Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial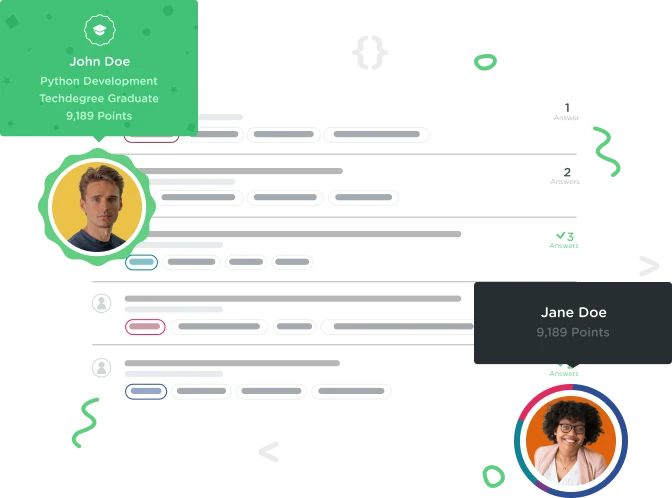

Anthony c
20,907 PointsAny reason to not use constructor to bind functions?
I had some errors coming up, but when I changed the syntax to below, everything worked again:
export default class AddPlayerForm extends Component {
constructor(props){
super(props);
this.onNameChange = this.onNameChange.bind(this);
this.onSubmit = this.onSubmit.bind(this);
this.state = {
name: ''
};
}
static propTypes: {
onAdd: React.PropTypes.func.isRequired,
};
onNameChange(e) {
const name = e.target.value;
this.setState({ name: name });
}
onSubmit(e) {
if (e) e.preventDefault();
this.props.onAdd(this.state.name);
this.setState({ name: '' });
}
render(){
return <div className="add-player-form">
<form onSubmit={this.onSubmit}>
<input
type="text"
value={this.state.name}
onChange={this.onNameChange}
placeholder="Player Name"
/>
<input type="submit" value="Add Player" />
</form>
</div>
}
}
1 Answer

Seth Kroger
56,414 PointsWhile you can manually bind the methods like that, using the property initializer and arrow function syntax Guil uses makes it redundant and a lot cleaner.
Seth Kroger
56,414 PointsSeth Kroger
56,414 PointsIt should be noted that Guil and Beau use a couple of proposed extensions to ES6 in this course like property initializers and object spread syntax. This is why they are using Babel's stage-0 preset.
Anthony c
20,907 PointsAnthony c
20,907 PointsThanks seth. I finally stumbled on this. My current projects outside of this class didn't have the necessary presets (as you pointed out). Good stuff.
No Reply
85 PointsNo Reply
85 PointsI am not sure about your response, as
stage=0
in .babelrc will allow class methods to be used with two colons, in order to avoid binding:<button onClick={::this.onBtnClick} />
In this case
::this.onBtnClick
===this.onBtnClick.bind(this)