Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial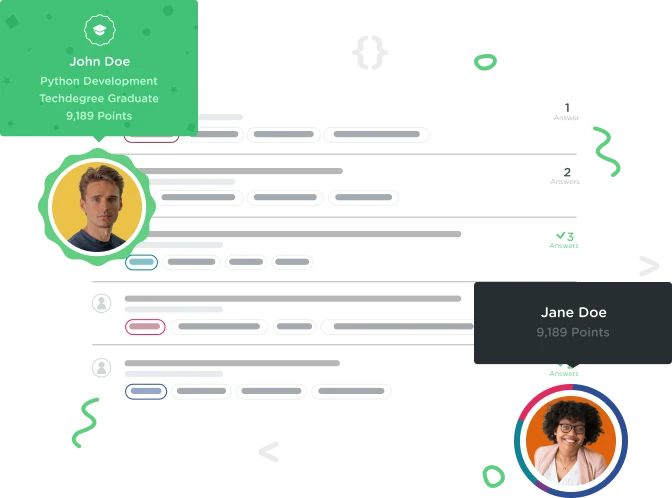

Jackson Monk
4,527 PointsAnyone know why setInterval() only runs once?
Trying to create a slow gravity effect on a CSS circle with JS. However, the circle only moves once instead of every 500 milliseconds like I tell it to in setInterval(). Why is this?
<html>
<head>
<style>
.circle {
position: relative;
padding: 15px;
background-color: black;
border-radius: 50%;
width: 0.2%;
}
</style>
</head>
<body>
<div class = circle></div>
<script>
let circle = document.getElementsByTagName('div')[0];
function fall(rate) {
circle.style.top += rate;
}
function execute() {
fall(2);
}
setInterval(execute, 500);
</script>
</body>
</html>
1 Answer
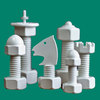
Steven Parker
230,274 PointsThe interval is running, but you don't see any effect because the property value is a string and you need to convert it to a number to do math with it:
function fall(rate) {
var top = parseInt(circle.style.top || 0) + rate;
circle.style.top = top + "px";
}
Jackson Monk
4,527 PointsJackson Monk
4,527 PointsI thought I tried that, but I thought it was weird because it ran once, and if it wasn't working it wouldn't run at all. I'll try this though
Jackson Monk
4,527 PointsJackson Monk
4,527 PointsWow! Works perfectly! Why is it that when I tried it with
parseInt(circle.style.top)
, it didn't work, but when I didparseInt(circle.style.top || 0)
, it worked perfectly?Steven Parker
230,274 PointsSteven Parker
230,274 PointsThat's because until you assign it, that property returns only an empty string. But the "
|| 0
" will substitute a zero when the string is empty.Happy coding!