Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial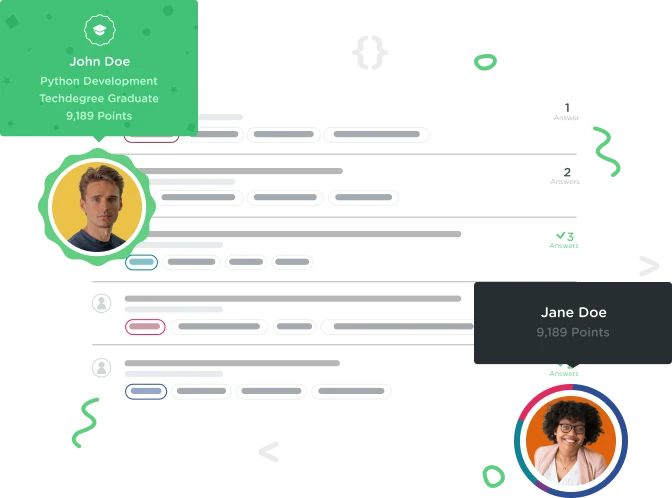
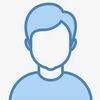
Y. Kravets
10,350 PointsAny ideas what am I doing wrong?
Hi Guys! So I am trying to figure out datetimes and wrote the following script for this objective, but I keep getting: Try again!
Can anyone give me hint of what is it I am doing wrong?
Thanks :)
import datetime
def minutes(time1, time2):
diff = round(((time2 - time1).year * 525949) +
((time2 - time1).month * 43829.1) +
((time2 - time1).day * 1440) +
((time2 - time1).hour * 60) +
(time2 - time1).minute +
((time2 - time1).seconds / 60))
return diff
4 Answers
William Li
Courses Plus Student 26,868 PointsYes, it's actually quite simple.
import datetime
def minutes(dt1, dt2):
return round((dt2 - dt1).seconds / 60)
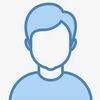
Y. Kravets
10,350 PointsThanks William for a quick response! so basically when I say
something.seconds()
it converts everything in this datetime into seconds? Years, months, days? Everything?
William Li
Courses Plus Student 26,868 Pointsno, of course not, that only works on datetime object, and for this particular code challenge, we can assume that the grader pass in two datetime object to test if your code pass.
takes two datetimes and returns the number of minutes, rounded, between them
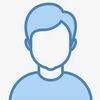
Y. Kravets
10,350 PointsOh, no I think you misunderstood my question. Let me try to phrase it better. So assume we have a datetime object and we call it "something". So this "something" will have the following attributes: year, month, day, hour, minute, second, microsecond.
The way I thought the problem works is that if we have 2 datetimes like that we need to:
- take the difference between the year attributes and convert it to minutes (multiply by 525949),
- then take the difference between month attributes of these 2 datetimes and again convert it to minutes (multiply by 43829.1)
- then take the difference between day attributes of these 2 datetimes and again convert it to minutes (multiply by 1440)
- then take the difference between hour attributes of these 2 datetimes and again convert it to minutes (multiply by 60)
- then take the difference between minutes attributes of these 2 datetimes and do nothing
- then take the difference between seconds attributes of these 2 datetimes and again convert it to minutes (divide by 60)
and at the end to sum it all together so we get the total difference expressed in minutes. You on the other hand simply use something.seconds()
and thats it. So my question is:
Does using .seconds()
converts the entire datetime to seconds? (Year, month, day etc.)
Thank in advance for clarification!
William Li
Courses Plus Student 26,868 PointsOh, I see. If that's what you mean. Then let me explain.
Here's the key point to understand, when you subtract 2 datetime
objects, the result you get back is NOT another datetime object, but a timedelta
object instead.
What timedelta
object is, according Python doc
A duration expressing the difference between two date, time, or datetime instances to microsecond resolution.
and there's a .seconds
attribute in timedelta, that gives you the difference between the two datetime
representing in seconds.
Does that make sense?
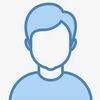
Y. Kravets
10,350 PointsHi William! Yes indeed that makes perfect sense now. My mistake was to think that the difference between two datetime objects is also a datetime object so I treated it as one. But with the correction you explained above I can clearly see why approached it this way! Thanks for taking time to explain this for me! I really appreciate it.
William Li
Courses Plus Student 26,868 Pointsno problem, happy to help.
Adam Oliver
8,214 PointsAdam Oliver
8,214 PointsHi,
Why won't return round((dt2 - dt1).minute) work? It returns an error
William Li
Courses Plus Student 26,868 PointsWilliam Li
Courses Plus Student 26,868 PointsBecause if you take a look at Python doc, there's no minutes property in timedelta object