Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial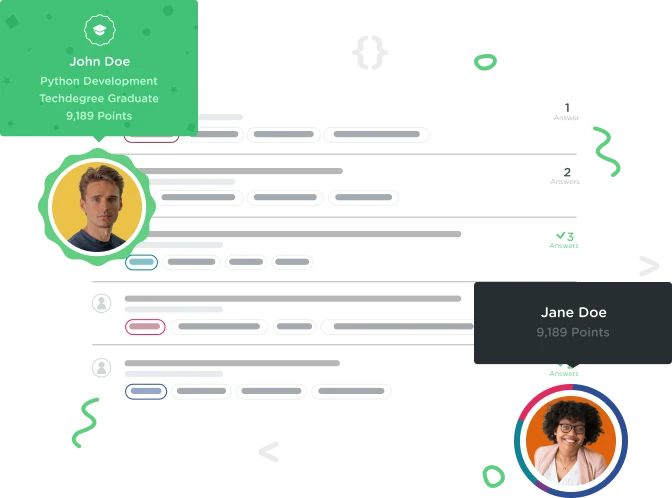
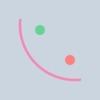
Martin Wiulsrød
Front End Web Development Techdegree Graduate 16,314 PointsAny tips or pointers?
The amount of "AHA!" moments I had during this coding challenge was insane, what a great challenge! Previously in this tech-degree, I have posted my code and asked what improvements that can be done. I have gotten some really great responses which have helped me become a better programmer. I'm open to any criticism or pointers! Here is my code:
// define variables
var html = "";
var students = [
{name : "Martin", track : "JavaScript", achievements : 5, points : 1005},
{name : "Alex", track : "Java", achievements : 2, points : 25},
{name : "Jonas", track : "C#", achievements : 10, points : 66},
{name : "Hannah", track : "Python", achievements : 3, points : 22},
{name : "Maria", track : "CSS", achievements : 2, points : 16784},
];
// display objects from an array
function displayStudents (arr) {
for (var i = 0; i < arr.length; i++) {
for (var property in arr[i]) {
if (property === "name") {
html += "<h2>" + capitalizeFirstLetter(property) + ": " + arr[i][property] + "</h2>";
} else {
html += capitalizeFirstLetter(property) + ": " + arr[i][property] + "<br>";
}
}
html += "<br>";
}
return html;
}
// print out message
function print(message) {
document.getElementById("output").innerHTML = message;
}
// capitalize first letter of a string
function capitalizeFirstLetter(string) {
return string.charAt(0).toUpperCase() + string.slice(1);
}
// call functions
print(displayStudents(students));
1 Answer
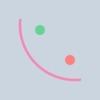
Martin Wiulsrød
Front End Web Development Techdegree Graduate 16,314 PointsThanks for your response 🍹 Dave 🌴 StSomeWhere! Definitely gonna clean up my comments and start using let and const forward. I totally agree with the refactoring of that overly complicated function, I'm gonna chew on that when I go to bed (but listen to the guy in Cannonball Run). I have not read a lot of other peoples code, and I'm not used to documenting it very well from before. I need to create some rules and do some more research on how to name and document my code in a better way.
Thank you very much for taking the time though!
Dave StSomeWhere
19,870 PointsDave StSomeWhere
19,870 PointsHere's what pops out to me (just my silliness of course):
var
and start usinglet
andconst
.displayStudents
by trying to avoid nesting twofor
statements and then anif
and then anelse
- anything deeper that 2 levels causes me to do a special review.displayStudents()
. So, it is really just a Student array function. Calling the parameterarr
also doesn't help, since it is really the students array - why not call itstudentsArray
.Have fun and be glad you understand how to loop an array and process indexes and your style will continue to develop.
Quit worrying so much, your code is fine - nicely done