Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial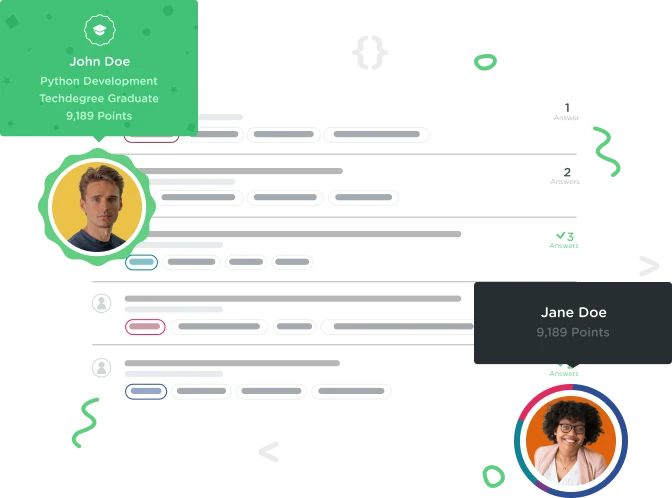

martin cartledge
1,933 PointsAPI call is successfully made but, getting an error in the console
I am attempting to make an API call using the Netflix Roulette API and I've console logged the response to ensure I am retrieving info (which I am) but, I am still getting this error in the console:
Failed to load resource: net::ERR_INCOMPLETE_CHUNKED_ENCODING
I have been told this could be cause from a few things:
⢠Anti-virus software
⢠Google chrome browser settings
⢠Ensuring all responses have a response.end()
I have eliminated these cause and I am still getting this error. Below is where I make the call:
var EventEmitter = require("events").EventEmitter;
var https = require("https");
var http = require("http");
var util = require("util");
var unirest = require('unirest');
/**
* An EventEmitter to get actor's Netflix info
* @param actor
* @constructor
*/
function Actor(actor) {
EventEmitter.call(this);
actorEmitter = this;
var request = unirest.get("https://community-netflix-roulette.p.mashape.com/api.php?actor=" + actor)
.header("X-Mashape-Key", "DHmuGLtqcomshyLbBKe5akHeFbN1p1UqyGmjsn7uCNpoDXhBXo")
.header("Accept", "application/json")
.end(function(response) {
var body = "";
if (response.statusCode !== 200) {
request.abort();
actorEmitter.emit("error", new Error("There was an error getting titles for " + actor.replace("%20", " ") + ". (" + http.STATUS_CODES[response.statusCode] + ")"));
}
response.on('data', function(chunk) {
body += chunk;
actorEmitter.emit("data", chunk);
});
response.on("end", function() {
if (response.statusCode === 200) {
try {
var actor = JSON.parse(body);
actorEmitter.emit("end", actor);
} catch (error) {
actorEmitter.emit("error", error);
}
}
}).on("error", function(error) {
actorEmitter.emit("error", error);
});
});
}
util.inherits(Actor, EventEmitter);
module.exports = Actor;
If you need more reference, here's a link to the repo:
2 Answers
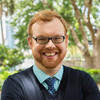
Andrew Chalkley
Treehouse Guest TeacherCheck out this video - https://teamtreehouse.com/library/getting-the-response-body I use the toString()
method.
But you may have to use a new Buffer();
for the body
and on line body += chunk;
do replace it with something like body.concat(chunk)
: https://nodejs.org/api/buffer.html#buffer_class_method_buffer_concat_list_totallength
Then body.toString()
when you parse the JSON.

martin cartledge
1,933 PointsI'm kind of at a loss as where to go from here. I've tried multiple variations of implementing a new Buffer but, I must be missing something. Can someone please help?? It has to be something small I am glancing over.
Here are a few things I've tried:
response.on('data', function(data) {
var concatBody = Buffer.concat(body, data.toString());
actorEmitter.emit("data", data);
});
console.log(typeof concatBody);
response.on("end", function() {
if (response.statusCode === 200) {
try {
var actor = JSON.parse(concatBody);
actorEmitter.emit("end", actor);
} catch (error) {
actorEmitter.emit("error", error);
}
}
response.on('data', function(data) {
body.concat(data.toString());
actorEmitter.emit("data", data);
});
console.log(typeof body);
response.on("end", function() {
if (response.statusCode === 200) {
try {
var actor = JSON.parse(body);
actorEmitter.emit("end", actor);
} catch (error) {
actorEmitter.emit("error", error);
}
}
martin cartledge
1,933 Pointsmartin cartledge
1,933 PointsHey thanks Andrew Chalkley, that all makes sense but, I still must be missing something.
Here's my code:
I've also tried these: