Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial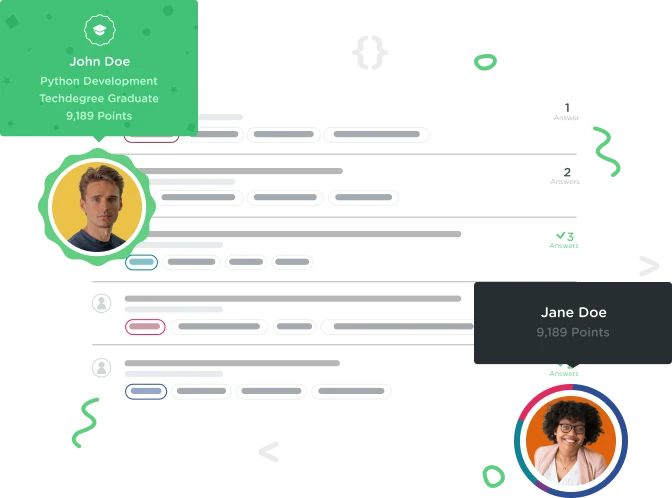

Arsalan Raja
6,508 PointsAny suggestions
var lowNumber = prompt("Enter 1st number?"); var upperNumber = prompt("Enter 2nd number");
var low = parseInt(lowNumber); var upper = parseInt(upperNumber);
function randomNumber( low, upper) {
if(upper > low) { var random = Math.floor(Math.random() * (upper - low + low)) + 1; return random; } else if(low > upper) var random = Math.floor(Math.random() * (low - upper + upper) + 1); return random; }
document.write("Your 1st number was " + low + "and 2nd number was " +upper + "random number is " + randomNumber(low, upper) );
1 Answer
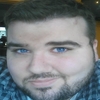
Marcus Parsons
15,719 PointsHi Arsalan,
I would revamp your randomNumber() function a bit. The problem is that each time you run your randomNumber() function, whichever number is the lowest cancels itself out in your generation of the random number. The reason being is that when you have a -number and then + that same number, side by side (with no higher order operations), they will always cancel out.
So, your range is actually [1, max] instead of [min, max]. Test your code out and type in 30 and then 28. You should be getting a number from 28 to 30 but instead you'll get between [1,30].
Here is the method that will work the best and generate a number between [low, upper] (inclusive of low and upper). I'm also going to cut down on the code just a bit by combining things that can be combined. I'll include comments:
/*
included parseInt() around each prompt so that the integers will be stored in lowNumber
and upperNumber directly and forego the need to create two new variables
*/
var lowNumber = parseInt(prompt("Enter 1st number?"));
var upperNumber = parseInt(prompt("Enter 2nd number"));
function randomNumber(low, upper) {
/*
Changed each random generation to this method. This is the best method to
generate numbers in a range.
*/
if (upper > low) {
var random = Math.floor(Math.random() * (upper - low + 1)) + low;
}
//changed else if to just else
else {
var random = Math.floor(Math.random() * (low - upper + 1)) + upper;
}
//put return outside the if-else block because no matter what we're returning random
//and it's best to not repeat yourself as much as possible
return random;
}
//Changed references to low and upper to lowNumber and upperNumber
document.write("Your 1st number was " + lowNumber + " and 2nd number was " + upperNumber + ". Random number is " + randomNumber(lowNumber, upperNumber));
Emily Peregrine
16,848 PointsEmily Peregrine
16,848 PointsWhat are you trying to acheive, would love to help but could you please provide more details