Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial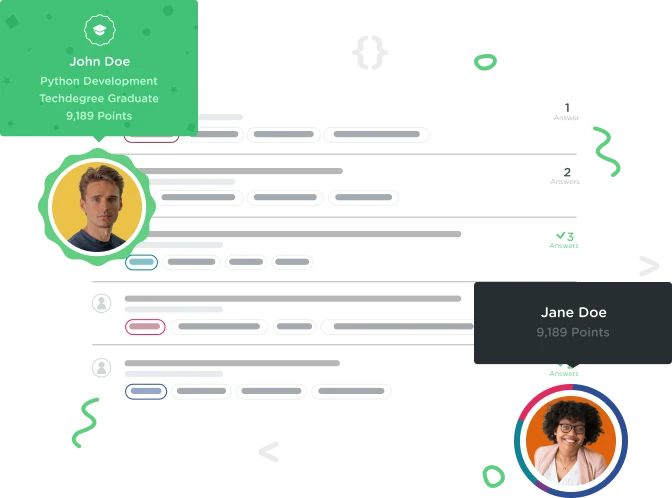
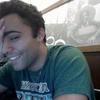
Kevin Lavi
3,311 PointsApp can't connect to the blog after doing "Opening a Webpage in Browser" class.
My app was working fine. I had the title and author showing. For some reason after finishing the "Opening a Webpage in Browser" section I went to try it out and now my app can't connect to the blog (I'm getting the error message we made earlier). I'm having trouble understanding logcat to figure out what I did wrong, especially since we have a few different exceptions. Here is my code if someone can help:
public class MainListActivity extends ListActivity {
public static final int NUMBER_OF_POSTS = 20;
public static final String TAG = MainListActivity.class.getSimpleName();
protected JSONObject mBlogData;
protected ProgressBar mProgressBar;
private final String KEY_TITLE = "title";
private final String KEY_AUTHOR = "author";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main_list);
mProgressBar = (ProgressBar) findViewById(R.id.progressBar1);
if (isNetworkAvailable()) {
mProgressBar.setVisibility(View.VISIBLE);
GetBlogPostsTask getBlogPostsTask = new GetBlogPostsTask();
getBlogPostsTask.execute();
}
else {
Toast.makeText(this, "Network is unavailable!", Toast.LENGTH_LONG).show();
}
//String message = getString(R.string.no_items);
//Toast.makeText(this, message, Toast.LENGTH_LONG).show();
}
@Override
protected void onListItemClick(ListView l, View v, int position, long id) {
super.onListItemClick(l, v, position, id);
try {
JSONArray jsonPosts = mBlogData.getJSONArray("posts");
JSONObject jsonPost = jsonPosts.getJSONObject(position);
String blogUrl = jsonPost.getString("url");
Intent intent = new Intent(Intent.ACTION_VIEW);
intent.setData(Uri.parse(blogUrl));
startActivity(intent);
} catch (JSONException e) {
logException(e);
}
}
private void logException(Exception e) {
Log.e(TAG, "exception caught!", e);
}
private boolean isNetworkAvailable() {
ConnectivityManager manager = (ConnectivityManager)
getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo networkinfo = manager.getActiveNetworkInfo();
boolean isAvailable = false;
if(networkinfo != null && networkinfo.isConnected()) {
isAvailable = true;
}
return isAvailable;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
public void handleBlogResponse() {
mProgressBar.setVisibility(View.INVISIBLE);
if (mBlogData == null) {
updateDisplayForError();
}
else {
try {
JSONArray jsonPosts = mBlogData.getJSONArray("posts");
ArrayList<HashMap<String, String>> blogPosts =
new ArrayList<HashMap<String, String>>();
for (int i = 0; i < jsonPosts.length(); i++) {
JSONObject post = jsonPosts.getJSONObject(i);
String title = post.getString(KEY_TITLE);
title = Html.fromHtml(title).toString();
String author = post.getString(KEY_AUTHOR);
author = Html.fromHtml(author).toString();
HashMap<String, String> blogPost = new HashMap<String, String>();
blogPost.put(KEY_TITLE, title);
blogPost.put(KEY_AUTHOR, author);
blogPosts.add(blogPost);
}
String[] keys = {KEY_TITLE, KEY_AUTHOR};
int[] ids = {android.R.id.text1, android.R.id.text2 };
SimpleAdapter adapter =
new SimpleAdapter(this, blogPosts, android.R.layout.simple_list_item_2, keys, ids);
setListAdapter(adapter);
}
catch (JSONException e) {
Log.e(TAG, "Exception caught!", e);
}
}
}
private void updateDisplayForError() {
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setTitle(getString(R.string.title));
builder.setMessage(getString(R.string.Error_message));
builder.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
TextView emptyTextView = (TextView) getListView().getEmptyView();
emptyTextView.setText(getString(R.string.no_items));
//TODO: Handle error
}
private class GetBlogPostsTask extends AsyncTask<Object, Void, JSONObject> {
@Override
protected JSONObject doInBackground(Object... params) {
int responseCode = -1;
JSONObject jsonResponse = null;
try {
URL blogFeedUrl = new URL("http://blog.teamtreehouse.com/api/get_recent_summary/?count=" + NUMBER_OF_POSTS);
HttpURLConnection connection = (HttpURLConnection) blogFeedUrl.openConnection();
connection.connect();
responseCode = connection.getResponseCode();
if(responseCode == HttpURLConnection.HTTP_OK){
InputStream inputstream = connection.getInputStream();
Reader reader = new InputStreamReader(inputstream);
int contentLength = connection.getContentLength();
char[] charArray = new char[contentLength];
reader.read(charArray);
String responseData = new String(charArray);
jsonResponse = new JSONObject(responseData);
}
else {
Log.i(TAG, "Unsuccessful HTTP Response Code: " + responseCode);
}
}
catch(MalformedURLException e) {
logException(e);
}
catch (IOException e) {
logException(e);
}
catch (Exception e) {
logException(e);
}
return jsonResponse;
}
@Override
protected void onPostExecute(JSONObject result) {
mBlogData = result;
handleBlogResponse();
}
}
}
2 Answers

Ben Jakuben
Treehouse TeacherGlad you got it working, but sorry we don't have a good explanation! I have a hunch though.
There is a known issue with this section of code that's listed in the Teacher's Notes of this video page. (I will be refreshing this course soon, too.) If you change the number of posts from 20 to 50, you might be able to reproduce this bug. There was a bad post in the past month that was causing problems, but once it gets beyond one of the 20 most recent, it clears itself up. Let me know if you are able to recreate it by requesting more entries.

Ben Jakuben
Treehouse TeacherCan you paste in your logcat errors? That will help us troubleshoot as well.
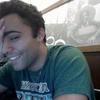
Kevin Lavi
3,311 PointsI ran the emulator again so that i can get the logcat errors to paste here and sure enough it works just fine now... This isn't the first time something like this has happened to me, is it possible to run a program a wrong way?