Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial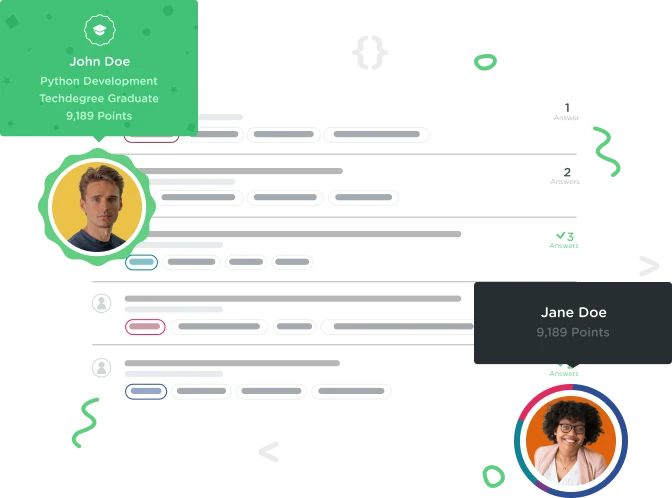

Daniel Horowitz
Courses Plus Student 7,305 PointsAny idea why none of the functionality is working for me yet?
var taskInput = document.getElementById("new-task");
var addButton = document.getElementById("button")[0];
var incompleteTasksHolder = document.getElementById("incomplete-tasks");
var completedTasksHolder = document.getElementById("completed-tasks");
var createNewTaskElement = function(taskString) {
var listItem = document.createElement("li");
var checkBox = document.createElement("input");
var label = document.createElement("label");
var editInput = document.createElement("input");
var editButton = document.createElement("button");
var deleteButton = document.createElement("button");
// Each element needs appending
listItem.appendChild(checkBox):
listItem.appendChild(label):
listItem.appendChild(editInput):
listItem.appendChild(editButton):
listItem.appendChild(deleteButton):
return listItem;
}
// Add new task
var addTask = function() {
console.log("Add task...");
// Create a new list item with the next from #new-task:
var listItem = createNewTaskElement("some New Task");
// Append listItem to incompleteTasksHolder
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
}
// Edit an existing task
var editTask = function() {
console.log("Edit task...");
}
// Delete an existing task
var deleteTask = function() {
console.log("Delete task...");
}
// Mark a task complete
var taskCompleted = function() {
console.log("Task complete...");
var listItem = this.parentNode;
completedTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskIncomplete);
}
// Mark a task incomplete
var taskIncomplete = function() {
console.log("Task incomplete...");
var listItem = this.parentNode;
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
}
var bindTaskEvents = function(taskListItem, checkBoxEventHandler) {
console.log("Bind list item events...");
var checkBox = taskListItem.querySelector("input[type=checkbox]");
var editButton = taskListItem.querySelector("button.edit");
var deleteButton = taskListItem.querySelector("button.delete");
editButton.onclick = editTask;
deleteButton.onclick = deleteTask;
checkBox.onchange = checkBoxEventHandler;
}
addButton.onclick = addTask;
for(var i = 0; i< incompleteTasksHolder.children.length; i++) {
bindTaskEvents(incompleteTasksHolder.children[i], taskCompleted);
}
for(var i = 0; i< completedTasksHolder.children.length; i++) {
bindTaskEvents(completedTasksHolder.children[i], taskCompleted);
}
3 Answers
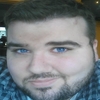
Marcus Parsons
15,719 PointsHey Daniel,
There are a couple things wrong with your code at this point. In your very bottom for loop that binds the task events in the completedTasksHolder, your callback function should be "taskIncomplete" not "taskCompleted".
for(var i = 0; i< completedTasksHolder.children.length; i++) {
bindTaskEvents(completedTasksHolder.children[i], taskIncomplete);
}
Also, you should have your delete function completed by the end of the video.
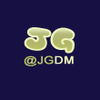
Jonathan Grieve
Treehouse Moderator 91,252 PointsHi Daniel,
It looks like your code is correct up to this point. Are you sure you've correctly linked your JS file to the html page?
Hope this helps :)

Cristian Necsulescu
6,382 PointsHi Daniel, your code has 2 problems . first :
var addButton = document.getElementById("button")[0]; and the correct way is : var addButton = document.getElementsByTagName("button")[0];
and second :
at the appending of each element you've used : instead of ;
// Each element needs appending listItem.appendChild(checkBox): listItem.appendChild(label): listItem.appendChild(editInput): listItem.appendChild(editButton): listItem.appendChild(deleteButton):
// Each element needs appending listItem.appendChild(checkBox); listItem.appendChild(label); listItem.appendChild(editInput); listItem.appendChild(editButton); listItem.appendChild(deleteButton);