Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial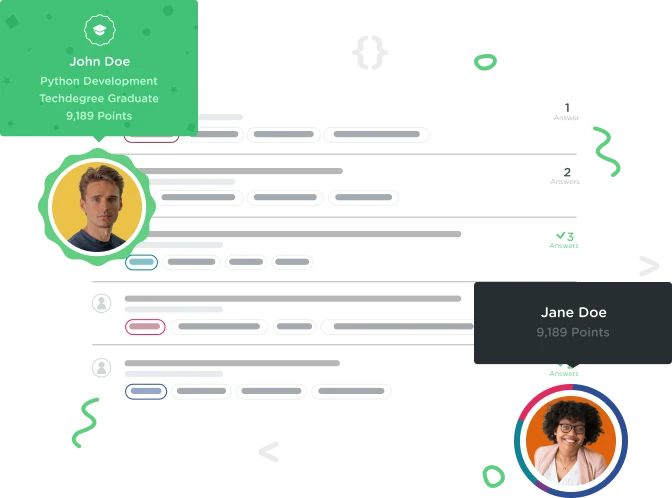

Chase Maddox
Courses Plus Student 5,461 PointsApostrophe in Given Setup Causing Error or Bad Code?
Hi,
In the given setup for this Code Challenge there is an apostrophe in the word "I'm" of the given move() function that causes the rest of the code to be commented out. I deleted that apostrophe, but I'm not sure if that's what's causing my code to not be accepted as correct or how to keep it in and not have everything be commented out.
When I try my code in a separate Xcode playground it seems to work fine, and I'm able to create instances of Robot and use the override move() function to then check my location has moved.
Thanks, Chase
class Point {
var x: Int
var y: Int
init(x: Int, y: Int) {
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(_ direction: String) {
print("Do nothing! Im a machine!")
}
}
// Enter your code below
class Robot: Machine {
init(x: Int, y: Int) {
super.init()
}
override func move(_ direction: String) {
switch direction {
case "Up": location.y += 1
case "Down": location.y -= 1
case "Right": location.x += 1
case "Left" : location.x -= 1
default: break
}
}
}
1 Answer
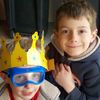
Chris Stromberg
Courses Plus Student 13,389 PointsRemove the following code. The challenge did not ask for you to provide a designated initializer for the Robot class.
init(x: Int, y: Int) {
super.init()
}
I don't think your code will work as you intend it to. The following will take in parameters you provide, however it will set the values of both x and y to zero. This is because you are calling super.init() which is calling the initializer in the base class.
init(x: Int, y: Int) {
super.init()
}
If you wanted to provide values for x and y, you would need to do something like this. I named the internal parameter names robotX and robotY for clarity.
init(robotX : Int, robotY : Int) {
super.init()
//point : Point(x: robotX, y: robotY)
self.location = Point(x: robotX, y: robotY)
}
Chase Maddox
Courses Plus Student 5,461 PointsChase Maddox
Courses Plus Student 5,461 PointsAhhh ok, thanks so much! I think I got so used to calling super.init() that I didn't even realize it didn't ask for it.