Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial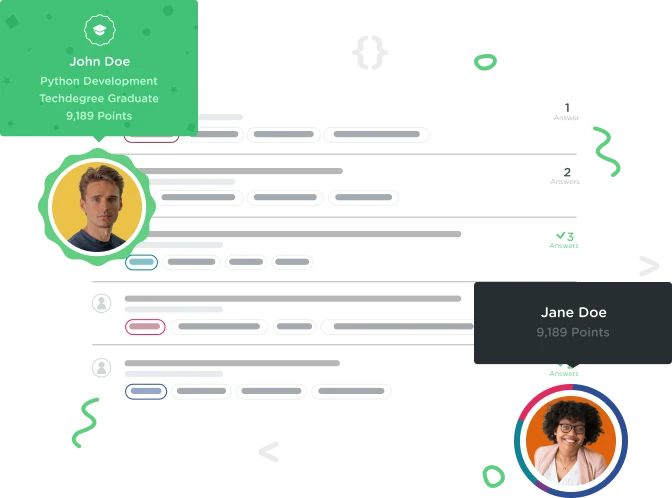

Oscar P
3,690 PointsAny Tips on how to clean this code up?
This is my solution for the List Challenge. I was trying myself to clean up some of the items from the original solution. Wanted some thoughts on being able to make this better. Thanks
soccer_players = []
add_player = input("Its time to select your Soccer team for the inter office showdown. "
"Would you like to add a player to the list? (Yes/No) ")
try:
if add_player != "yes":
raise ValueError()
except ValueError:
print("Ok, Maybe next time")
while add_player == "yes":
soccer_players.append(input("Enter the name of the player you would like to add: "))
add_player = input("Would you like to add another player to the list? (Yes/No) ")
if add_player == "yes":
continue
elif add_player == "no":
print("\nThere are {} players on the team".format(len(soccer_players)))
else:
sys.exit()
x = 1
for i in soccer_players:
print("Player {}: {}".format(x, i))
x += 1
if soccer_players:
goal_keeper = int(input("Please select the goal keeper by selecting "
"the player number 1 -{}: ".format(len(soccer_players))))
print("Great the goalkeeper for the game will be {}.".format(soccer_players[goal_keeper - 1]))
1 Answer
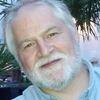
Jeff Muday
Treehouse Moderator 28,716 PointsI like where you are going with this-- you have the beginnings of a league simulator!
It's great to share your code and get feedback. One of the things I love about programming is there are MANY correct ways to write a program and express your style.
Things that are great about your program--
- very clearly written and easy to follow
- well-named variables
- good program flow
I don't know if I would even want to change your program. It works and is pretty robust.
Just in case you are considering refactoring:
- Adding a specific "Yes/No/Quit" function will remove some repeated code! And, you will probably use it again and again when you add more features to your program.
- I would have them enter a BLANK or empty name to finalize the list. It will seem less repetitive to users.
- A cool trick is to use Python
enumerate()
. Look at the last loop in the program.
def yes_no_quit(prompt):
import sys # just in case it was not imported at the beginning
while True:
response = input("{} (Yes/No/Quit) > ".format(prompt))
if response.upper().startswith("Y"):
return True
if response.upper().startswith("N"):
return False
if response.upper().startswith("Q"):
print("Thank you-- terminating program.")
sys.exit(0)
print("Please answer with a Yes, No, or Quit")
soccer_players = []
print("It's time to select your Soccer team for the inter-office showdown.")
add_players = yes_no_quit("Would you like to add players?")
while add_players:
name = input("Enter the name of the player to add (blank for done): ")
if name:
soccer_players.append(name)
else:
# user entered a blank, done adding players
add_players = False
print("\nThere are {} players on the team".format(len(soccer_players)))
for idx, name in enumerate(soccer_players):
print("Player {}: {}".format(idx+1, name))
if soccer_players:
goal_keeper = int(input("Please select the goal keeper by selecting "
"the player number 1 - {}: ".format(len(soccer_players))))
print("Great, the goalkeeper for the game will be {}.".format(soccer_players[goal_keeper - 1]))
Oscar P
3,690 PointsOscar P
3,690 PointsThank you Jeff for your input, thats exactly the kind of response I was looking for, it helps.