Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial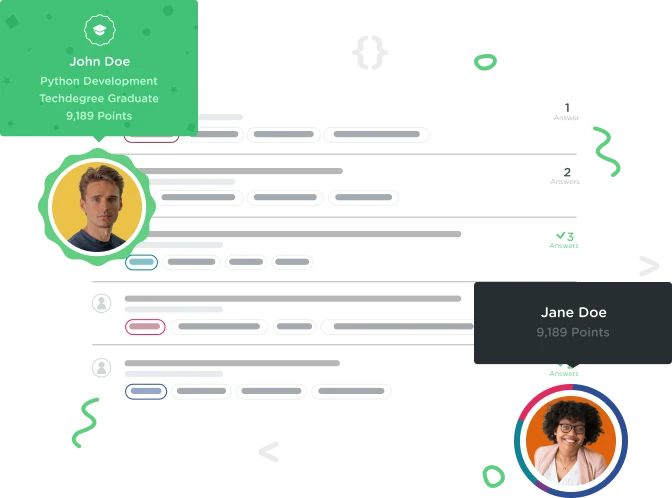

Nicolas Deroin
6,404 PointsAny bad habits I should kick before I move forward?
So here's my code
function print(message) {
document.write(message);
}
let correct = 0;
let incorrect = 0;
let grading = [];
let quiz = [
[ 'How many stripes are there on the US flag?', '13'],
[ 'What shape has three sides?', 'TRIANGLE'],
[ 'What is the flight speed of an unladen swallow?', 'AFRICAN OR EUROPEAN?']
]
for ( i = 0; i < quiz.length; i += 1 ) {
let input = prompt(quiz[i][0])
if (input.toUpperCase() === quiz[i][1]) {
grading.push('1')} else
grading.push('0')
if (input.toUpperCase() === quiz[i][1]) {
correct += 1} else
incorrect += 1
}
print(`You answered ${correct} question(s) correctly, and missed ${incorrect} question(s).`)
print(`<h2>You got these questions right:</h2>`)
for ( i = 0; i < grading.length; i += 1 ) {
if (grading[i] === '1') {
print(`<li> ${quiz[i][0]}</li>`)
} }
print(`<h2>You got these questions wrong:</h2>`)
for ( i = 0; i < grading.length; i += 1 ) {
if (grading[i] === '0') {
print(`<li> ${quiz[i][0]}</li>`)
} }
This code works and outputs exactly as I was expecting, but I also noticed that the way I went about it rarely - if ever - matches with Dave's technique. Are there any particularly bad habits I should kick, or is my version also acceptable?
1 Answer
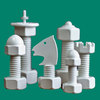
Steven Parker
231,275 PointsInstead of performing the same test twice, you could combine the code blocks into a single test:
if (input.toUpperCase() === quiz[i][1]) {
grading.push("1");
correct += 1;
} else {
grading.push("0");
incorrect += 1;
}
And I wouldn't call it a "bad habit", but it's more "best practice" to declare things things that don't change within the program (like "quiz") by using "const" instead of "let".
Nicolas Deroin
6,404 PointsNicolas Deroin
6,404 PointsGroovy, thanks for the help!