Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial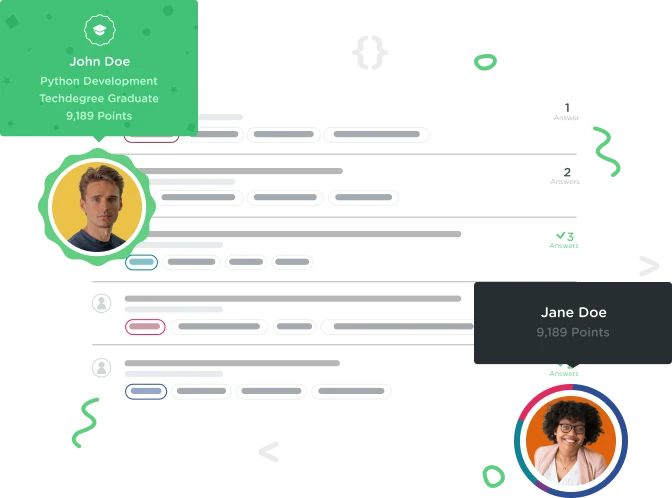
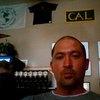
Justin Noor
3,692 PointsAnyone out there working on the build_a_soccer_league project?
I've been stuck on this for a few. Anyone out there want to compare logic? Did you use a for loop or a while loop? I couldn't get either to work.
The only thing I could come up with was to create a list of dicts, and then split it into two camps using a list comprehension. From there, reassign the players into teams. The problem is that this method does not use any logic as the instructions demand. Furthermore, it uses methods that we did not cover. Any feedback or hints would be great. This is what I came up with:
league = [
{'name': 'Smith, Joe', 'height': 42, 'experience': 'y', 'gaurdian name(s)': 'Jan and Jim Smith'},
{'name': 'Tanner, Jill', 'height': 36, 'experience': 'y', 'gaurdian name(s)': 'Clara Tanner'},
{'name': 'Bon, Bill', 'height': 43, 'experience': 'y', 'gaurdian name(s)': 'Sara and Jenny Bon'},
{'name': 'Gordon, Eva', 'height': 45, 'experience': 'n', 'gaurdian name(s)': 'Wendy and Mike Gordon'},
{'name': 'Gill, Bill', 'height': 40, 'experience': 'n', 'gaurdian name(s)': 'Charles and Sulvia Gill'},
{'name': 'Stein, Kimmy', 'height': 41, 'experience': 'n', 'gaurdian name(s)': 'Bill and Hillary'},
{'name': 'Adams, Sammy', 'height': 45, 'experience': 'n', 'gaurdian name(s)': 'Jeff Adams'},
{'name': 'Saygan, Karl', 'height': 42, 'experience': 'y', 'gaurdian name(s)': 'Heather Bledsoe'},
{'name': 'Greenberg, Suzane', 'height': 44, 'experience': 'y', 'gaurdian name(s)': 'Henrietta Dumas'},
{'name': 'Dali, Sal', 'height': 41, 'experience': 'n', 'gaurdian name(s)': 'Gala Dali'},
{'name': 'Kavalier, Joe', 'height': 39, 'experience': 'n', 'gaurdian name(s)': 'Sam and Elaine Kavalier'},
{'name': 'Finkelstein, Ben', 'height': 44, 'experience': 'n', 'gaurdian name(s)': 'Aaron and Jill Finkelstein'},
{'name': 'Soto, Diego', 'height': 41, 'experience': 'y', 'gaurdian name(s)': 'Robin and Sarik Soto'},
{'name': 'Alaska, Chloe', 'height': 47, 'experience': 'n', 'gaurdian name(s)': 'David and Jamie Alaska'},
{'name': 'Willis, Arnold', 'height': 43, 'experience': 'n', 'gaurdian name(s)': 'Claire Willis'},
{'name': 'Helm, Phillip', 'height': 44, 'experience': 'y', 'gaurdian name(s)': 'Thomas and Eva Jones'},
{'name': 'Clay, Les', 'height': 42, 'experience': 'y', 'gaurdian name(s)': 'Wynonna Brown'},
{'name': 'Krustofski, Herschel', 'height': 45, 'experience': 'y', 'gaurdian name(s)': 'Hyman and Rachel Krustofski'}
]
#split league into two lists according per experience or inexperience
experienced = [player for player in league if 'y' == player['experience']]
inexperienced = [player for player in league if 'n' == player['experience']]
#create new teams with even numbers of experienced and inexperienced players
Dragons, Sharks, Raptors = map(list, zip(experienced, inexperienced))
2 Answers
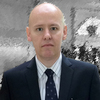
Nathan Tallack
22,160 PointsWhat you could do is iterate through your list to separate your experienced players out, so you have two lists, one with experienced players and one without.
Next sort your lists so that you have them ordered from tallest to smallest.
Finally, in order, take three from the top of each list and assign one to each of the three teams. Randomize that each time so you are assigning to the teams fairly. Do this until your lists are done.
You should have ended up with three teams having an equal number of experienced players and inexperienced players, and they should have their height fairly distributed.
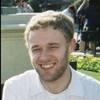
Adam Cameron
Python Web Development Techdegree Graduate 16,731 PointsI've figured out how to randomize which team the experienced player is assigned to, but I can't figure out how to make Python keep the assignments to no more than three per list. I've tried while and if statements and nothing; any idea how to do this?