Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial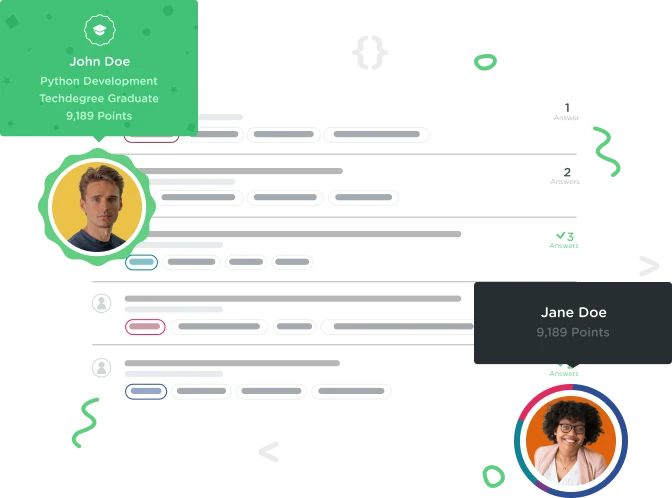

Christopher Bryant
220 PointsAnyone know why this won't work?
Any help appreciated. Thank you!
var request = new XMLHttpRequest();
request.onreadystatechange = function () {
if (request.readyState === 4) {
document.getElementById("footer").innerHTML = request.responseText;
}
};
request.open("GET", "footer.html");
function sendAJAX() {
request.send();
}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>AJAX with JavaScript</title>
<script src="app.js"></script>
</head>
<body>
<div id="main">
<h1>AJAX!</h1>
</div>
<div id="footer"></div>
</body>
</html>
1 Answer
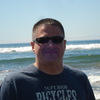
Mark Ihrig
19,966 PointsThe last part of your code looks like this:
request.open("GET", "footer.html");
function sendAJAX() {
request.send();
}
For the quiz, your request.send(); is correct, and is the only thing that is needed after the request.open(). Try removing the function sendAJAX(){} portion. The variable called "request" is already defined at the beginning of the code, so your last part of the code should be the following.
request.open("GET", "footer.html");
request.send();
A X
12,842 PointsA X
12,842 Points@Mark Ihrig: I appreciate you explaining what was wrong more thoroughly than Dave's video did. I didn't understand the majority of the code he wrote because he didn't explain what he was using it for. So I didn't understand what
function sendAJAX() { request.send();}
meant or why it would be needed or not, so I included it just like Christopher did.