Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial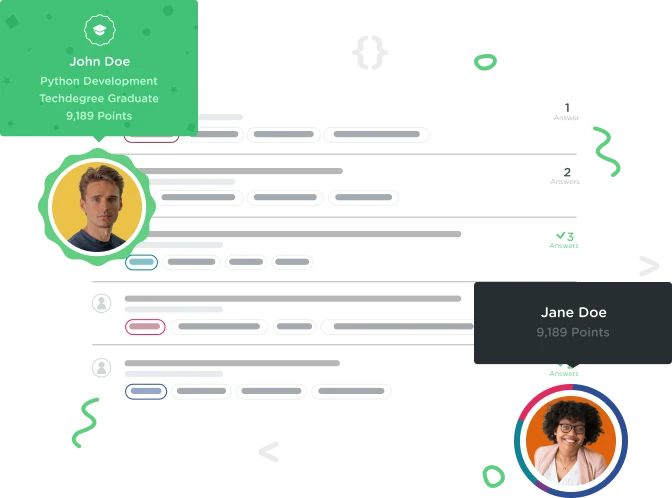

Javier Pacheco
382 PointsApp closes inmediately
Hi, I'm getting an error each time I try to run my app, it says something like "You need to use a Theme .AppCompat theme (or descendant) with this directory"
13 Answers

Tom Williams
7,473 PointsAndroidManifext.xml
```<manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.crystalball" android:versionCode="1" android:versionName="1.0" >
<uses-sdk android:minSdkVersion="8" android:targetSdkVersion="18" />
<application android:allowBackup="true" android:icon="@drawable/ic_launcher" android:label="@string/app_name" android:theme="@android:style/Theme.Black.NoTitleBar.Fullscreen" > <activity android:name="com.example.crystalball.MainActivity" android:label="@string/app_name" android:screenOrientation="portrait"> <intent-filter> <action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application> </manifest>```
MainActivity.java
```package com.example.crystalball;
import android.app.Activity; import android.graphics.Color; import android.os.Bundle; import android.support.v7.app.ActionBarActivity; import android.view.Menu; import android.view.MenuItem; import android.view.View; import android.widget.Button; import android.widget.RelativeLayout; import android.widget.TextView;
public class MainActivity extends Activity {
private CrystalBall mCrystalBall = new CrystalBall();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//Declare our view variables
final TextView answerLabel = (TextView) findViewById(R.id.textView1);
Button getAnswerButton = (Button) findViewById(R.id.button1);
//final RelativeLayout mRelativeLayout = (RelativeLayout) findViewById(R.id.layout1);
getAnswerButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
//mRelativeLayout.setBackgroundColor(Color.GREEN);
String answer = mCrystalBall.getAnAnswer();
//Update our label with our dynamic answer
answerLabel.setText(answer);
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
}```
activity_main.xml
```<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" >
<ImageView
android:id="@+id/imageView1"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:scaleType="fitCenter"
android:src="@drawable/ball01" />
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_centerVertical="true"
android:gravity="center_horizontal"
android:shadowColor="@android:color/white"
android:shadowRadius="10"
android:textColor="@android:color/white"
android:textSize="32sp" />
<Button
android:id="@+id/button1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/imageView1"
android:layout_alignParentBottom="true"
android:text="Dale"
android:textColor="#3f0f7f"
android:textSize="24sp"
android:textStyle="bold|italic"
android:typeface="serif" />
</RelativeLayout>```
I know what is happening now sorry about that these file contents work. MainActivity should extend Activity also verify that the package names in each file match your project package example: com.example.crystalball
[Dale Crystal Ball Screen Shot](C:/Users/infiniti technician/Pictures/DaleCrystalBall.png)

Tom Williams
7,473 PointsJavier, is this actually in reference to the Customizing Buttons and Labels video or the video before it on changing the app layout? Android specifically throws this error when the theme has been deprecated for example the, Theme.Black.NoTitleBar.Fullscreen theme has been deprecated and will now throw this error even though the option is still in ADT for selection. The second reason Android will throw this error is if the theme is not compatible with either your physical or emulated testing devices. Further, if you are speaking of the video for changing app layout the fix is under the video in Ben's teacher's notes, but if you would like I could go over the code with you

Javier Pacheco
382 PointsThis is regarding the button customization video, I'm sure because my code from the previous video actually worked like a charm. I can send you my code if you want. BTW, I'm currently using my Samsung Galaxy Nexus for the testing.

Tom Williams
7,473 PointsYeah can you send me the code either copy and paste it here or zip the file and email it to me

Javier Pacheco
382 Pointswhich of the files do you want me to send you or paste here?

Tom Williams
7,473 Pointsactivity_main.xml, the manifest file, and the MainActivity.java

Javier Pacheco
382 Pointsactivity_main.xml:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/layout1" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context="com.example.crystalball.MainActivity$PlaceholderFragment" >
<ImageView
android:id="@+id/imageView1"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:scaleType="fitCenter"
android:src="@drawable/ball01" />
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_centerVertical="true"
android:gravity="center_horizontal"
android:shadowColor="@android:color/white"
android:shadowRadius="10"
android:textColor="@android:color/white"
android:textSize="32sp" />
<Button
android:id="@+id/button1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/imageView1"
android:layout_alignParentBottom="true"
android:text="Dale"
android:textColor="#3f0f7f"
android:textSize="24sp"
android:textStyle="bold|italic"
android:typeface="serif" />
</RelativeLayout>

Javier Pacheco
382 PointsMainActivity.java:
package com.example.crystalball;
import android.graphics.Color; import android.os.Bundle; import android.support.v7.app.ActionBarActivity; import android.view.Menu; import android.view.MenuItem; import android.view.View; import android.widget.Button; import android.widget.RelativeLayout; import android.widget.TextView;
public class MainActivity extends ActionBarActivity {
private CrystalBall mCrystalBall = new CrystalBall();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//Declare our view variables
final TextView answerLabel = (TextView) findViewById(R.id.textView1);
Button getAnswerButton = (Button) findViewById(R.id.button1);
final RelativeLayout mRelativeLayout = (RelativeLayout) findViewById(R.id.layout1);
getAnswerButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
//mRelativeLayout.setBackgroundColor(Color.GREEN);
String answer = mCrystalBall.getAnAnswer();
//Update our label with our dynamic answer
answerLabel.setText(answer);
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
}

Javier Pacheco
382 PointsManifest:
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.crystalball" android:versionCode="1" android:versionName="1.0" >
<uses-sdk
android:minSdkVersion="8"
android:targetSdkVersion="18" />
<application
android:allowBackup="true"
android:icon="@drawable/ic_launcher"
android:label="@string/app_name"
android:theme="@android:style/Theme.Black.NoTitleBar.Fullscreen" >
<activity
android:name="com.example.crystalball.MainActivity"
android:label="@string/app_name" android:screenOrientation="portrait">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>

Tom Williams
7,473 PointsDo you have layout attributes specified for the relative layout tag in activity_main.xml?

Javier Pacheco
382 PointsNo, the only attributes I have specified are the ones for ImageView, TextView and Button elements

Tom Williams
7,473 Points<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<ImageView
android:id="@+id/imageView1"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:scaleType="fitCenter"
android:src="@drawable/ball01" />
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_centerVertical="true"
android:gravity="center_horizontal"
android:shadowColor="@android:color/white"
android:shadowRadius="10"
android:textColor="@android:color/white"
android:textSize="32sp" />
<Button
android:id="@+id/button1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/imageView1"
android:layout_alignParentBottom="true"
android:text="Dale"
android:textColor="#3f0f7f"
android:textSize="24sp"
android:textStyle="bold|italic"
android:typeface="serif" />
</RelativeLayout>
Try copying all of that into your activity_main.xml file and that should resolve the issue that you are having. Notice in the opening RelativeLayout tag the attributes which designate the template and the size of the relative view.

Javier Pacheco
382 PointsI'm still getting the same error. Here is my code:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/layout1"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.example.crystalball.MainActivity$PlaceholderFragment" >
<ImageView
android:id="@+id/imageView1"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:scaleType="fitCenter"
android:src="@drawable/ball01" />
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_centerVertical="true"
android:gravity="center_horizontal"
android:shadowColor="@android:color/white"
android:shadowRadius="10"
android:textColor="@android:color/white"
android:textSize="32sp" />
<Button
android:id="@+id/button1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/imageView1"
android:layout_alignParentBottom="true"
android:text="Dale"
android:textColor="#3f0f7f"
android:textSize="24sp"
android:textStyle="bold|italic"
android:typeface="serif" />
</RelativeLayout>

Tom Williams
7,473 PointsJavier are you closing the RelativeLayout tag at the end of the code? also did you try running the code exactly as I sent over to you and then tried changing the attributes after that I only ask because there are more attributes including an id attribute that what I sent over. Just trying to figure it out because after I edited that file the application launched without crashing

Javier Pacheco
382 PointsYes, I just tried with your code and the app still crashes

Javier Pacheco
382 PointsCould it be something related to the theme?

Javier Pacheco
382 PointsThank you very much! It is working now!

Tom Williams
7,473 PointsNo problem that is why we are all here
Tom Williams
7,473 PointsTom Williams
7,473 Pointsthree things is like I said above you need to extend activity because there is no ActionbarActivity to extend. Notice that I commented out the final RelativeLayout. Lastly in the manifest I removed the xml version tag because android doesn't really like that also I apologize in advance because I am using the syntax from the markdown cheat sheet but it is formatting the code weird if you notice it cut out a large amount of the manifest if you would like you can find me on skype at williamscodes@yahoo.com