Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial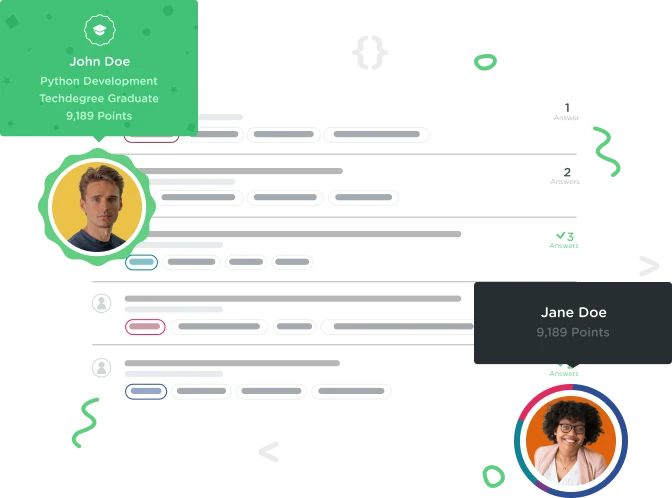
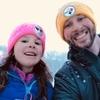
Dale Sublett
14,231 PointsAny videos specifically tackling how to validate multiple checkboxes in an HTML form using only JavaScript??
I MUST validate a HTML form using plain JavaScript. (not jQuery)
I have my form built, and most of my fields are validating like I expect. But I am having a heck of a time figuring out how to validate my checkboxes. I have multiple checkboxes and I must validate that at least 1 of them has been check before allowing the form to submit.
Any help is appreciated.
9 Answers

Eric Butler
33,512 PointsHey Dale, The code works in a general sense, here's a proof-of-concept Codepen to play with: https://codepen.io/ericbutler555/pen/zyMXdm?editors=1011
Without seeing your HTML and JS I can't know how to modify this for your particular setup, and I'm hoping to help you figure out how you can solve it yourself by providing an example of how it works in general. But if you're really at a loss, post some more code and maybe I can figure out how to make this work for you.
Cheers!
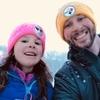
Dale Sublett
14,231 PointsThis is my JS code: At the bottom is where I was wanting to plug my checkbox validation in.
//form validation function function validate() { //function to check if a name has been entered var name = document.getElementById('name').value;
if (name == null || name == "") {
document.getElementById('nameerror').innerHTML = "Please enter your full name: ";
}
//function to check if an email has been entered
var email = document.getElementById('email').value;
if (email == null || email == "") {
document.getElementById('emailerror').innerHTML = "Please enter your email address: ";
}
//function to check if a telephone number has been provided
var phone = document.getElementById('phone').value;
if (phone == null || phone == "") {
document.getElementById('phoneerror').innerHTML = "Please enter your telephone number: ";
}
//function to validate a radio button has been selected
var radios = document.getElementsByName("radio");
var formValid = false;
var i = 0;
while (!formValid && i < radios.length) {
if (radios[i].checked) formValid = true;
i++;
}
if (!formValid) {
document.getElementById('radioerror').innerHTML = "(Please check one)";
return formValid;
}
//function to confirm if checkbox has been checked
}

Eric Butler
33,512 PointsHi again Dale, Thanks for posting all this. I have a solution for you. I rewrote your validate.js file a little:
function validate() {
// NEW: move this way up here so all validations can affect its value:
var formValid = true;
// function to check if a name has been entered
var name = document.getElementById('name').value;
if (name == null || name.trim() == "") {
document.getElementById('nameerror').innerHTML = "Please enter your full name";
formValid = false;
} else {
document.getElementById('nameerror').innerHTML = "";
}
// function to check if an email has been entered
var email = document.getElementById('email').value;
if (email == null || email.trim() == "") {
document.getElementById('emailerror').innerHTML = "Please enter your email address";
formValid = false;
} else {
document.getElementById('emailerror').innerHTML = "";
}
// function to check if a telephone number has been provided
var phone = document.getElementById('phone').value;
if (phone == null || phone.trim() == "") {
document.getElementById('phoneerror').innerHTML = "Please enter your telephone number";
formValid = false;
} else {
document.getElementById('phoneerror').innerHTML = "";
}
// function to validate if any radio button has been selected
var radios = document.getElementsByName('radio');
var radiosValid = false;
var i = 0;
while (!radiosValid && i < radios.length) {
if (radios[i].checked) radiosValid = true;
i++;
}
if (!radiosValid) {
document.getElementById('radioerror').innerHTML = "(Please check one)";
formValid = false;
} else {
document.getElementById('radioerror').innerHTML = "";
}
// function to confirm if any checkbox has been checked
var checkboxes = document.getElementsByName('checkbox');
var checkboxesValid = false;
var j = 0;
while (!checkboxesValid && j < checkboxes.length) {
if (checkboxes[j].checked) checkboxesValid = true;
j++;
}
if (!checkboxesValid) {
document.getElementById('checkboxerror').innerHTML = "(Please select at least one)";
formValid = false;
} else {
document.getElementById('checkboxerror').innerHTML = "";
}
// now that all validations have run, return the conclusion
return formValid;
}
Here's a breakdown:
Your formValid
variable should represent all validations, not just the radios. If we set it to true
at the start of the file, then as we run through each validation, if it fails, we change formValid
to false, which will ultimately be what is returned by the function (because we return formValid
at the end of the file), and returning false will prevent the form from submitting. If formValid
stays true and thus validate()
returns true, the form will submit.
You want to .trim()
the values before checking if they're ""
, otherwise a person can just enter spaces and it validates true.
You want to remove the error messages once the field validates (by setting `.innerHTML=""). If a user tries to submit the form and gets 2 errors, the fixes 1 and tries to submit the form again, you want the 1 fixed error to go away while the other one still prevents the form from submitting.
The way to do the checkbox-check can be done basically the same way your radio-check was done. You set a variable that assumes no option is checked, then loop through and as soon as one is found to be checked, the field is validated. What I changed was basically just letting them be their own validation, that once finished, let that factor into the whole form validation via the formValid
variable.
Does all that make sense? Hope this gets you out of the fog, and good luck with everything!

Eric Butler
33,512 PointsHey Dale, Sounds like you have a pretty good grasp of JS, so here's a short and sweet solution to get you over the hump (change things to increase specificity for your particular form/html):
document.querySelector('form').addEventListener('submit', function(e){
e.preventDefault();
var boxesChecked = document.querySelectorAll('[type="checkbox"]:checked').length;
if (boxesChecked > 0) {
// this.submit();
console.log('form can be submitted');
} else {
console.log('please check at least 1 checkbox');
}
});
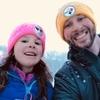
Dale Sublett
14,231 PointsHey Eric, thanks for the reply. I tried plugging that code in with the necessary modifications, but it does nothing. Nothing logs to the console. The rest of my form validations work.
I have a function that all of my code is inside of, called Validate(). I have that attached to my HTML with a onsubmit="return validate()"
Do I need to remove the addEventListener('submit', function(e){}
Also, I am not the greatest at debugging, but I put a breakpoint in, and then once I stepped over it, my page reloaded and I ended up with a jquery.min file instead of my JS.
I think I remember watching a debugger video and it used a "blackbox" to put all of the extra files in so just my JS and HMTL files run.
Any help with any of that??
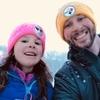
Dale Sublett
14,231 PointsI will post my code below this. I have spent two whole days watching videos, reading docs, and searching StackOverflow. I have came up with many solutions, but i can't figure out what it is that I am missing, or overlooking, or just flat out not doing. I was hoping to solve this myself, but I am at a loss now lol
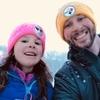
Dale Sublett
14,231 PointsSome of my requirements require inline things and other scripting within script tags. Don't mind those. But this is my HTML that contains my checkboxes. I put some extra spaces in where my Form starts, and also where my checkboxes start as well.
<!DOCTYPE html>
<html lang="en" xmlns="http://www.w3.org/1999/xhtml"> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no"> <title>Contact Us</title> <style>
.contact-header {
font-family: cursive;
text-align: center;
font-size: 50px;
color: darkred;
}
form {
font-weight: bold;
text-align: center;
}
.contact {
font-weight: normal;
}
.checkbox input {
margin: 0 10px 0;
}
textarea {
width: 20%;
height: 5rem;
}
.sendRequest {
text-align: center;
}
</style>
<!--link to bootstrap css-->
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css" integrity="sha384-Gn5384xqQ1aoWXA+058RXPxPg6fy4IWvTNh0E263XmFcJlSAwiGgFAW/dAiS6JXm" crossorigin="anonymous">
<!--link for icons-->
<link rel="stylesheet" href="https://use.fontawesome.com/releases/v5.6.3/css/all.css" integrity="sha384-UHRtZLI+pbxtHCWp1t77Bi1L4ZtiqrqD80Kn4Z8NTSRyMA2Fd33n5dQ8lWUE00s/" crossorigin="anonymous">
<!--link to external stylesheet-->
<link href="restaurantStyles.css" rel="stylesheet" type="text/css" />
</head> <body> <div class="container"> <header> <div class="jumbotron name-font"> <h1 class="display-4">Dan's Cakes</h1> <hr class="my-4"> <p class="lead">BIG NEWS!! Dan's Cakes will be opening a new restaurant VERY soon!!!!</p>
</div>
</header>
<hr />
<nav>
<!--home icon link-->
<a href="index.html" class="btn badge-pill"><i class="fas fa-home"></i></a>
<a href="menu.html" class="btn badge-pill">Menu</a>
<a href="contact.html" class="btn badge-pill">Contact Us</a>
</nav>
<hr />
<h2 class="contact-header">Contact Us</h2>
<hr />
<!--form for contact info-->
<form name="contactForm" method="post" id="contactForm" novalidate onsubmit="return validate()">
<div class="form-group col-form-label">
<label for="name">Name: </label>
<input type="text" class="form-control" id="name" placeholder="Please enter your full name.." required>
<span id="nameerror" class="hint"></span>
</div>
<div class="form-group">
<label for="email">Email: </label>
<i class="fas fa-envelope prefix"></i>
<input type="email" class="form-control" id="email" placeholder="Please enter your email address.." aria-describedby="email" required>
<span id="emailerror" class="hint"></span>
<small id="emailHelp" class="form-text text-muted">We'll never share your email with anyone else.</small>
</div>
<div class="form-group">
<label for="phone">Phone: </label>
<i class="fas fa-phone-square"></i>
<input type="tel" class="form-control" id="phone" required>
<span id="phoneerror" class="hint"></span>
</div>
<!--select menu-->
<label for="reason-select">Reason For Inquiry:</label>
<select id="select " name="reason" class="custom-select" required>
<option value="0">--Please Choose an Option--</option>
<option value="1">Catering</option>
<option value="2">Private Party</option>
<option value="3">Feedback</option>
<option value="4">Other</option>
</select>
<span id="selecterror" class="hint"></span>
<br />
<br />
<!--text area for additional info-->
<div class="form-group">
<label for="info">Additional Information: </label>
<textarea class="form-control" id="info" rows="5"></textarea>
<span id="textareaerror" class="hint"></span>
</div>
<!--radio buttons for visiting restaurant-->
<label for="radio">Have you been to the restaurant?</label>
<div class="form-check form-check-inline">
<input class="form-check-input" type="radio" name="radio" id="no-radio" value="no">
<label class="form-check-label" for="no-radio">
No
</label>
</div>
<div class="form-check form-check-inline">
<input class="form-check-input" type="radio" name="radio" id="yes-radio" value="yes">
<label class="form-check-label" for="yes-radio">
Yes
</label>
<span id="radioerror" class="hint"></span>
</div>
<br />
<!--checkboxes for contacting-->
<label for="checkboxes">Best days to contact you:</label>
<div id="checkboxlist">
<div class="form-check form-check-inline">
<input class="form-check-input" name="checkbox" type="checkbox" id="monday" value="monday">
<label class="form-check-label" for="monday">M</label>
</div>
<div class="form-check form-check-inline">
<input class="form-check-input" name="checkbox" type="checkbox" id="tuesday" value="tuesday">
<label class="form-check-label" for="tuesday">T</label>
</div>
<div class="form-check form-check-inline">
<input class="form-check-input" name="checkbox" type="checkbox" id="wednesday" value="wednesday">
<label class="form-check-label" for="wednesday">W</label>
</div>
<div class="form-check form-check-inline">
<input class="form-check-input" name="checkbox" type="checkbox" id="thursday" value="thursday">
<label class="form-check-label" for="thursday">Th</label>
</div>
<div class="form-check form-check-inline">
<input class="form-check-input" name="checkbox" type="checkbox" id="friday" value="friday">
<label class="form-check-label" for="friday">F</label>
</div>
<span id="checkboxerror" class="hint"></span>
</div>
<!--send request button-->
<div class="sendRequest" id="contact-submit">
<input type="submit" value="Send Request">
</div>
</form>
<br />
<br />
<footer>
<p>1123 Silk Way, Anchorage, AK, 99501</p>
<p>907-998-0122</p>
</footer>
</div>
<script>
document.contactForm.name.onfocus = function () {
document.getElementById('namehint').innerHTML = "(Enter full name)";
}
</script>
<!--scripts for jquery, popper, and bootstrap-->
<script src="https://code.jquery.com/jquery-3.3.1.slim.min.js" integrity="sha384-q8i/X+965DzO0rT7abK41JStQIAqVgRVzpbzo5smXKp4YfRvH+8abtTE1Pi6jizo" crossorigin="anonymous"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.14.6/umd/popper.min.js" integrity="sha384-wHAiFfRlMFy6i5SRaxvfOCifBUQy1xHdJ/yoi7FRNXMRBu5WHdZYu1hA6ZOblgut" crossorigin="anonymous"></script>
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.2.1/js/bootstrap.min.js" integrity="sha384-B0UglyR+jN6CkvvICOB2joaf5I4l3gm9GU6Hc1og6Ls7i6U/mkkaduKaBhlAXv9k" crossorigin="anonymous"></script>
<!--javascript link to external sheet-->
<script src="validate.js"></script>
<!--<script>
document.contactForm.name.onfocus = function () {
document.getElementById('namehint').innerHTML = "(Enter full name)";
}
</script>
-->
</body> </html>

Eric Butler
33,512 PointsOne note here: You insisted in your question not to use jQuery, yet you are loading in the jQuery library (because it's a prerequisite for Bootstrap). Not sure if you didn't realize this or if this is like a school assignment with a coding restriction, but figured I should point it out since it's silly to load a big library into a site and not use it.
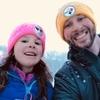
Dale Sublett
14,231 PointsThank you very much for the code, but even more so for the breakdown of everything. Understanding what I was doing wrong is the most valuable piece of information. I really hope that you're a developer currently, because this problem had me stumped and you just took my code and fixed it. Thank you again!!
On a side note, we aren't allowed to use jQuery to validate the form, just plain JS. We were permitted to use Bootstrap for the responsiveness and other small things.
But again, thank you very much for all of your help!! I hope to see a video you make for form validation one day lol

Eric Butler
33,512 PointsAwesome! So glad to hear it, Dale, and I hope everything is making more sense to you. Yes, I've been employed as a front-end developer for a handful of years, and I still get stumped/blocked myself frequently and have to browse Stack Overflow/MDN/W3 Schools and all the rest to help me figure things out. It can be frustrating but finding a solution and learning is what makes coding so fun! Best of luck to you.
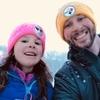
Dale Sublett
14,231 PointsHey Eric,
I have another question. So I now need to provide a success message once the user has submitted a valid form. I have tried a few different combinations of IF statements, but I am not having any success(pun intended)
Is an If statement the correct way to do this? Do I need to add this at the bottom of my code? Inside of the same set of curly braces? Or make a new function?
Thank you