Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial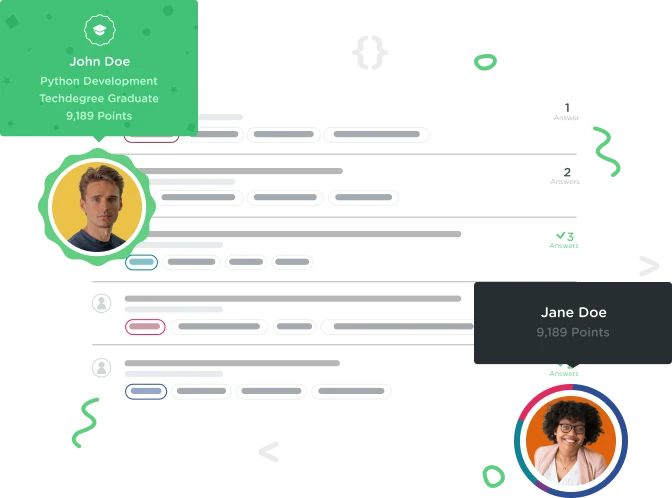

Andrew Sherman
6,799 PointsAnyone have any tips on getting this code Challenge to pass?
I feel like I've tried a multitude of different things but the instructions are (like most of the code challenges) unclear and confusing. You'd think for people trying to learn they wouldn't set you up with challenges featuring things the videos have only skimmed over...
struct Location {
let latitude: Double
let longitude: Double
}
1 Answer
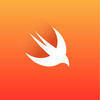
Steven Deutsch
21,046 PointsHey Andrew Sherman,
This challenge wants you to create a Business class. This class will have two constant stored properties. The first will be called name and be of type String. The second will be called location and be of type Location. This Location type is the Location struct that the challenge has provided you with.
After you set up the skeleton of the Business class and its properties, you need to create an initializer for these properties. Remember that the purpose of an initializer is to make sure that an object is ready for use at runtime. An object being ready for use means that its properties have values.
The initializer will take two arguments, one for each of the properties of the class you are initializing. These arguments will match the properties types - the first is a String, the second is a Location. Inside the body of the initializer, you will assign these values being passed in to the properties of the Business class. We have to use the keyword self here to refer to the property of the Business class because the names of the initializer parameters share the same names. If you used different names, however, self would not be required.
Finally, all thats left is to call the Business initializer and assign the result to a constant called someBusiness. When calling the initializer, you need to pass in the types of values that you defined in its implementation. The first argument will be a String, the second argument will be a Location.
In order to create the Location instance, you can call through to the Location initializer. You will see that you need to pass the Location initializer two arguments in order to create an instance. The first is a Double and the second is a Double.
The result of the Location initializer call will be passed in as the second argument for the Business initializer call.
struct Location {
let latitude: Double
let longitude: Double
}
class Business {
let name: String
let location: Location
init(name: String, location: Location) {
self.name = name
self.location = location
}
}
let someBusiness = Business(name: "Business Name", location: Location(latitude: 20.0, longitude: 45.0))
Good Luck!