Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial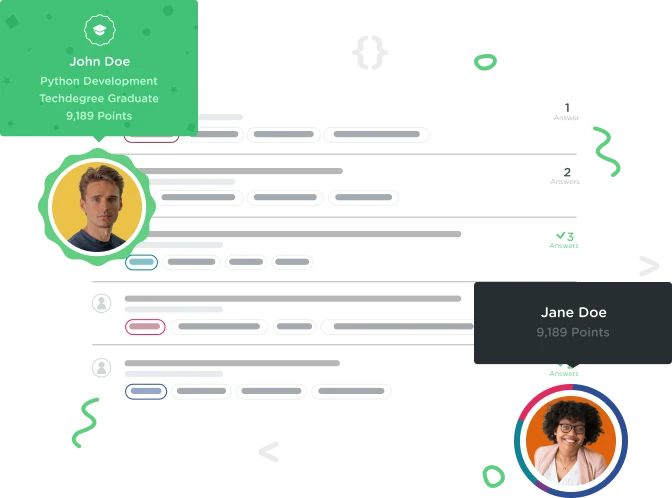
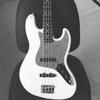
Lee Puckett
12,539 PointsAny tips for this challenge - Updating Entities - appreciated.
I tried to follow the model but obviously I'm getting much of it wrong. Any tips appreciated.
using System.Collections.Generic;
using System.Data.Entity;
using System.Linq;
namespace Treehouse.CodeChallenges
{
public static class Repository
{
public static List<Course> GetCourses()
{
using (var context = new Context())
{
return context.Courses
.OrderBy(c => c.Teacher.LastName)
.ThenBy(c => c.Teacher.FirstName)
.ToList();
}
}
public static List<Course> GetCoursesByTeacher(string lastName)
{
using (var context = new Context())
{
return context.Courses
.Where(c => c.Teacher.LastName == lastName)
.ToList();
}
}
public static Course GetCourse(int id)
{
using (var context = new Context())
{
return context.Courses
.Include(c => c.Teacher)
.SingleOrDefault(c => c.Id == id);
}
}
public static void AddCourse(Course course)
{
using (var context = new Context())
{
context.Courses.Add(course);
context.SaveChanges();
}
}
public static void UpdateCourse(Course course)
{
using (Context context = GetContext())
{
RepositoryToUpdate.SeriesId = repository.SeriesId;
Repository.Entry(repositoryToUpdate).CurrentValues(repository);
context.Repository.Attach(repository);
context.Entry(repository).State = EntityState.Modified;
}
}
}
}
4 Answers
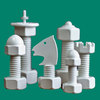
Steven Parker
229,783 PointsI noticed that you mentioned "not knowing this language" ... but while this course may have "Basics" in the title, this is definitely an intermediate-level course. This would not be a good first course for learning C#! If that's your situation, you might set this course aside and do the Beginning C# track first.
Otherwise, if you're still stuck after giving it another try, post your current code for additional assistance.
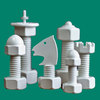
Steven Parker
229,783 PointsWhen you say "follow the model" I'm guessing the "model" is something outside of the challenge? I see references to things like "RepositoryToUpdate", but there's nothing defined by that name in this code. External examples may be helpful as structural models, but it's most unlikely that anything cut from one can be pasted into a challenge and work without adapting to the names used in the challenge.
On the other hand, you could use some of the other code in the challenge as a model. For example, the first instruction of the challenge is "Instantiate an instance of the Context
class within a using
statement". You can see examples of this being done in a consistent way in the other methods of the provided code.
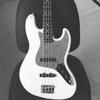
Lee Puckett
12,539 PointsWell that’s my problem. I don’t know what’s applicable and what’s not. I feel like these challenges were done after the videos, instead of writing the challenge first and then designing the video to teach the skills required to complete the challenge. I see a lot of irrelevant skills taught - irrelevant to the challenge - although I’m sure they are relevant to other application - bu those skills/ methods are red herrings for me because not knowing this language, I believe they were introduced for A purpose , because usually educators design a lesson plan so that information introduced is relevant to assessment.
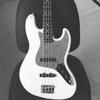
Lee Puckett
12,539 PointsI greatly appreciate your help though. !!!