Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial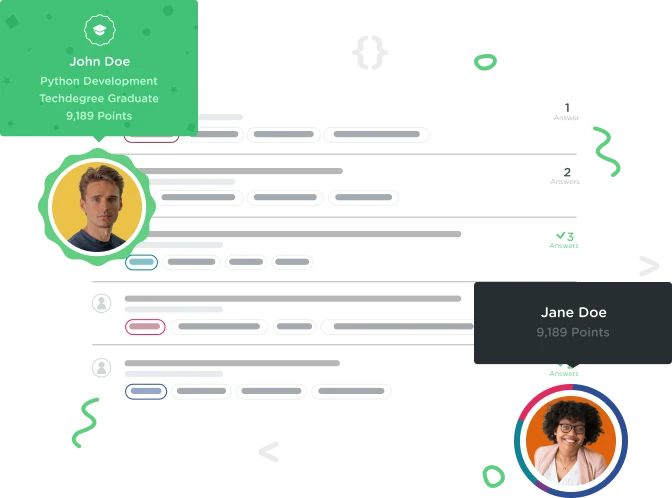
Kevin Lewis
15,088 PointsAny one know how to make a restart button?
The game is done but it would be cool to add a restart button instead of having to press the refresh button. Any one have any ideas on how to implement this?
3 Answers
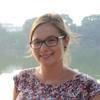
Birthe Vandermeeren
17,146 PointsGreat idea! I've programmed it so that the Start button reappears together with the Game Over message, but with Restart on it:
gameOver(message) {
const gameOverBox = document.getElementById("game-over");
gameOverBox.style.display = "block";
gameOverBox.innerHTML = message;
beginGameButton.style.display = "block";
beginGameButton.textContent = "Restart";
}
Then I've added the function Eric suggested to the beginGameButton event listener like this:
beginGameButton.addEventListener('click', function() {
if ( beginGameButton.textContent.trim() === 'Start' ) {
game.startGame();
this.style.display = 'none';
document.getElementById('play-area').style.opacity = '1';
} else if ( beginGameButton.textContent.trim() === 'Restart' ) {
window.location.reload();
}
});
Like this you will need to click the Start button again though. Does anyone have a better idea?

Eric Butler
33,512 PointsJavaScript has an easy way to trigger a page refresh. It's:
window.location.reload();
So you could add that to a button's click event handler, like so:
// Let's say your HTML has <button class="restart-btn">Restart Game</button>
document.querySelector('.restart-btn').addEventListener('click', function(){
window.location.reload();
return false;
});
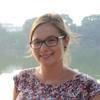
Birthe Vandermeeren
17,146 PointsAt the risk of asking a dumb question: what's the "return false;" part for?

Courtney Wilson
8,031 PointsIf you don't want to press the start button again, I created a restart button and used the same css styling for the start button except I set the display to none (or just use Birthe's idea to rename the start button). I added this listener to reset the game and create a new game:
document.querySelector('#restart').addEventListener('click', e => {
game.reset();
game = new Game(); // instantiate a new game object to reset values
game.startGame();
e.target.style.display = 'none';
});
And added this reset method to Game.js
reset() {
let tokenElements = document.querySelectorAll('.token'); // get all token html elements for removal
tokenElements.forEach(token => {
token.remove();
});
document.querySelector('#game-over').style.display = 'none'; // remove the game over message
}
If you want to keep score using this way, you'd have to do so from app.js since a new game is created with each reset.
Matthew McQuain
14,184 PointsMatthew McQuain
14,184 PointsSorry, I might be a bit dumb. Where do these go? Are they complete replacements for the game over message and 'click' event listeners?