Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial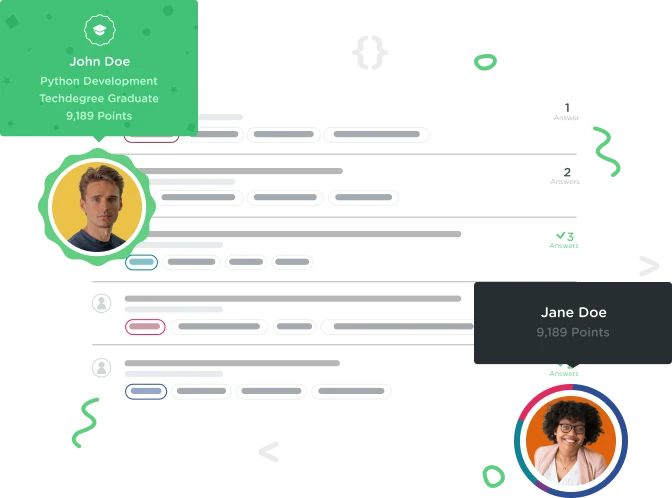

Sum Tsui
Full Stack JavaScript Techdegree Student 29,117 PointsApp crashed clicking Hourly button. Required view 'recyclerView' with ID 2131492971 for field 'mRecyclerView' not found.
when i clicked the Hourly button the app crashed. the logcat said: Caused by: java.lang.IllegalStateException: Required view 'recyclerView' with ID 2131492971 for field 'mRecyclerView' was not found. If this view is optional add '@Nullable' annotation.
I checked my code line by line with the code downloaded from Treehouse but still counldn't find out where went wrong :(
HourAdapter.java
package net.sumtsui.stormy.adapters;
import android.content.Context;
import android.support.v7.widget.RecyclerView;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ImageView;
import android.widget.TextView;
import android.widget.Toast;
import net.sumtsui.stormy.R;
import net.sumtsui.stormy.weather.Hour;
public class HourAdapter extends RecyclerView.Adapter<HourAdapter.HourViewHolder> {
private Hour[] mHours;
private Context mContext;
public HourAdapter(Context context, Hour[] hours) {
mContext = context;
mHours = hours;
}
@Override
public HourViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View view = LayoutInflater.from(parent.getContext())
.inflate(R.layout.hourly_list_item, parent, false);
HourViewHolder viewHolder = new HourViewHolder(view);
return viewHolder;
}
@Override
public void onBindViewHolder(HourViewHolder holder, int position) {
holder.bindHour(mHours[position]);
}
@Override
public int getItemCount() {
return mHours.length;
}
public class HourViewHolder extends RecyclerView.ViewHolder
implements View.OnClickListener {
public TextView mTimeLabel;
public TextView mSummaryLabel;
public TextView mTemperatureLabel;
public ImageView mIconImageView;
public HourViewHolder(View itemView) {
super(itemView);
mTimeLabel = (TextView) itemView.findViewById(R.id.timeLabel);
mSummaryLabel = (TextView) itemView.findViewById(R.id.summaryLabel);
mTemperatureLabel = (TextView) itemView.findViewById(R.id.temperatureLabel);
mIconImageView = (ImageView) itemView.findViewById(R.id.iconImageView);
itemView.setOnClickListener(this);
}
public void bindHour(Hour hour) {
mTimeLabel.setText(hour.getHour());
mSummaryLabel.setText(hour.getSummary());
mTemperatureLabel.setText(hour.getTemperature() + "");
mIconImageView.setImageResource(hour.getIconId());
}
@Override
public void onClick(View v) {
String time = mTimeLabel.getText().toString();
String temperature = mTemperatureLabel.getText().toString();
String summary = mSummaryLabel.getText().toString();
String message = String.format("At %s it will be %s and %s",
time,
temperature,
summary);
Toast.makeText(mContext, message, Toast.LENGTH_LONG).show();
}
}
}
HourlyForecastActivity
package net.sumtsui.stormy.ui;
import android.content.Intent;
import android.os.Bundle;
import android.os.Parcelable;
import android.support.v7.widget.LinearLayoutManager;
import android.support.v7.widget.RecyclerView;
import java.util.Arrays;
import butterknife.ButterKnife;
import butterknife.Bind;
import net.sumtsui.stormy.R;
import net.sumtsui.stormy.adapters.HourAdapter;
import net.sumtsui.stormy.weather.Hour;
public class HourlyForecastActivity extends AppCompatActivity {
private Hour[] mHours;
@Bind(R.id.recyclerView) RecyclerView mRecyclerView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_hourly_forecast);
ButterKnife.bind(this);
Intent intent = getIntent();
Parcelable[] parcelables = intent.getParcelableArrayExtra(MainActivity.HOURLY_FORECAST);
mHours = Arrays.copyOf(parcelables, parcelables.length, Hour[].class);
HourAdapter adapter = new HourAdapter(this, mHours);
mRecyclerView.setAdapter(adapter);
RecyclerView.LayoutManager layoutManager = new LinearLayoutManager(this);
mRecyclerView.setLayoutManager(layoutManager);
mRecyclerView.setHasFixedSize(true);
}
}
hourly_list_item.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="wrap_content"
tools:background="@drawable/bg_gradient"
android:paddingTop="8dp"
android:paddingBottom="8dp">
<TextView
android:layout_width="80dp"
android:layout_height="wrap_content"
android:id="@+id/timeLabel"
android:layout_centerVertical="true"
android:layout_alignParentStart="true"
android:textColor="#ffffffff"
android:textSize="24sp"
tools:text="12 PM"/>
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/iconImageView"
android:layout_alignParentTop="true"
android:layout_toEndOf="@+id/timeLabel"
android:layout_toRightOf="@+id/timeLabel"
android:layout_centerVertical="true"
android:src="@drawable/partly_cloudy" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
tools:text="100"
android:id="@+id/temperatureLabel"
android:layout_alignBottom="@+id/timeLabel"
android:layout_alignParentEnd="true"
android:layout_centerVertical="true"
android:textColor="#ffffffff"
android:textSize="24sp"/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/summaryLabel"
android:layout_centerVertical="true"
android:layout_toEndOf="@+id/iconImageView"
android:textColor="#ffffffff"
android:layout_toLeftOf="@+id/temperatureLabel"
android:paddingLeft="15dp"
android:paddingRight="15dp"
tools:text="Partly Cloudy"
android:textSize="16sp"/>
</RelativeLayout>
content_hourly_forecast.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:paddingBottom="@dimen/activity_vertical_margin"
app:layout_behavior="@string/appbar_scrolling_view_behavior"
tools:context="net.sumtsui.stormy.ui.HourlyForecastActivity"
android:background="@drawable/bg_gradient">
<android.support.v7.widget.RecyclerView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@+id/recyclerView" />
</RelativeLayout>
Hour.java
package net.sumtsui.stormy.weather;
import android.os.Parcel;
import android.os.Parcelable;
import java.text.SimpleDateFormat;
import java.util.Date;
public class Hour implements Parcelable {
private long mTime;
private String mSummary;
private double mTemperature;
private String mIcon;
private String mTimezone;
public long getTime() {
return mTime;
}
public void setTime(long time) {
mTime = time;
}
public String getSummary() {
return mSummary;
}
public void setSummary(String summary) {
mSummary = summary;
}
public int getTemperature() {
return (int)Math.round(mTemperature);
}
public void setTemperature(double temperature) {
mTemperature = temperature;
}
public String getIcon() {
return mIcon;
}
public int getIconId() {
return Forecast.getIconId(mIcon);
}
public void setIcon(String icon) {
mIcon = icon;
}
public String getTimezone() {
return mTimezone;
}
public void setTimezone(String timezone) {
mTimezone = timezone;
}
public String getHour() {
SimpleDateFormat formatter = new SimpleDateFormat("h a");
Date date = new Date(mTime * 1000);
return formatter.format(date);
}
public Hour() {}
@Override
public int describeContents() {
return 0; // ignore
}
@Override
public void writeToParcel(Parcel dest, int flags) {
dest.writeLong(mTime);
dest.writeString(mSummary);
dest.writeDouble(mTemperature);
dest.writeString(mIcon);
dest.writeString(mTimezone);
}
private Hour(Parcel in) {
mTime = in.readLong();
mSummary = in.readString();
mTemperature = in.readDouble();
mIcon = in.readString();
mTimezone = in.readString();
}
public static final Creator<Hour> CREATOR = new Creator<Hour>() {
@Override
public Hour createFromParcel(Parcel source) {
return new Hour(source);
}
@Override
public Hour[] newArray(int size) {
return new Hour[size];
}
};
}
1 Answer
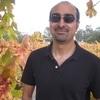
Kourosh Raeen
23,733 PointsDo you have the file activity_hourly_forecast.xml? And does it have the following include tag?
<include layout="@layout/content_hourly_forecast"/>
Sum Tsui
Full Stack JavaScript Techdegree Student 29,117 PointsSum Tsui
Full Stack JavaScript Techdegree Student 29,117 PointsNo and I added the line and it finally work! thank you. That line should be there automatically when the layout created right? i must deleted it by accident.