import datetime
dates = [ datetime.datetime(2012, 12, 15), datetime.datetime(1987, 8, 20), datetime.datetime(1965, 2, 28), datetime.datetime(2015, 4, 29), datetime.datetime(2012, 6, 30), ]
def is_2012(args): for i in args: if i.year == 2012: return True
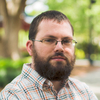
Kenneth Love
Treehouse Guest TeacherTaylor Gresen Your function is expecting an iterable but it's only going to get a single item (check the prompt again). Adjust accordingly.