Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial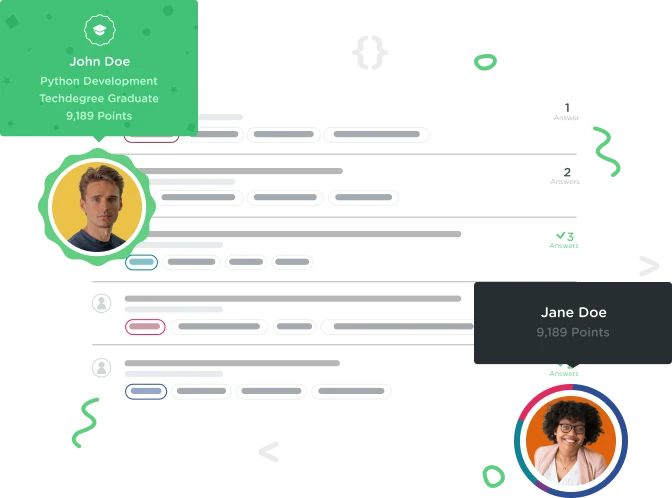

David Gabriel
Python Web Development Techdegree Student 979 PointsAny help please ?
vowels = ("a", "e", "i", "o", "u")
def disemvowel(word): word = list(word) for letter in word: if letter.lower() in vowels: word.remove(letter) return word
vowels = ("a", "e", "i", "o", "u")
def disemvowel(word):
word = list(word)
for letter in word:
if letter.lower() in vowels:
word.remove(letter)
return word
4 Answers
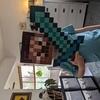
Gabbie Metheny
33,778 PointsYou're getting there! Let's work on your list. Right now you have:
letter = vowels[]
There's two problems here. First, let's rename it to new_word
, since you're also using letter
to represent each letter
in word
and variable confusion is going to break your code. Second, what you're going to need is an empty list, which looks like this:
new_word = []
This is the list you're going to add consonants to, and it will eventually become the word you'll return. One more thing, this variable needs to live inside your function, rather than in the global scope with the vowels tuple. If Kenneth tests more than one word against this function (which he will), letters will keep being added to the same list. For example:
disemvowel('apple)' #returns 'ppl'
disemvowel('cheese') #returns 'pplchs'
Now we can jump into your function.. Since you're appending consonants to a new list, rather than removing vowels from the old word, you need to use not in
, rather than in
. This way, if a letter is in your vowels tuple (so, it's a vowel), you'll disregard it. However, if it's not in vowels
(so, it's a consonant), it will be added to new_word
:
def disemvowel(word):
new_word = []
for letter in word:
if letter.lower() not in vowels:
new_word.append(letter)
Now you're ready to return new_word
, except it's a list, not a string. You can join list items into a string with .join()
, calling it on what you want your joiner to be (in this case, nothing, or an empty string) and passing it what you want joined (in this case, the letters you added to new_word
):
return ''.join(new_word)
Let me know how it goes!
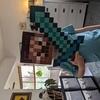
Gabbie Metheny
33,778 PointsA couple things:
- First off, you have some unnecessary indentation on line 1 that is causing some problems. If you remove that, you should start to see more helpful error messages rather than the dreaded "Bummer! Try again!"
- Each time you remove an item from your list in your loop, the indexes change, and so some of your letters will never be checked. For example, if the word was "you," the
y
would start at index0
,o
at index1
andu
at index2
. Your code would remove theo
on the second time through the loop, but would bump theu
down to index1
, the letter that was just checked, so the code wouldn't run a final time to check for thatu
. To get around this, rather than remove letters from the list, you could create an empty list andappend
the letters to the list if they arenot in vowels
. - Currently, you are returning
word
, which is now a list, rather than a string. You'll need tojoin
the letters together before returning them.
Let me know if you need further clarification!

David Gabriel
Python Web Development Techdegree Student 979 PointsHi Gabbie,
Thank you very much, but still no progress on the task vowels = ("a", "e", "i", "o","u") letter = vowels[] def disemvowel(word): for letter in word: if letter.lower() in word: letter.append(vowels) return false return true

David Gabriel
Python Web Development Techdegree Student 979 Pointsvowels = ("a", "e", "i", "o", "u") new_word = list [] def disemvowel(word): for letter in word: if letter.lower() not in vowels: new_word.append(letter) return ''.join(new_word)
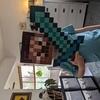
Gabbie Metheny
33,778 PointsYou're so close! Only one change (well, two, but on the same line):
new_word = list []
You don't need to say 'list' before your set of brackets. Simply assigning empty square brackets to the new_word
variable will create an empty list. You also still need to move new_word
inside your function, otherwise each word Kenneth tests will keep getting added to the same list and joined into a single string:
disemvowel('apple') #returns 'ppl'
disemvowel('cheese') #returns 'pplchs'
disemvowel('watermelon') #returns 'pplchswtrmln'
And so on. So you just need to fix that line (removing the word list
, and moving the variable from the global scope to the local scope), and your code should pass!

David Gabriel
Python Web Development Techdegree Student 979 PointsHi Gabby, thanks for you patient and max support; please see
vowels = ("a", "e", "i", "o", "u") def disemvowel(word): new_word = [] for letter in word: if letter.lower() not in vowels: new_word.append(letter) return ' '.join(new_word)
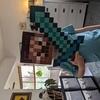
Gabbie Metheny
33,778 PointsLooks like the problem is on your return
statement: the string that you're calling .join()
on should be completely empty, but right now you have a space in there (' ' vs ''). You need the string to be totally empty, otherwise the letters in new_word
will be joined with spaces in between each pair of letters ('ppl' vs 'p p l'). If that doesn't fix it, can you format your code using the Markdown Cheatsheet so I can see how your lines are indented?
David Gabriel
Python Web Development Techdegree Student 979 PointsDavid Gabriel
Python Web Development Techdegree Student 979 PointsHi Gabby, Thank very much for the support, but still not passing, I will keep trying. the explanation is very helpful.